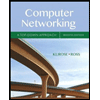
Mix and incorporate these code with binary search engine
BST
If root == NULL
return NULL;
If number == root->data
return root->data;
If number < root->data
return search(root->left)
If number > root->data
return search(root->right)
#include <stdio.h>
//Represent a node of the singly linked list
struct node{
int data;
struct node *next;
};
//Represent the head and tail of the singly linked list
struct node *head, *tail = NULL;
//addNode() will add a new node to the list
void addNode(int data) {
//Create a new node
struct node *newNode = (struct node*)malloc(sizeof(struct node));
newNode->data = data;
newNode->next = NULL;
//Checks if the list is empty
if(head == NULL) {
//If list is empty, both head and tail will point to new node
head = newNode;
tail = newNode;
}
else {
//newNode will be added after tail such that tail's next will point to newNode
tail->next = newNode;
//newNode will become new tail of the list
tail = newNode;
}
}
//removeDuplicate() will remove duplicate nodes from the list
void removeDuplicate() {
//Node current will point to head
struct node *current = head, *index = NULL, *temp = NULL;
if(head == NULL) {
return;
}
else {
while(current != NULL){
//Node temp will point to previous node to index.
temp = current;
//Index will point to node next to current
index = current->next;
while(index != NULL) {
//If current node's data is equal to index node's data
if(current->data == index->data) {
//Here, index node is pointing to the node which is duplicate of current node
//Skips the duplicate node by pointing to next node
temp->next = index->next;
}
else {
//Temp will point to previous node of index.
temp = index;
}
index = index->next;
}
current = current->next;
}
}
}
//display() will display all the nodes present in the list
void display() {
//Node current will point to head
struct node *current = head;
if(head == NULL) {
printf("List is empty \n");
return;
}
while(current != NULL) {
//Prints each node by incrementing pointer
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main()
{
//Adds data to the list
addNode(1);
addNode(2);
addNode(3);
addNode(2);
addNode(2);
addNode(4);
addNode(1);
printf("Originals list: \n");
display();
//Removes duplicate nodes
removeDuplicate();
printf("List after removing duplicates: \n");
display();
return 0;
}
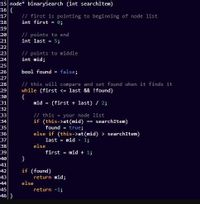

Step by stepSolved in 2 steps with 1 images

- struct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forwardIN C LANGUAGE When inserting a node into a linked list, where (in memory) will that node be placed if there is no overflow? A) The location of the AVAIL pointer B) The next contiguous spot of memory from the last node C) The very end of the contiguous memory block D) A randomized memory locationarrow_forwardplease complate code in fill in the blanksarrow_forward
- In this code: #include <iostream> #include <string> using namespace std;// Node struct to store data for each song in the playliststruct Node { string data; Node * next;};// Function to create a new node and return its addressNode * getNewNode(string song) { Node * newNode = new Node(); newNode -> data = song; newNode -> next = NULL; return newNode;}// Function to insert a new node at the head of the linked listvoid insertAtHead(Node ** head, string song) { Node * newNode = getNewNode(song); if ( * head == NULL) { * head = newNode; return;} newNode -> next = * head; * head = newNode;}// Function to insert a new node at the tail of the linked listvoid insertAtTail(Node ** head, string song) { Node * newNode = getNewNode(song); if ( * head == NULL) { * head = newNode; return; } Node * temp = * head; while (temp -> next != NULL) { temp = temp -> next; } temp -> next = newNode;}// Function to remove a node from the linked listvoid removeNode(Node ** head,…arrow_forwardWhich of the following data structures can we use the [] operator on? Arrays B-Trees Maps Multimapsarrow_forwardTepis Linked List By using stt Usc this declaration of the Node clas3: atzuct Node { ant infoJ Node next Node *p, r, *g: Question 1 nitiol Setup Exercise Final Configuration Use o single cssignment Exomple stotement to make the p=p->next->data, variable p refer to the Node with info 2 Use a single ossignment Code: stotement to cssignment statement must refer to both variables p and q. Use o single Ossignment stotement to make the| Code: variable a refer to the / Node with info T. Use o single ossianment | Code: statement to make the variable r refer to the Node with info 2. Use a single ossignment Code: stotement to set the info referred to by p equal to the info of the Node referred to by ryou must access this info through r; do not refer to the of the Node character '3' directly)arrow_forward
- Stuck on thisarrow_forwardCreate a random sized linked list of randomly generated integers. Insert 25 to 75 random integers in the inclusive range 0 to 100 into the linked list. Display the contents, the sum, the count, and average of the integers. Create a program named LastnameFirstname17.c, that does the following: Define a structure named node that stores a single integer and a pointer to another node. You may use the struct definition in linkedlist.c as a template to get started. Keep in mind that the data in the linkedlist.c example was a string implemented as a char[]. For this assignment, the data is an integer, just a single int. You may want to define a typedef to create alias' for the node structure and node pointer for convenience. Setup your program to generate random numbers. Import the necessary includes and seed the random function. Generate a random integer between 25 and 75, inclusive, this number will be the size of the linked list. The size of the linked list: int size = 25 + rand()…arrow_forwardprogram Linked List: modify the following program to make a node containing data values of int, char, and string. #include <iostream> using namespace std; struct node { int data; struct Node *next; }; struct Node* head = nullptr;//or Null or 0; void insert(int new_data) { struct Node* new_node=(struct Node*) new(struct Node); new_mode->data=new_data; new_mode->next=head; head=new_node; } void display() { struct Node* ptr; ptr=head; while(ptr ! = NULL) { cout<<ptr->data<<""; ptr=ptr->next; } } int main() { insert{2}; display{}; return0; }arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
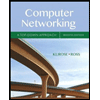
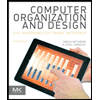
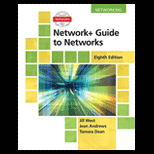
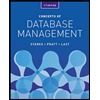
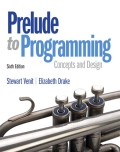
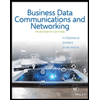