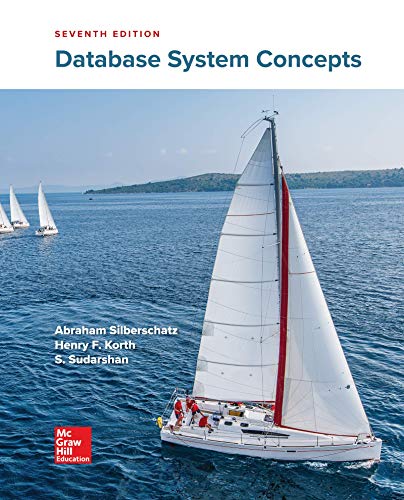
Concept explainers
I need help with this code
Python:
import random
from tabulate import tabulate
#select the marks
gradePoints = {"A":4,"B":3,"C":2,"D":1,"F":0}
courseList = ["CST 161","Mat 144","ENG 201","PSY 101","HIS 101"]
gradeList = ["A","B","C","D","F"]
creditList = [3,4]
data = []
total_credit = 0
sums = 0
#input the number of course that you want to select randomly
n = int(input("Enter the number of courses : "))
for i in range(n):
temp = []
#for courses
k = random.randint(0,4)
temp.append(courseList[k])
#for credits
k = random.randint(0,1)
total_credit += creditList[k]
temp.append(creditList[k])
#for grades
k = random.randint(0,4)
temp.append(gradeList[k])
#for gradepoints
sums += gradePoints[gradeList[k]] * temp[1]
temp.append(gradePoints[gradeList[k]] * temp[1])
data.append(temp)
print(tabulate(data,headers = ["Course","Credits","Grade","Value Per Course"]))
print(f"Total credits taken: {total_credit}")
print(f"Total quality points earned : {sums}")
print(f"Grade : {round(total_credit/sums,2)}")
![Write a program that will take this dictionary:
gradePoints = {"A":4,"B":3,"C":2,"D":1,"F":0}
and this list:
courseList=["CST 161","Mat 144", "ENG 201","PSY 101","HIS 101"]
and randomly use elements from each of these lists:
gradeList = ["A","B","CD","F"]
creditList = [3,4]
and produce a grade point average.
Academic average is determined by dividing the total number of quality
points earned by the total number of credits taken, whether passed or
failed. The following table is an illustration of this computation:
GRADE & QUALITY NUMERICAL POINTS
Course... Credits Value Per Course
HIS 101...3 C (2) 6
PSY 101...3 B (3) 9
MAT 144....4 D (1) 4
ENG 201.... 3 B+ (3.5) 10.5
CST 161.....3 F (0) 0
Total credits taken: 16
Total quality points earned 29.5
29.5 divided by 16 results in a 1.8 grade
point average.
Here the gradeList element will be embedded as the key in referencing the grade points.
(e.g.gPoints = gradePoints[random gradeList element])
(e.g. creditHours = random creditList element).
To get a random list element, use the randint method with a range of (0,len(list)).
That will be multiplied by the gPoints per course.
Multiply them to get the the valuePerCourse for the that course.
(e.g.valuePerCourse = (creditHours" gPoints), this will yield the value for that course.
For each course (each pass through the loop).
add creditHours to totalCredits and
add valuePerCourse to qualityPoints
qualityPoints divided by total Credits results in a GPA.
Display each course with their grade, and the value per course.
Display the quality points and the GPA.
Display the proper string literals that will identify your output.
After you are done, Add the logic to read the Courses, grades and credits from a file.
Ex.
ABB
А
CST 161
ENG 101
MAT 119
Process these courses, grades and credits in your program instead of using the courseList, gradeList and creditList.
434](https://content.bartleby.com/qna-images/question/2b5ce93e-94f9-49fd-8fc5-aabefed0184c/058fdf08-821c-40e9-9e6e-924dd7617d6a/bvz0gc_thumbnail.png)
![p?
QU
nage
cion
Advanced Dictionary.py
File Edit Format Run Options Window Help
import random
from tabulate import tabulate
#select the marks
que sums=0
- C:/Users/Justin/Desktop/Fall 2022/cor File
gradePoints = {"A":4,"B":3,"C":2,"D":1,"
courseList = ["CST 161", "Mat 144", "ENG 2
gradeList = ["A", "B","C", "D","F"]
creditList = [3,4]
data= []
total credit = 0
#input the number of course that you wan
n = int (input ("Enter the number of cours
for i in range (n) :
temp = []
#for courses
k = random. randint (0,4)
temp. append (courseList[k])
#for credits
k = random.randint (0,1)
total_credit += creditList [k]
temp.append(creditList[k])
#for grades
k = random.randint (0,4)
temp.append (gradeList[k])
#for gradepoints
sums += grade Points [gradeList[k]] *
temp.append (gradePoints [gradeList[k]
data.append (temp)
print (tabulate (data, headers = ["Course",
print (f"Total credits taken: {total_cred
print (f"Total quality points earned : {s
print (f"Grade: {round (total_credit/sums
IDLE Shell 3.10.7
Edit Shell Debug Options Window Help
Python 3.10.7 (tags/v3.10.7:6cc6b13, Sep 5 2022, 14:08:36) [MSC v.193
3 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license ()" for more informatio
n.
