Programming Logic & Design Comprehensive
9th Edition
ISBN: 9781337669405
Author: FARRELL
Publisher: Cengage
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
- Needs to be written in C
![Problem #1 - Initializing and summing an array using for loops
Write a program which creates a one dimensional, array of int type with 10 elements. Name the array "data[]". Initialize the array using a for loop such that each element is its index number
plus 10. For example, data[0] will be a value of 10, data[1] will be 11, etc. Print out all the elements of the array using two columns. The first column is the index and the second column is
the value of the element. After printing out the index and values, print out the sum of all the elements of the array. Use a for loop to sum all the elements. Make the output look like this
screen shot. Name the file "array_sum.c"
Index
Value
10
11
12
3
13
14
15
16
17
18
9
19
145
Sum
Process returned 0
Press any key to co
Problem #2 - Initialize an array and compute the average
Write a program which creates a one dimensional array of float type with 5 elements. Name the array "data[]". Initialize the array when it is created with these values: 2.5, 3.33, 4.2, 8.0, 5.1.
Compute the average of all the elements in the array using a for loop to sum the array. Print out all the elements of the array using two columns. The first column is the index and the
second column is the value of the element. After printing out the index and values, print out the average of all the elements of the array. Make the output look like this screen shot. Name
the file "array_average.c"
Index
Value
2.500000
1
3.330000
4.200000
12
3
4
Avg
8.000000
5.100000
4.626000
Process returned 0
Press any key to co](https://content.bartleby.com/qna-images/question/b488d958-b9f8-4820-a61d-1a1d881a5d5d/96f9d993-2d4c-4a20-a8a9-586c58ecbbe4/zku057i_thumbnail.png)
Transcribed Image Text:Problem #1 - Initializing and summing an array using for loops
Write a program which creates a one dimensional, array of int type with 10 elements. Name the array "data[]". Initialize the array using a for loop such that each element is its index number
plus 10. For example, data[0] will be a value of 10, data[1] will be 11, etc. Print out all the elements of the array using two columns. The first column is the index and the second column is
the value of the element. After printing out the index and values, print out the sum of all the elements of the array. Use a for loop to sum all the elements. Make the output look like this
screen shot. Name the file "array_sum.c"
Index
Value
10
11
12
3
13
14
15
16
17
18
9
19
145
Sum
Process returned 0
Press any key to co
Problem #2 - Initialize an array and compute the average
Write a program which creates a one dimensional array of float type with 5 elements. Name the array "data[]". Initialize the array when it is created with these values: 2.5, 3.33, 4.2, 8.0, 5.1.
Compute the average of all the elements in the array using a for loop to sum the array. Print out all the elements of the array using two columns. The first column is the index and the
second column is the value of the element. After printing out the index and values, print out the average of all the elements of the array. Make the output look like this screen shot. Name
the file "array_average.c"
Index
Value
2.500000
1
3.330000
4.200000
12
3
4
Avg
8.000000
5.100000
4.626000
Process returned 0
Press any key to co
![Problem #3 - Search a string
Write a program which counts the number of a particular character found in a string. For example, in the string "double down" there are two 'd' characters. Use a loop to go through the
string from beginning to end and compare each character in the string with the character to find. If the character is found, increment a count. Print out the string and the number found. See
the screen shot below. The string is "Find the letter i in this string." The character to find and count is 'i'. Name the file "string_search.c".
The typical way to go through a string from beginning to end is using a while loop. The test in the loop checks if the current element in the loop is a NULL character. If a NULL is found, stop
looping since the end of the string has been found. For example,
i = 0;
while(str[i] != '\0') {
}
Of course, i must be incremented to go through the string each time through the loop.
String: Find the letter i in this string.
Count: 5
Process returned 0 (0x0)
Press any key to continue.
execution time : 0.081 s](https://content.bartleby.com/qna-images/question/b488d958-b9f8-4820-a61d-1a1d881a5d5d/96f9d993-2d4c-4a20-a8a9-586c58ecbbe4/gipv93j_thumbnail.png)
Transcribed Image Text:Problem #3 - Search a string
Write a program which counts the number of a particular character found in a string. For example, in the string "double down" there are two 'd' characters. Use a loop to go through the
string from beginning to end and compare each character in the string with the character to find. If the character is found, increment a count. Print out the string and the number found. See
the screen shot below. The string is "Find the letter i in this string." The character to find and count is 'i'. Name the file "string_search.c".
The typical way to go through a string from beginning to end is using a while loop. The test in the loop checks if the current element in the loop is a NULL character. If a NULL is found, stop
looping since the end of the string has been found. For example,
i = 0;
while(str[i] != '\0') {
}
Of course, i must be incremented to go through the string each time through the loop.
String: Find the letter i in this string.
