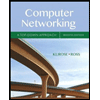
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Need Help Debugging
1. Program produces correct output
2. Method FancyDisplay is overloaded to accept a double
3. Method FancyDisplay is overloaded to accept an int
4. Method FancyDisplay is overloaded to accept a string
// Program demonstrates overloaded methods
// that display an int, an amount of money, or a string
// decorated with an argument character
// or a default character 'X'
using System;
using static System.Console;
using System.Globalization;
class DebugEight4
{
static void Main()
{
FancyDisplay(33);
FancyDisplay(44, "@");
FancyDisplay(55.55);
FancyDisplay(77.77, '*');
FancyDisplay("hello");
FancyDisplay("goodbye", '#');
}
public static void FancyDisplay(int num, char decoration = 'X')
{
WriteLine("{0}{1}{2} {1} {0}{1}{2}\n",
decoration, num);
}
public static void FancyDisply(double num, char decoration = 'X')
{
WriteLine("{0}{0}{0} {0}{0}{0}\n",
decoration, num.ToString("C", CultureInfo.GetCultureInfo("en-US")));
}
public static void FancyDisplay(word, char decoration = 'X')
{
WriteLine("{0}{0}{0} {1} {1}{0}{0}\n",
decoration, word);
}
}
Expert Solution

arrow_forward
Output
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- Write a program in c++ that asks the users to enter a series of single digit numbers with nothing separating them. Read the input as a C-string object. The program should display the sum of all the single-digit numbers in the string. For an example, if the user enters 2518, the program should display 16, which is the sum of 2, 5, 1, and 8. The program should also display the highest and lowest digits in the string.arrow_forwardCode that need debugging fix with no errors // Gets a String from user // Converts the String to lowercase, and // displays the String's length // as well as a count of letters import java.util.*; public class DebugSeven4 { public static void main(String[] args) { Scanner kb = new Scanner(System.in); String aString ; int numLetters = 0; int stringLength; System.out.println("Enter a String. Include"); System.out.println("some uppercase letters, lowercase"); System.out.print("letters, and numbers >> "); aString = kb.nextLine(); stringLength = aString.length(); System.out.print("In all lowercase, the String is: "); for(int i = 0; i <= stringLength; i++) { char ch = Character.toLowerCase(aStringcharAt(i)); System.out.print(ch); if(!Character.isLetter(ch)) numLetters++; } System.out.println(); System.out.println ("The number of CHARACTERS…arrow_forwardin C# Write a program named InputMethodDemo2 that eliminates the repetitive code in the InputMethod() in the InputMethodDemo program in Figure 8-5. Rewrite the program so the InputMethod() contains only two statements: one = DataEntry("first");two = DataEntry("second"); I am getting the error Method DataEntry is defined to eliminate repetitive code 0 out of 1 checks passed. unit Test Incomplete Method DataEntry prompts the user to enter an integer and returns the integer Build Status Build Failed Build Output Compilation failed: 1 error(s), 0 warnings NtTest37b77fb0.cs(21,47): error CS0234: The type or namespace name `DataEntry' does not exist in the namespace `InputMethodDemo2'. Are you missing an assembly reference? Test Contents [TestFixture] public class DataEntryMethodTest { [Test ] public void DataEntryTest() { string consoleInput = "97"; int returnedValue; string expectedString = "Enter third integer"; using (var inputs = new StringReader(consoleInput)) {…arrow_forward
- String personName is read from input. Output personName left aligned with a width of 6, followed by " Taylor". End with a newline. Ex: If the input is Juno, then the output is: Juno Taylor Use Javaarrow_forwardThe countSubstring function will take two strings as parameters and will return an integer that is the count of how many times the substring (the second parameter) appears in the first string without overlapping with itself. This method will be case insensitive. For example: countSubstring(“catwoman loves cats”, “cat”) would return 2 countSubstring(“aaa nice”, “aa”) would return 1 because “aa” only appears once without overlapping itself. public static int countSubstring(String s, String x) { if (s.length() == 0 || x.length() == 0) return 1; if (s.length() == 1 || x.length() == 1){ if (s.substring(0,1).equals(x.substring(0,1))){ s.replaceFirst((x), " "); return 1 + countSubstring(s.substring(1), x); } else { return 0 + countSubstring(s.substring(1), x); } } return countSubstring(s.substring(0,1), x) + countSubstring(s.substring(1), x); } public class Main { public static void main(String[] args) { System.out.println(Recursion.countSubstring("catwoman loves cats","cat"));…arrow_forwardC++ write an application that enable users to enter student id and three exam scores. Provide a method to compute and return overall exam average. Provide another method that prints all score and average value formatted with no digits to the right of the decimal. Use single method to enter all dataarrow_forward
- NEED HELP PYTHON ONLY PLZZarrow_forwardIn C programming language. Objectives:• Use of functions and passing by value and reference.• Use of selection constructs.• Use of repetition constructs (loops).• The use of the random number generator and seeding.• Display formatted output using input/outputstatements.• Use format control strings to format text output. Description: For this project you are tasked with building a user application that will select sets of random numbers. Your application must use functions and pass values and pointers. Your program will pick sets of 6 random numbers with values of 1 to 53. The user should be able to choose how many sets to produce. The challenge will be to ensure that no number in a set of 6 numbers is a repeat. The number can be in another set just not a duplicate in the same set of 6. Your program should prompt the user and ask them how many number sets they wish to have generated. The program should not exit unless the user enters a value indicating they wish to quit. Minimum…arrow_forwardScala Programming: Given string str, write a Scala program to create a new string where "Scala " is added to the front of a given string. If the string already begins with "Scala", return the string unchanged. Otherwise, return the string after adding "Scala ". Test the function for two strings "Scala Programming" and "Programming" inside the main method.arrow_forward
- JAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forwardThe files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { publicstaticvoidmain(String[] args) { Scanner input =newScanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145,190,235}; char plan; int x, fp =99; String prompt ="Please select a floor plan\n"+ "Our floorPlanss are:\n"+"A - Augusta, a ranch\n"+ "B - Brittany, a split level\n"+ "C - Colonial, a two-story\n"+ "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x =0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp =99) System.out.println("Invalid floor plan code entered")); else { if(fp…arrow_forward16arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
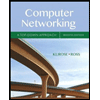
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
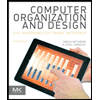
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
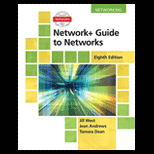
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
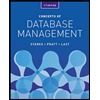
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
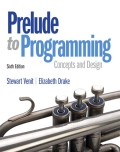
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
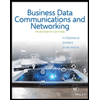
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY