Prime Number List Use the isPrime method that you wrote in Programming Challenge 13 in a program that prompts the user to enter an integer number, then displays all prime numbers less than the user number separated by a space. Here is a working code but please fix it so it will in Hypergrade which as all the test casses. I DO NOT NEED THANK YOU IN THE PROGRAM. IT HAS TO PASS ALL THE TEST CASSES PLEASE. THANK YOU!!!!!: import java.util.Scanner; public class PrimeNumberList { // Method to check if a number is prime public static boolean isPrime(int num) { if (num <= 1) { return false; } for (int i = 2; i <= Math.sqrt(num); i++) { if (num % i == 0) { return false; } } return true; } public static void main(String[] args) { Scanner scanner = new Scanner(System.in); // Prompt the user to enter a number System.out.print("Enter a number: \n"); int userInput = scanner.nextInt(); // Display prime numbers less than the user's number System.out.print("Prime numbers are " + userInput + ": "); for (int i = 2; i < userInput; i++) { if (isPrime(i)) { System.out.print(i + " "); } } // Close the scanner scanner.close(); } } Test Case 1 Enter a number: \n 10ENTER 2 3 5 7 Test Case 2 Enter a number: \n 100ENTER 2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97
import java.util.Scanner;
public class PrimeNumberList {
// Method to check if a number is prime
public static boolean isPrime(int num) {
if (num <= 1) {
return false;
}
for (int i = 2; i <= Math.sqrt(num); i++) {
if (num % i == 0) {
return false;
}
}
return true;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Prompt the user to enter a number
System.out.print("Enter a number: \n");
int userInput = scanner.nextInt();
// Display prime numbers less than the user's number
System.out.print("Prime numbers are " + userInput + ": ");
for (int i = 2; i < userInput; i++) {
if (isPrime(i)) {
System.out.print(i + " ");
}
}
// Close the scanner
scanner.close();
}
}
Test Case 1
10ENTER
2 3 5 7
Test Case 2
100ENTER
2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

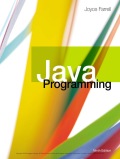
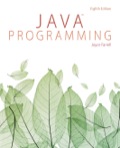
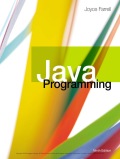
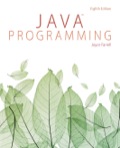
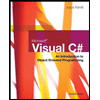