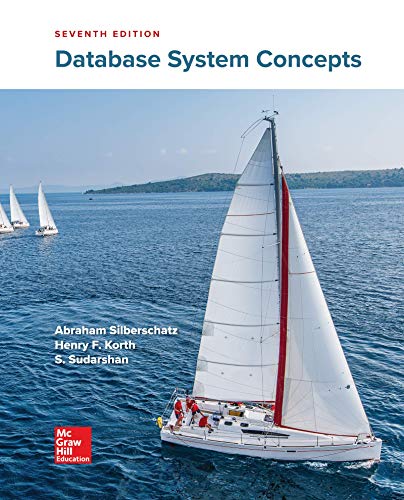
please be as clear as you can.
Write a C++ program, named "average.cpp". Your program should:
- Prompt the user to enter the name of a data file
- Open and read the data stored in the file while computing the average, as well as finding the minimum and maximum value in the file
- After reading the file, close the file, and display the values read, the minimum, maximum, and average of the values
Testing and Compilation
Since your program will be reading from a data file, first copy the data file into your working directory. You can do this like so:
ranger0$ cp $PUB/lab5-*.dat ./ This command will copy two data files (lab5-1.dat and lab5-2.dat) into your working directory.
After copying the data files into your working directory, compile your code like so:
ranger0$ g++ average.cpp -o average Here is an example execution of the program using the first data file: Enter the name of the data file: lab5-1.dat The values read are: 1 30 2 -40 3 25 4 64 5 89 6 103 7 45 8 89 9 34 10 -2 11 15 12 63 12 values are read. There are 7 values greater than its previous value. The largest is 103. The smallest is -40. The average is 42.92.
Here is an example run of the program using the second data file:
Enter the name of the data file: lab5-2.dat The values read are: 1 500 2 430 3 240 4 -390 5 -230 6 100 7 394 8 444 9 882 10 -30 11 -29 12 683 13 732 14 990 15 -538 16 93 16 values are read. There are 10 values greater than its previous value. The largest is 990. The smallest is -538. The average is 266.94.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- Using C++, create a program that reads integers from the file named "123456" and performs the following actions: Define a function named "calculateFileStats" that reads all the integers from the file and displays the following on the console: -The total sum of all numbers in the file -The minimum value of the numbers in the file -The maximum value of the numbers in the file -The average value with 2 decimal places. If the program fails to access the file, display an error message to the user and terminate the program. Close the file once you have finished reading it. Call the function "calculateFileStats" in main. Note: I did not forget to attach an input file. Use your own input code. I am interested in your code. sample run: Total: 30309 Minimum: 1 Maximum: 10 Average: 5.58arrow_forwardHi, c++ help Please see my code The code reads two numbers from a file and creates a dice which must be played a certain amount of times (dertimined by input file). The code must then output the results of the multiple dice rolls. For line 1 **the numbers in brackets are the results of the multiple dice roll while the last number is the sum of the throws for the first die As you can see for the first line everything adds up correctly as the results of the dice (in brackets) are summed up correctly (last number) For the second row and all other following rolls, the code adds the rolls of the new dice and the result of the previous sum to get the new sum. This should not happen, I want the sum of each rows to be displayed on the end of each line The correct code should be display **please don't output comment [2] - [4 ]-[5] - 11 //dice rolled 3 times and sum is 11 [1] - [1 ]-2 //dice rolled 2 times with sum 2arrow_forwardTopical Information Use C++. This lab should provide you with practice in file handling, libraries, and random variate generation. Program Information Write a program that allows the user to create files of random data. They should be offered a menu of possibilities: 1) create random Whole number data file 2) create random Decimal number data file 3) create random Character data file 4) Quit program The first 3 options should ask for appropriate bounds. Your program should make sure they are in order before passing them to your random value generation function. Then, ask how many random values to generate and what file to place them in. Assuming the file opened correctly, proceed to generate all requested random values. The menu should continue to loop until the quit option is chosen. Options should be choosable by either the number or the capitalized word. (For example, to quit, they should be able to enter 4, Q, or q.)arrow_forward
- qerwr2arrow_forward1. Write a program in C/C++ that reads a table of data from an input file and displays the data. The program then prompts the user to see the data in specific order. The output of your program should display the sorted data (descending order) according to the user selection. Your program should display the prompt repeatedly until the user wants to exit. See the test run. The input file contains four data fields: Country, Total cases, New cases, and Total deaths. The number of rows (data) can vary between 5 to 100. You must use Quick sort in your implementation. Example test run: Country Total Cases New Cases Total Deaths USA 48072665 70590 784775 India 34447536 10560 463655 Brazil 21960766 2799 611384 UK…arrow_forwardC++ How do I read initial data in from a file, and then use class to store the data?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
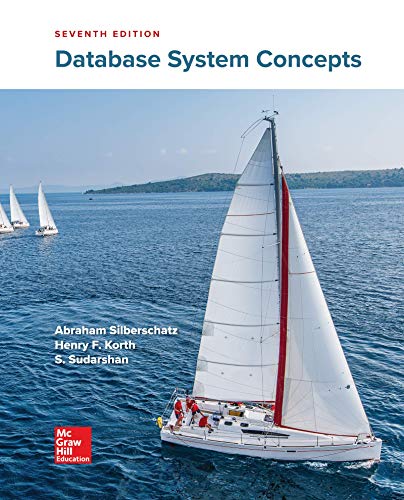
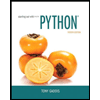
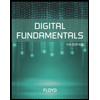
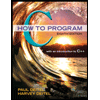
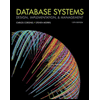
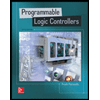