ODDITERATOR AND ODDITERABLE OddIterator is an Iterator of type Integer OddIterable is an Iterable of type Integer In OddIterator, the elements returned by the Iterator are the odd numbers in a given list. Since OddIterator is an Iterator, we will want to include the following methods in the class: o +OddIterator(Iterable) A constructor which is passed in the Integer iterable list to iterate over. Tip: note that the list is really an Iterable of type Integer. The private member iter of this class is assigned as an iterator to the passed in Iterable o +hasNext() method is required by the Iterator interface. This method returns true if there are more elements to be returned by the iterator (i.e there are still odd numbers in the list). Or the method returns false when there are no more odd numbers left in the list. o +next() method is required by the Iterator interface. This method returns the next element from the list that is an odd number. Tip: do not forget to throw a NoSuchElementException if Tip: feel free to add your own private attribute and protected or private methods in this class. After having written the OddIterator, we also want to create the OddIterable class; which is only responsible for producing an iterator when called upon. This class requires two methods: a constructor, plus the iterator method itself: o +OddIterable(Iterable) A constructor which is passed in an Integer iterable list to be assigned to the private member variable of this class named iter. o +iterator() simply returns an object of the OddIterator class, instantiating it with Iterable iter. Tip: since almost all Java collection-related code uses the java.util package in some way, all of the classes that you write for this assignment are likely to need import java.util.*; at the top. Below are examples of the result of a completed OddIterator and OddIterable: Ex. 1 LinkedList list = new LinkedList(); Collections.addAll(list, -33, -6, 1, 2, 3, 4, 5, 6, 100, 3000, 99); for(Integer i : new OddIterable(list)) { System.out.print(i + " "); } System.out.println(); Output: -33 1 3 5 99 Ex. 2 ArrayList list = new ArrayList(); Collections.addAll(list, 1, 3, 2); for(Integer i : new OddIterable(list)) { System.out.print(i + " "); } System.out.println(); Output: 1 3 EVENITERABLE AND EVENITERATOR PRIMEITERABLE AND PRIMEITERATOR EvenIterator is an Iterator of type Integer EvenIterable is an Iterable of type Integer PrimeIterator is an Iterator of type Integer PrimeIterable is an Iterable of type Integer In EvenIterator, the elements returned by the Iterator are the even numbers in a given In PrimeIterator, the elements returned by the Iterator are the prime numbers in a given Everything should be implemented in a similar fashion to OddIterator and OddIterable. Below are examples of the result of a completed EvenIterator/Iterable and the PrimeIterator/Iterable: Ex. 1 LinkedList list = new LinkedList(); Collections.addAll(list, -33, -6, 1, 2, 3, 4, 5, 6, 100, 3000, 99); for(Integer i : new EvenIterable(list)) { System.out.print(i + " "); } System.out.println(); Output: -6 2 4 6 100 3000 Ex. 2 ArrayList list = new ArrayList(); Collections.addAll(list, -33, -6, 1, 2, 3, 4, 5, 6, 100, 3000, 99); for(Integer i : new PrimeIterable(list)) { System.out.print(i + " "); } System.out.println(); Output: 2 3 5 TEACHSIMPLEMATH TeachSimpleMath is a class that has one-member variable named collection of type Collection of +TeachSimpleMath(Collection) is a parametrized constructor used to initialize the class attribute collection. +printOdd() is a void method which uses a for-each loop to print only the odd numbers in the attribute collection. This method uses System.out.print(), each odd number is printed with a space and a new line is printed at the very end. i.e 1 3 5 7 [newline] +printEven() is a void method which uses a for-each loop to print only the even numbers in the attribute collection. This method uses System.out.print() and prints in the similar fashion to the above. +printPrime() is a void method which uses a for-each loop to print only the prime numbers in the attribute collection. This method uses System.out.print() and prints in the similar fashion to the above. Below are examples of the result of a completed TeachSimpleMath class: Ex. 1 LinkedList list = new LinkedList(); Collections.addAll(list, -33, -6, 1, 2, 3, 4, 5, 6, 100, 3000, 99); TeachSimpleMath obj = new TeachSimpleMath(list); obj.printOdd(); obj.printEven(); obj.printPrime(); Output: -33 1 3 5 99 -6 2 4 6 100 3000 2 3 5
ODDITERATOR AND ODDITERABLE
- OddIterator is an Iterator of type Integer
- OddIterable is an Iterable of type Integer
- In OddIterator, the elements returned by the Iterator are the odd numbers in a given list.