>>>
>>>
RESTART: C:/Users/Justin/Desktop/Fall 2022/computer programin fundam
ental/Advanced Dictionary.py
Traceback (most recent call last):
File "C:/Users/Justin/Desktop/Fall 2022/computer programin fundament
al/Advanced Dictionary.py", line 2, in <module>
from tabulate import tabulate
ModuleNotFoundError: No module named 'tabulate'](https://content.bartleby.com/qna-images/question/2b5ce93e-94f9-49fd-8fc5-aabefed0184c/058fdf08-821c-40e9-9e6e-924dd7617d6a/ttcluze_thumbnail.png)

Step by stepSolved in 4 steps with 2 images

- 7bact2 Please help me answer this in python programming.arrow_forwardPythonarrow_forwardhelp with python code import time timeStamp = time.ctime()print(timeStamp)forum_list = [ {'date':'10-01-2022', 'name':'Student1', 'comment':'Very helpful'}, {'date': '10-01-2022', 'name': 'Student2', 'comment': 'Very good'} ] menu = """ Forum Comments 0: Exit 1: Display Comments 2: Add Comment """ done = False while not done: print(menu) selection = input('Please make a selection: ') if selection == "0": done = True print('Exiting now, thank you for participating') if selection == "1": for d in forum_list: print(d['date'], end='') print(' ', end='') print(d['name']) print(d['comment']) if selection == "2": comment = {} comment['date'] = input('Please enter date: ') comment['name'] = input('Please enter name: ') comment['comment'] = input('Please enter comment: ') forum_list.append(comment) # what's inside the data.txt file #Date Name…arrow_forward
- The chapter is nested lists Create code in python using for loops Thanks!arrow_forwardPlease help. This is Python I feel so lostarrow_forwardIn python, Problem Description:You are hosting a party and do not have room to invite all of your friends. You use the following unemotional mathematical method to determine which friends to invite. Number your friends 1, 2, . . . , K and place them in a list in this order. Then perform m rounds. In each round, use a number to determine which friends to remove from the ordered list. The rounds will use numbers r1, r2, . . . , rm. In round i remove all the remaining people in positions that are multiples of ri (that is, ri, 2ri, 3ri, . . .) The beginning of the list is position 1. Output the numbers of the friends that remain after this removal process. Input Specification:The first line of input contains the integer K (1 ≤ K ≤ 100). The second line of input contains the integer m (1 ≤ m ≤ 10), which is the number of rounds of removal. The next m lines each contain one integer. The ith of these lines (1 ≤ i ≤ m) contains ri ( 2 ≤ ri ≤ 100) indicating that every person at a position…arrow_forward
- Python code please help, indentation would be greatly appreciatedarrow_forwardpython LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forwardUsing Python This assignment is about temperatures and requires the main function and a custom value-returning function. The value-returning function takes a list of random Fahrenheit temperatures as its only argument and returns a smaller list of temperatures that are below freezing. The main function needs these steps in this sequence: create an empty list that will the hold Fahrenheit temperatures. use a loop to add 25 random integertemperatures to the list. All temperatures should be between 5 and 75, inclusive. use another loop to display all 25 temperatures on one line separated by spaces. report the highest and lowest temperatures in the list. 32 might be in the list. Report the index of the first instance of 32 or report that it didn't make the list. using slice syntax: print the first 10 temperatures in the list. print the middle 5 temperatures in the list. print the final 10 temperatures in the list. execute the custom value-returning function with the complete list as…arrow_forward
- in pythonarrow_forwardİn C Language pls help #include <stdio.h>#include <stdlib.h>#include <string.h> typedef struct ArrayList_s{ void **list; int size; int capacity; int delta_capacity;} ArrayList_t, *ArrayList; ArrayList arraylist_create(int capacity, int delta_capacity){ ArrayList l; l = (ArrayList)malloc(sizeof(ArrayList_t)); if (l != NULL) { l->list = (void **)malloc(capacity * sizeof(void *)); if (l->list != NULL) { l->size = 0; l->capacity = capacity; l->delta_capacity = delta_capacity; } else { free(l); l = NULL; } } return l;} void arraylist_destroy(ArrayList l){ free(l->list); free(l);} int arraylist_isempty(ArrayList l){ return l->size == 0;} int arraylist_isfull(ArrayList l){ return l->size == l->capacity;} int arraylist_set(ArrayList l, void *e, int index, void **replaced){ if (!arraylist_isfull(l)) {…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
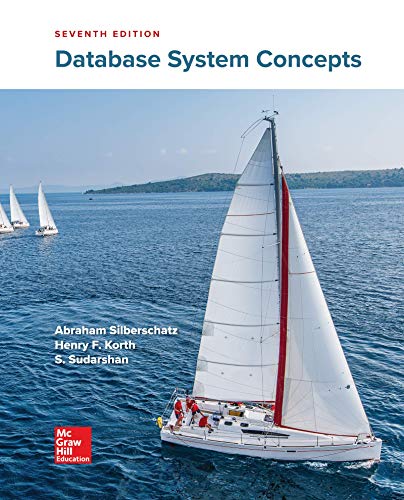
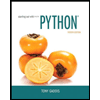
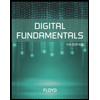
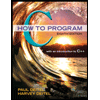
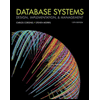
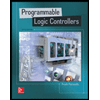