Count: 5
Process returned 0 (0x0)
Press any key to continue.
execution time : 0.081 s
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- (Statistics) a. Write a C++ program that reads a list of double-precision grades from the keyboard into an array named grade. The grades are to be counted as they’re read, and entry is to be terminated when a negative value has been entered. After all grades have been input, your program should find and display the sum and average of the grades. The grades should then be listed with an asterisk (*) placed in front of each grade that’s below the average. b. Extend the program written for Exercise 1a to display each grade and its letter equivalent, using the following scale: Between90and100=AGreaterthanorequalto80andlessthan90=BGreaterthanorequalto70andlessthan80=CGreaterthanorequalto60andlessthan70=DLessthan60=Farrow_forward(Electrical eng.) a. An engineer has constructed a two-dimensional array of real numbers with three rows and five columns. This array currently contains test voltages of an amplifier. Write a C++ program that interactively inputs 15 array values, and then determines the total number of voltages in these ranges: less than 60, greater than or equal to 60 and less than 70, greater than or equal to 70 and less than 80, greater than or equal to 80 and less than 90, and greater than or equal to 90. b. Entering 15 voltages each time the program written for Exercise 7a runs is cumbersome. What method could be used for initializing the array during the testing phase? c. How might the program you wrote for Exercise 7a be modified to include the case of no voltage being present? That is, what voltage could be used to indicate an invalid voltage, and how would your program have to be modified to exclude counting such a voltage?arrow_forward(Numerical) Given a one-dimensional array of integer numbers, write and test a function that displays the array elements in reverse order.arrow_forward
- (Electrical eng.) Write a program that specifies three one-dimensional arrays named current, resistance, and volts. Each array should be capable of holding 10 elements. Using a for loop, input values for the current and resistance arrays. The entries in the volts array should be the product of the corresponding values in the current and resistance arrays (sovolts[i]=current[i]resistance[i]). After all the data has been entered, display the following output, with the appropriate value under each column heading: CurrentResistance Voltsarrow_forward(Electrical eng.) Write a program that declares three one-dimensional arrays named volts, current, and resistance. Each array should be declared in main() and be capable of holding 10 double-precision numbers. The numbers to store in current are 10.62, 14.89, 13.21, 16.55, 18.62, 9.47, 6.58, 18.32, 12.15, and 3.98. The numbers to store in resistance are 4, 8.5, 6, 7.35, 9, 15.3, 3, 5.4, 2.9, and 4.8. Your program should pass these three arrays to a function named calc_volts(), which should calculate elements in the volts array as the product of the corresponding elements in the current and resistance arrays (for example ,volts[1]=current[1]resistance[1]). After calc_volts() has passed values to the volts array, the values in the array should be displayed from inside main().arrow_forward(Program) Write a declaration to store the following values in an array named rates: 12.9, 18.6, 11.4, 13.7, 9.5, 15.2, and 17.6. Include the declaration in a program that displays the values in the array by using pointer notation.arrow_forward
- (Data processing) Write a program that uses an array declaration statement to initialize the following numbers in an array named slopes: 17.24, 25.63, 5.94, 33.92, 3.71, 32.84, 35.93, 18.24, and 6.92. Your program should locate and display the maximum and minimum values in the array.arrow_forward(Numerical) a. Define an array with a maximum of 20 integer values, and fill the array with numbers input from the keyboard or assigned by the program. Then write a function named split() that reads the array and places all zeros or positive numbers in an array named positive and all negative numbers in an array named negative. Finally, have your program call a function that displays the values in both the positive and negative arrays. b. Extend the program written for Exercise 6a to sort the positive and negative arrays into ascending order before they’re displayed.arrow_forward(Data processing) a. Write a program to input 10 integer numbers in an array named fmax and determine the maximum value entered. Your program should contain only one loop, and the maximum should be determined as array element values are being input. (Hint: Set the maximum equal to the first array element, which should be input before the loop used to input the remaining array values.) b. Repeat Exercise 8a, keeping track of both the maximum element in the array and the index number for the maximum. After displaying the numbers, print these two messages (replacing the underlines with the correct values): The maximum value is: ___ This is element number ___ in the list of numbers c. Repeat Exercise 8b, but have your program locate the minimum of the data entered.arrow_forward
- (Practice) a. Write a declaration to store the string "This is a test" in an array named strtest. Include the declaration in a program to display the message using the following loop: for( i=0;iNUMDISPLAY;i++)coutstrtest[i]; NUMDISPLAY is a named constant for the number 14. b. Modify the for statement in Exercise 5a to display only the array characters t, e, s, and t. c. Include the array declaration written in Exercise 5a in a program that uses a cout statement to display characters in the array. For example, the statement cout << strtest; causes the string stored in the strtest array to be displayed. Using this statement requires having the end-of-string marker, \0, as the last character in the array. d. Repeat Exercise 5a, using a while loop. (Hint: Stop the loop when the \0 escape sequence is detected. The expression while (strtest[i]!=0)canbeused.)arrow_forward(Data processing) Write an array declaration statement that stores the following values in an array named volts: 16.24, 18.98, 23.75, 16.29, 19.54, 14.22, 11.13, and 15.39. Include these statements in a program that displays the values in the array.arrow_forward(File creation) Write a C++ program that creates an array containing the integer numbers 60, 40, 80, 90, 120, 150, 130, 160, 170, and 200. Your program should then write the data in the array to a text file. (Alternatively, you can create the file with a text editor.)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
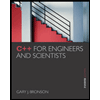
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
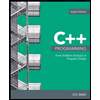
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
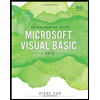
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
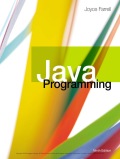
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
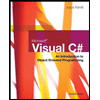
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,