- Since OddIterator is an Iterator, we will want to include the following methods in the class:
o +OddIterator(Iterable) A constructor which is passed in the Integer iterable list to iterate over.
- Tip: note that the list is really an Iterable of type Integer. The private member iter of this class is assigned as an iterator to the passed in Iterable
o +hasNext() method is required by the Iterator interface. This method returns true if there are more elements to be returned by the iterator (i.e there are still odd numbers in the list). Or the method returns false when there are no more odd numbers left in the list.
o +next() method is required by the Iterator interface. This method returns the next element from the list that is an odd number.
- Tip: do not forget to throw a NoSuchElementException if
- Tip: feel free to add your own private attribute and protected or private methods in this class.
- After having written the OddIterator, we also want to create the OddIterable class; which is only responsible for producing an iterator when called upon. This class requires two methods: a constructor, plus the iterator method itself:
o +OddIterable(Iterable) A constructor which is passed in an Integer iterable list to be assigned to the private member variable of this class named iter.
o +iterator() simply returns an object of the OddIterator class, instantiating it with Iterable iter.
Tip: since almost all Java collection-related code uses the java.util package in some way, all of the classes that you write for this assignment are likely to need import java.util.*; at the top.
Below are examples of the result of a completed OddIterator and OddIterable:
Ex. 1
LinkedList<Integer> list = new LinkedList<Integer>(); Collections.addAll(list, -33, -6, 1, 2, 3, 4, 5, 6, 100, 3000, 99); for(Integer i : new OddIterable(list))
{
System.out.print(i + " ");
}
System.out.println();
Output: -33 1 3 5 99
Ex. 2
ArrayList<Integer> list = new ArrayList<Integer>(); Collections.addAll(list, 1, 3, 2);
for(Integer i : new OddIterable(list))
{
System.out.print(i + " ");
}
System.out.println();
Output: 1 3
EVENITERABLE AND EVENITERATOR PRIMEITERABLE AND PRIMEITERATOR
- EvenIterator is an Iterator of type Integer
- EvenIterable is an Iterable of type Integer
- PrimeIterator is an Iterator of type Integer
- PrimeIterable is an Iterable of type Integer
- In EvenIterator, the elements returned by the Iterator are the even numbers in a given
- In PrimeIterator, the elements returned by the Iterator are the prime numbers in a given
- Everything should be implemented in a similar fashion to OddIterator and OddIterable.
Below are examples of the result of a completed EvenIterator/Iterable and the PrimeIterator/Iterable:
Ex. 1
LinkedList<Integer> list = new LinkedList<Integer>(); Collections.addAll(list, -33, -6, 1, 2, 3, 4, 5, 6, 100, 3000, 99); for(Integer i : new EvenIterable(list))
{
System.out.print(i + " ");
}
System.out.println(); Output: -6 2 4 6 100 3000
Ex. 2
ArrayList<Integer> list = new ArrayList<Integer>(); Collections.addAll(list, -33, -6, 1, 2, 3, 4, 5, 6, 100, 3000, 99); for(Integer i : new PrimeIterable(list))
{
System.out.print(i + " ");
}
System.out.println();
Output: 2 3 5
TEACHSIMPLEMATH
- TeachSimpleMath is a class that has one-member variable named collection of type Collection of
- +TeachSimpleMath(Collection) is a parametrized constructor used to initialize the class attribute collection.
- +printOdd() is a void method which uses a for-each loop to print only the odd numbers in the attribute collection. This method uses System.out.print(), each odd number is printed with a space and a new line is printed at the very end. i.e 1 3 5 7 [newline]
- +printEven() is a void method which uses a for-each loop to print only the even numbers in the attribute collection. This method uses System.out.print() and prints in the similar fashion to the above.
- +printPrime() is a void method which uses a for-each loop to print only the prime numbers in the attribute collection. This method uses System.out.print() and prints in the similar fashion to the above.
