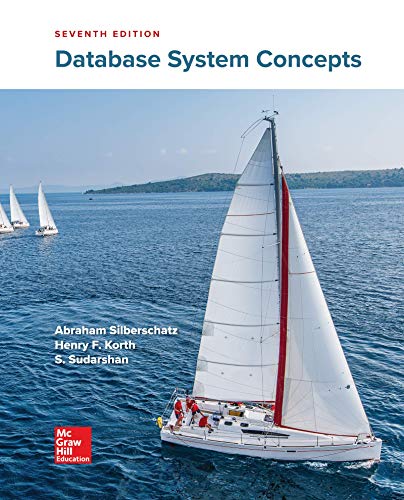
Concept explainers
Randomly generate a partially filled array of unique values.
a) Create a blank partially filled integer array of capacity 10.
b) Implement an array print function.
c) Implement a hasValue function which accepts the array and an integer
value as input. It checks the array for the value and returns true
if the value exists, false if it does not.
d) Implement a randArray function which initializes the array with random
integers between 0 and 9 inclusive. This function should use the
hasValue function to ensure all values are unique (no duplicates).
e) Print the array. Confirm that all values (0-9) are included.
Example 1 Output (input in bold italics)
2 5 8 9 3 4 0 1 7 6
Example 2 Output (input in bold italics)
5 9 3 4 7 0 1 6 2 8

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Description of the assignment: Your program should read in a set of grades (that are all integers) entered by the user. The user will signify the end of the list of grades by entering -999. Do NOT process this value. You can assume that the user will enter at least three grades and not more than 100 grades, all of which will be integers in between 0 and 100, inclusive. For the set of grades, your program should compute the minimum value, print this out to two decimal places and remove it from the data. Then your program should compute the maximum value, print it out to two decimal places and remove it from the data. Then, your program should calculate the mean and standard deviation for the remaining set of grades, and print these out to two decimal places. Finally, a histogram for this data (without the highest and lowest grades) should be printed out as well. Your program should include the following functions: void readGrades (double grades [], int *n); /* This function reads an…arrow_forwardTwo dimension array in C:Create a two dimension array of integers that is 5 rows x 10 columns.Populate each element in the first 2 rows (using for loops) with the value 5.Populate each element of the last three rows (using for loops) with the value 7.Write code (using for loops) to sum all the elements of the first three columns and output thesum to the screen.arrow_forwardPart 2: Unroll Write a function called unroll, which takes in a square array of arrays (i.e. a grid with n rows and n columns). An input could look like this: const square = [ [1, 2, 3, 4], [5, 6, 7, 8], ]; [9, 10, 11, 12], [13, 14, 15, 16] unroll should take in such a square array and return a single array containing the values in the square. You should obtain the values by traversing the square in a spiral: from the top- left corner, move all the way to the right, then all the way down, then all the way to the left, then all the way up, and repeat. For the above example, unroll should return [1, 2, 3, 4, 8, 12, 16, 15, 14, 13, 9, 5, 6, 7, 11, 10].arrow_forward
- C++ 1- Creating an array of 8 random integers /// 2- Copying the elements from the first array to another array that fulfills the following condition: The sum of the element to twice its position is less than the square of the difference between the element that follows it and the element that precedes it except for the first and last element They are copied automatically //// 3- Finds the largest even number in the first array and in what position it is found //// 4- Finds the two smallest odd numbers in the first array and in what position it isarrow_forwardAHPA #10:The Secure Array(use C programming)• A programmer that you work with, Peter, is a jerk.• He is responsible for an array [theArray] that is a key part of an importantprogram and he maintains a sum of the array values at location [0] in the array.• He won't give you access to this array; however, your boss has told you that youneed to get input from the user and then place it into the array.• Each evening Peter will scan the code and remove any illegal references to hisarray.