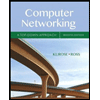
Please add to the Game code below (TODO LISTED):
/*TODO add no argument constructor constructor*/
/*TODO add three argument constructor*/
/*TODO create an attack method that returns a random value from 1 to weapon's strength and deducts 1 from durability*/
/*TODO in the attack method if durability is 0 return 0*/
/*TODO create a toString override*/
import java.util.Random;
//Game driver
//Notice it's the only class declared as public.
public class GameBattleDriver
{
public static void main(String [] args)
{
Character hero = new Character("Sparhawk", 40, 10);
//System.out.println(Character.numChars);
Character badguy = new Character("Gwerg", 100, 3);
//System.out.println(Character.numChars);
Character hero2 = new Character("Sparhawk", 40, 10);
//System.out.println(Character.numChars);
//BattleArena b1 = new BattleArena(hero, badguy);
//b1.fight();
BattleArena.fight(hero, badguy);
//System.out.println(hero.attack());
//System.out.println(hero.attack(45));
//System.out.println(hero);
//System.out.println(hero.toString());
/*TODO Create a weapon and "give it" to the hero to use*/
/*TODO Add some print statements to print the weapon that was given to the hero*/
}
}
/**
* Character class
* Generic RPG Character
*/
class Character
{
private String name;
private int hitPoints;
private int strength;
private boolean alive;
private Weapon w1;
public static int numChars = 0;
/**
* Construct a character object initializing all variables other than alive and set alive to be true
* @param name - characters name
* @param hitPoints - initial set of hitPoints for our character
* @param strength - initial strength of our character.
*/
public Character(String name, int hitPoints, int strength)
{
numChars++;
alive = true;
this.name=name;
this.hitPoints=hitPoints;
this.strength=strength;
}
public void setWeapon(Weapon w1)
{
this.w1 = w1;
}
//Example of method override
//Over riding the toString method in Object
@Override
public String toString()
{
return "name : " + name + "\nHit Poist : " + hitPoints + "\nStrenght :" + strength;
}
//These two attack methods are an example of method overloading....
public int attack()
{
Random r1 = new Random();
/*TODO update this function to add the weapon attack*/
/*TODO make sure weapon doesn't equal null before adding it*/
return r1.nextInt(strength);
//return r1.nextInt(strength) + w1.attack();
}
public int attack(int strength)
{
Random r1 = new Random();
return r1.nextInt(strength);
}
public void takeDamage(int damage)
{
setHitPoints(hitPoints - damage);
}
/**
* Basic setter for hitPoints
* Assigns new value to character hitPoints
* @param hitPoints
*/
public void setHitPoints(int hitPoints)
{
if (hitPoints <=0)
{
this.hitPoints = 0;
alive = false;
}
else
{
this.hitPoints=hitPoints;
}
}
//The minimum getters needed for this to work skipping documentation for this.
public String getName(){return name;}
public int getHitPoints(){return hitPoints;}
public int getStrength(){return strength;}
/**
* Return the value of the alive status variable
* Allows outside objects to determine if current character is alive
* @return value of alive status variable
*/
public boolean isAlive(){return alive;}
/*TODO Add a toString overload for character and test*/
}
/**
Battle Arena class object
Facilitates an epic battle between two character class objects
*/
class BattleArena
{
private Character c1;
private Character c2;
public BattleArena(Character c1, Character c2)
{
this.c1=c1;
this.c2=c2;
/*TODO Make the call to this manual instead of having it run by the constructor*/
//fight();
}
/**
* Epic battle between two characters
* Method internal to class object
*/
public static void fight(Character c1, Character c2)
{
int damage = 0;
//Random r1 = new Random();
//Main game loop
while(c1.isAlive() && c2.isAlive())
{
//damage = r1.nextInt(c1.getStrength());
damage = c1.attack();
System.out.println(c1.getName() + " hits " + c2.getName() + " for " + damage);
//c2.setHitPoints(c2.getHitPoints()-damage);
c2.takeDamage(damage);
//Pause for 2 seconds to add some suspense
//This is an example of a checked exception. It won't work without it...
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
//Don't do damage if 2nd combatant was killed
if (c2.isAlive())
{
//damage = r1.nextInt(c2.getStrength());
damage = c2.attack();
System.out.println(c2.getName() + " hits " + c1.getName() + " for " + damage);
//c1.setHitPoints(c1.getHitPoints() - damage);
c1.takeDamage(damage);
}
}
System.out.print("Our winner is : " );
//Report the winner
if (c1.isAlive())
System.out.println(c1.getName());
else
if (c2.isAlive())
System.out.println(c2.getName());
}
}
class Weapon
{
private String name;
private int strength;
private int durability;
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Charge Account ValidationCreate a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:5658845 4520125 7895122 8777541 8451277 13028508080152 4562555 5552012 5050552 7825877 12502551005231 6545231 3852085 7576651 7881200 4581002These numbers should be stored in an array. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.Write a program that tests the class by asking the user to enter a charge account number. The program should display a message indicating whether the number is valid or invalid.arrow_forwardA method that returns the value of an object's instance variable is calleda(n)a) mutator b) function c) constructor d) accessorarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
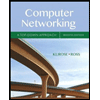
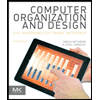
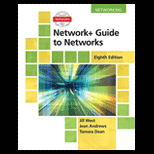
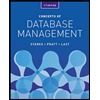
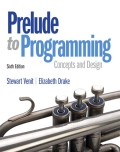
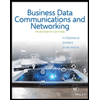