Modify from the code below, add an additional input from the use input to get how many random integers to create. Use a vector instead of an int array to store the random integers. Function prototypes: void showValues(const vector&); void fillVector(vector&, int, int); int maximum(const vector&); int minimum(const vector&); double average(const vector&); bool searchValue(const vector&, int); Code: #include #include #include #include using namespace std; // Function prototype void showValues(const int[], int); void fillArray(int[], int, int, int); int maximum(const int[], int); int minimum(const int[], int); double average(const int[], int); int main() { const int ARRAY_SIZE = 10; int numbers[ARRAY_SIZE]; srand(time(0)); int low = 0, high = 1; cout << "**This program will generate 10 random integers between [low] and [high]**" << endl; // prompt to get the user input for the low and high do { cout << "Please enter an integer value for the value for lower bound: "; cin >> low; cout << "Please enter an integer value for the value for higher bound: "; cin >> high; if (low > high) cout << "The lower bound should be smaller than the higher bound! \n"; } while (low > high); // .... // fill out the random numbers in the array fillArray(numbers, ARRAY_SIZE, low, high); cout << "\nThe 10 random integers between " << low << " and " << high << " are:" << endl; cout << fixed << setprecision(2); showValues(numbers, ARRAY_SIZE); cout << "The greatest value from the random numbers is, " << maximum(numbers, ARRAY_SIZE) << endl; cout << "The samllest value from the random numbers is, " << minimum(numbers, ARRAY_SIZE) << endl; cout << "The average from the random numbers is, " << average(numbers, ARRAY_SIZE) << endl; return 0; } void showValues(const int nums[], int size) { for (int index = 0; index < size; index++) cout << nums[index] << " "; cout << endl; // Use a loop to display all elements in the array } // This function will fill out the nums array with random values between low and high void fillArray(int nums[], int size, int low, int high) { for (int i = 0; i < size; i++) nums[i] = rand() % (high - low) + low; // Use a loop to fill out each element of the array between low to high } int maximum(const int nums[], int size) { int max = nums[0]; for (int i = 1; i < size; i++) if (nums[i] > max) max = nums[i]; // Use a loop to find the max value of the array return max; } int minimum(const int nums[], int size) { int min = nums[0]; for (int i = 1; i < size; i++) if (nums[i] < min) min = nums[i]; // Use a loop to find the min value of the array return min; } double average(const int nums[], int size) { int sum = 0; for (int i = 0; i < size; i++) sum = sum + nums[i]; // Use a loop to get the sum of the array return (double)sum / size; }
Modify from the code below, add an additional input from the use input to get how many random integers to create.
void showValues(const vector<int>&);
void fillVector(vector<int>&, int, int);
int maximum(const vector<int>&);
int minimum(const vector<int>&);
double average(const vector<int>&);
bool searchValue(const vector<int>&, int);
Code:
#include <iostream>
#include <iomanip>
#include <cstdlib>
#include <ctime>
using namespace std;
// Function prototype
void showValues(const int[], int);
void fillArray(int[], int, int, int);
int maximum(const int[], int);
int minimum(const int[], int);
double average(const int[], int);
int main()
{
const int ARRAY_SIZE = 10;
int numbers[ARRAY_SIZE];
srand(time(0));
int low = 0, high = 1;
cout << "**This program will generate 10 random integers between [low] and [high]**" << endl;
// prompt to get the user input for the low and high
do
{
cout << "Please enter an integer value for the value for lower bound: ";
cin >> low;
cout << "Please enter an integer value for the value for higher bound: ";
cin >> high;
if (low > high) cout << "The lower bound should be smaller than the higher bound! \n";
}
while (low > high);
// ....
// fill out the random numbers in the array
fillArray(numbers, ARRAY_SIZE, low, high);
cout << "\nThe 10 random integers between " << low << " and " << high << " are:" << endl;
cout << fixed << setprecision(2);
showValues(numbers, ARRAY_SIZE);
cout << "The greatest value from the random numbers is, " << maximum(numbers, ARRAY_SIZE) << endl;
cout << "The samllest value from the random numbers is, " << minimum(numbers, ARRAY_SIZE) << endl;
cout << "The average from the random numbers is, " << average(numbers, ARRAY_SIZE) << endl;
return 0;
}
void showValues(const int nums[], int size)
{
for (int index = 0; index < size; index++)
cout << nums[index] << " ";
cout << endl;
// Use a loop to display all elements in the array
}
// This function will fill out the nums array with random values between low and high
void fillArray(int nums[], int size, int low, int high)
{
for (int i = 0; i < size; i++)
nums[i] = rand() % (high - low) + low;
// Use a loop to fill out each element of the array between low to high
}
int maximum(const int nums[], int size)
{
int max = nums[0];
for (int i = 1; i < size; i++)
if (nums[i] > max)
max = nums[i];
// Use a loop to find the max value of the array
return max;
}
int minimum(const int nums[], int size)
{
int min = nums[0];
for (int i = 1; i < size; i++)
if (nums[i] < min)
min = nums[i];
// Use a loop to find the min value of the array
return min;
}
double average(const int nums[], int size)
{
int sum = 0;
for (int i = 0; i < size; i++)
sum = sum + nums[i];
// Use a loop to get the sum of the array
return (double)sum / size;
}
![**This program will generate random integers between [low] and [high]**
How many random integers you would like to generate: 25
Please enter an integer value for the lower bound: 240
Please enter an integer value for the higher bound: 280
Please enter an value between 240 and 280 to search: 256
The 25 random integers between 240 and 280 are:
277 279 277 267
272 276 278 259 263 262
274 251 277 274
270 266 269 274 250 261
261 270 272 243 240
The greatest value from the random numbers is, 279
The smallest value from the random numbers is, 240
The average from the random numbers is, 266.48
256 can't be found from the random numbers!
Program ended with exit code: 0](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8e7c4d7d-ede9-4b81-8e82-325ff8e9546c%2F9d26792b-02da-4239-b74c-36c5e6d00d5f%2Fzx0pn9p_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

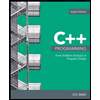
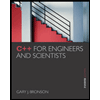
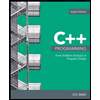
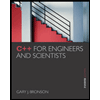