• Using pointers, access Peter's array without him knowing it and place threevalues that you got from the user (101, 63, 21) at locations 3, 6, and 9.Recalculate the sum value and update it. ( the output should be same as the picture)arrow_forward//Required Functionvoid func(int array_1[],int array_2[],int size){ for(int i=0;i<size;i++){ if(array_2[i]>array_1[i]){ array_1[i]=array_2[i]; } } return;} //main function to test the function.int main(){ int size=6; int a[]={1,5,9,8,7,6}; int b[]={1,4,10,12,7,15}; printf("First array before function call: "); for(int i=0;i<size;i++){ printf("%d ",a[i]); } func(a,b,size); //call to the created function printf("\nFirst array after function call: "); for(int i=0;i<size;i++){ printf("%d ",a[i]); } return 0;} 1. Write the statements to do the following: write a prototype for your function in the previous problem write a main function which includes the following steps declare two arrays of ints with 25 elements each prompt the user and read values into both arrays call your function from the previous problem print both arraysarrow_forward
- Two dimension array in C:Create a two dimension array of integers that is 5 rows x 10 columns.Populate each element in the first 2 rows (using for loops) with the value 5.Populate each element of the last three rows (using for loops) with the value 7.Write code (using for loops) to sum all the elements of the first three columns and output thesum to the screen. without using #definearrow_forwardMIPS Assembly The program: Write a function in MIPS assembly that takes an array of integers and finds local minimum points. i.e., points that if the input entry is smaller than both adjacent entries. The output is an array of the same size of the input array. The output point is 1 if the corresponding input entry is a relative minimum, otherwise 0. (You should ignore the output array's boundary items, set to 0.) My code: # (Note: The first/last entry of the output array is always 0# since it's ignored, never be a local minimum.)# $a0: The base address of the input array# $a1: The base address of the output array with local minimum points# $a2: Size of arrayfind_local_minima:############################ Part 2: your code begins here ###la $t1, ($t2)la $t1, ($t2)move $a1, $s0 li $a2, 4jal find_local_minima print:ble $a2, 0, exitlw $a0, ($s0)li $v0, 1syscall addi $s0, $s0, 4addi $a2, $a2, -1 ############################ Part 2: your code ends here ###jr $ra I am not getting the correct…arrow_forwarduse c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forward
- Mirrored Matrices Concepts Tested: iterating through multi-dimensional arrays, functions, function overloading Instructions: 1) Write a SET OF 3 OVERLOADED functions called flipMatrixHorizontal() that takes the following inputs: a) a 2-dimensional array with any number of rows and 3, 4, or 5 columns (this is where the overloading comes in). b) an array to put the flipped array values in c) number of rows d) number of columns The function should reverse the ROWS (rotate around the horizontal axis)of the original array and put the new values in the flipped array. 2) Write a SET OF 3 OVERLOADED functions called flipMatrixVertical() that takes the following inputs: a) a 2-dimensional array with any number of rows and 3, 4, or 5 columns (this is where the overloading comes in). b) an array to put the flipped array values in c) number of rows d) number of columns The function should reverse the COLUMNS (rotate around the vertical axis)of the original…arrow_forwardWrist a code to find the iterative sum of an array and the range (max and min)arrow_forwardAssume that the integer array myScores has been declared and assigned values. Assume that the integer variable size has been declared and initialized using the number of elements in the array myScores. Write a code block that declares a pointer to an integer and initializes it using the address of the array. Use that pointer to access the array elements, compute their average (as double), and display it on the screen.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
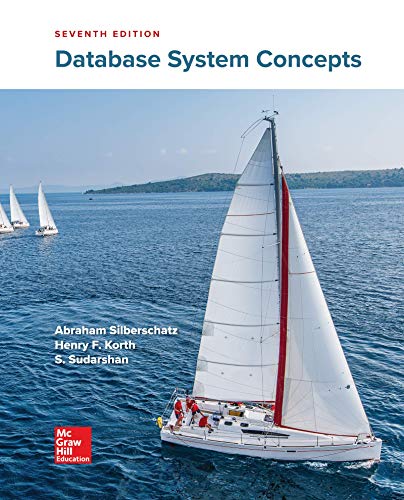
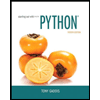
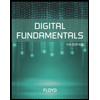
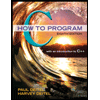
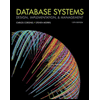
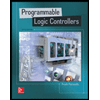