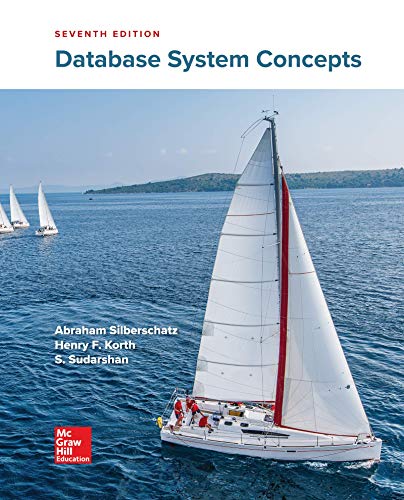
import java.util.Random;
public class TestLength {
public static void main(String[] args) {
Random rand = new Random(10);
Length length[] = new Length[10];
// fill length array and print
for (int i = 0; i < length.length; i++) {
length[i] = new Length(rand.nextInt(11) + 10, rand.nextInt(12));
System.out.print(length[i].toString() + " ");
}
System.out.println();
System.out.println();
// Print sum of the Length objects
Length sum = sum(length);
System.out.println("Sum is: "+sum);
System.out.println();
// use sort method and print
System.out.println("Sorted List");
selectionSort(length);
for (int i = 0; i < length.length; i++) {
System.out.print(length[i].toString() + " ");
}
System.out.println();
}
public static void selectionSort(Length[] arr){
// Your code goes here
}
public static Length sum(Length[] list) {
// Your code goes here
}
}
_____________________________________________________
public class Length
{
private int feet;
private int inches;
// Your code goes here
}
![STAGE 1 | The length of an object can be described by two integers: feet and inches (where 12
inches equal one foot). Class UML is as follows:
- feet: int
- inches : int
+ Length()
+ Length(newFeet: int, newinches: int)
+ getFeet(): int
+ setFeet(newFeet: int) : void
+ getinches(): int
+ setinches(newInches : int) : void
+ add(otherLength: Length): Length
+ subtract(otherLength: Length): Length
+ equals(otherLength: Length): boolean
+ compare To(otherLength: Length): int
+ toString(): String
Length
• Default constructor sets the length of an object to 0 feet & 0 inches
• Overloaded constructor sets the length of an object to given feet & inches (inches must
be in rage)
For each data field, define the accessor and mutator methods
•
add method, adds two length objects and return the result as a length object (feet &
inches must be in range)
• Subtract method, subtracts two length objects and return the result as a length object
(feet & inches must be in range. this object must be larger than otherLength)
• toString() method returns a String in format of [ ## ##"]
For example:
Length meLength = new Length(10, 6);
System.out.println(myLength.toString());
Will display: 10'6"
STAGE 2 | Implement the Length class using the above UML.
STAGE 3 | Compile "Length.java" and fix syntax errors
STAGE 4 | Driver program | Download "TestLength.java' from ZyBooks
1) implement the sum method to calculate sum of all the Length objects.
order
2) Implement the selectionSort to sort all Length objects in ascending or](https://content.bartleby.com/qna-images/question/e4e826cb-c133-47e8-aeaa-858e7b3a09da/c5054a1a-b7b9-4fa5-aa03-d58e23c9b32c/qdupqh_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Javaarrow_forwardI need help with this code !! import java.util.Arrays;import java.util.Scanner; public class MaxElement {public static void main(String[] args) { //create an object for Scanner class Scanner x = new Scanner (System.in);System.out.print ("Enter 10 integers: ");// create an arrayInteger[] arr = new Integer[10]; // Execute for loopfor (int i = 0; i < arr.length; i++) {//get the 10 integersarr[i] = x.nextInt(); } // Print the maximum numberSystem.out.print("The max number is = " + max(arr));System.out.print("\n"); } //max method public static <E extends Comparable<E>> E max(E[] arr) {E max = arr[0]; // Execute for loop for (int i = 1; i < arr.length; i++) { E element = arr[i];if (element.compareTo(max) > 0) {max = element;}}return max; }}arrow_forwardpublic class ArraySection { static void arraySection(int a[], int b[]) { int k = 0; int [] c = new int[a.length]; for(int i = 0; i < a.length; i++) { for(int j = 0; j < b.length; j++) if(a[i] == b[j]) c[k++] = a[i]; } for(int i = 0; i < k; i++) System.out.println(c[i]); System.out.println(); } public static void main(String[] args) { int a[] = { 1, 2, 3, 4, 5 }; int b[] = { 0, 2, 4,5 }; arraySection(a,b); }} Calculate the algorithm step number and algorithm time complexity of the above program?arrow_forward
- Correct my codes in java // Arraysimport java.util.Scanner;public class Assignment1 {public static void main (String args[]){Scanner sc=new Scanner (System.in);System.out.println("Enter mark of student");int n=sc.nextInt();int a[]=new int [n];int i;for(i=0;i<n;i++){System.out.println("Total marks of student in smster");a[i]=sc.nextInt();}int sum=0;for(i=0;i<n;i++){sum=sum+a[i];}System.out.println("Total marks is :");for (i=0;i<n;i++);{System.out.println(a[i]);}System.out.println();}}arrow_forwardimport java.util.ArrayList;import java.util.Scanner;public class CIS231A4JLeh {public static void main(String[] args) {Scanner scnr = new Scanner(System.in);ArrayList<Integer> integers = new ArrayList<>();String input = scnr.nextLine();while (input.isEmpty()) {String[] parts = input.split("\\s+");for (String part : parts) {try {integers.add(Integer.parseInt(part));} catch (NumberFormatException ignored) {}}input = scnr.nextLine();}System.out.println("Jakob");System.out.println("Number of integers input: " + integers.size());System.out.println("All values input, in ascending order:");printIntegers(integers);System.out.println("Lowest value input: " + integers.get(0));System.out.println("Highest value input: " + integers.get(integers.size() - 1));System.out.printf("Average of all values input: %.2f%n", calculateAverage(integers));System.out.println("Mode of the data set and its frequency: " + calculateMode(integers));}private static void printIntegers(ArrayList<Integer>…arrow_forwardNeed some help with this java Problemarrow_forward
- What type of sort is represented by the following code? public class MysterySortExample { public static void mysterySort(int array[]) { array.length; for (int j = 1; j -1) && ( array [i] > key )){ array [i+1] = array [i]; i-; } array[i+1] = key; A insertion bubble selection merge quick F heaparrow_forwardJavaarrow_forwardIn Java please explain how this code prints negative elements as positivearrow_forward
- Javaarrow_forwardWhat type of sort is represented by the following code? public void sort(int arrayToSort[]) { int n = arrayToSort.length; for (int num =n/2; num > 0; num /= 2) { for (int i = num;i= num && arrayToSort[j - num] > key) { arrayToSort[j] = arrayToSort[j - num]; j-= num; arrayToSort[j] = key; radix bubble shell selection insertion quickarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
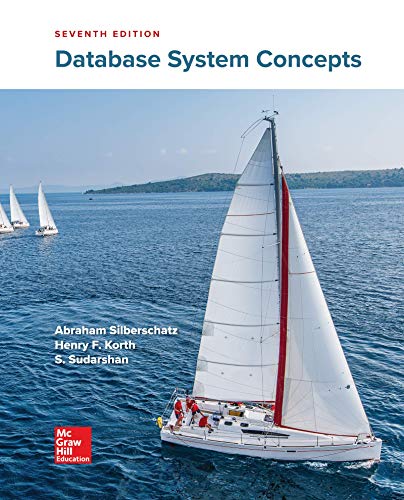
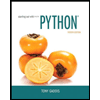
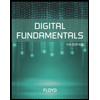
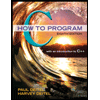
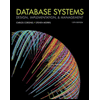
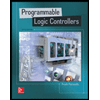