Below are examples of the result of a completed TeachSimpleMath class:
Ex. 1
LinkedList<Integer> list = new LinkedList<Integer>(); Collections.addAll(list, -33, -6, 1, 2, 3, 4, 5, 6, 100, 3000, 99); TeachSimpleMath obj = new TeachSimpleMath(list); obj.printOdd();
obj.printEven(); obj.printPrime();
Output:
-33 1 3 5 99
-6 2 4 6 100 3000
2 3 5
Ex. 2
Collections.addAll(list, 2039, 2161, 9551, -9551, 9967, 1, 0, -1, -2, -3, -100, 100, 92);
TeachSimpleMath obj = new TeachSimpleMath(list); obj.printOdd();
obj.printEven(); obj.printPrime();
Output:
2039 2161 9551 -9551 9967 1 -1 -3
0 -2 -100 100 92
2039 2161 9551 9967
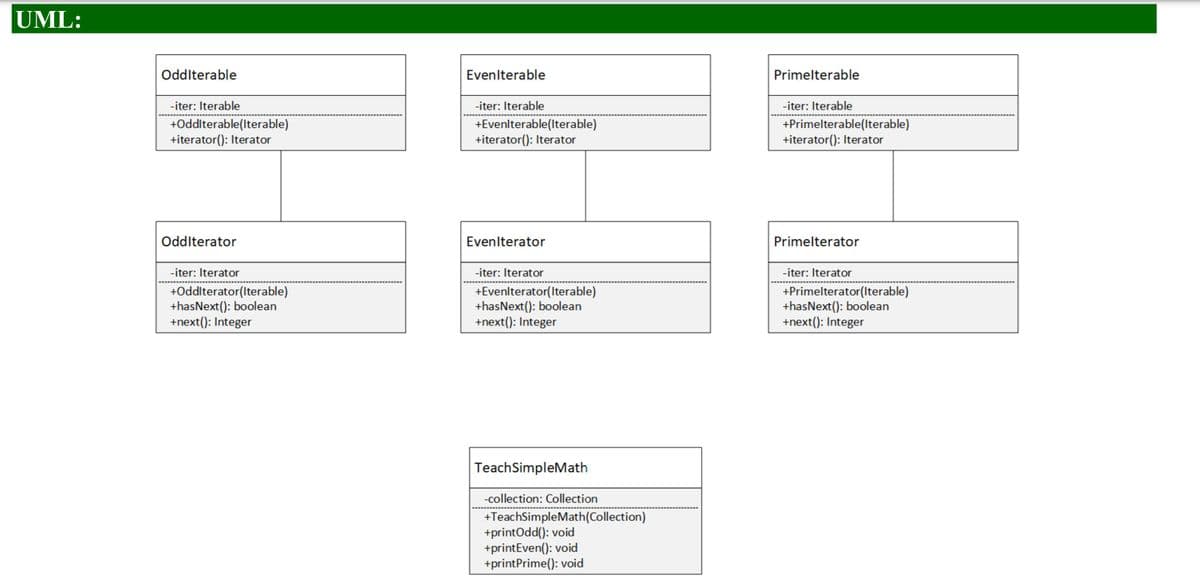

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

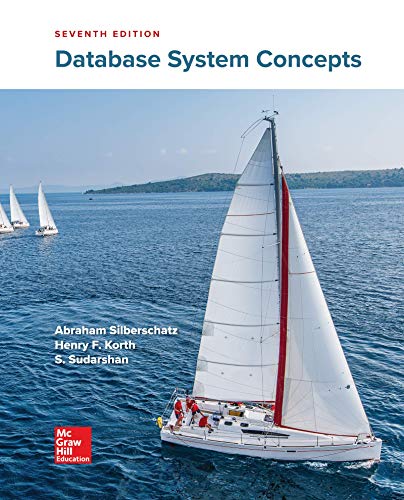
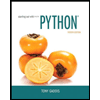
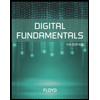
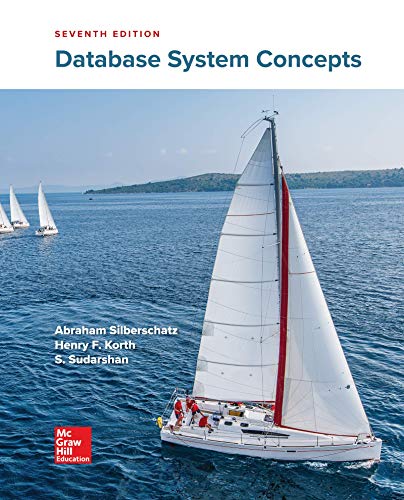
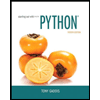
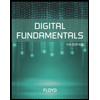
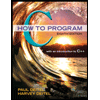
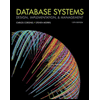
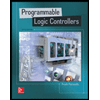