Problem D. Durdle Game Using the function from the previous problem, write a function durdle_game(target) which takes in as an argument a single string that will be the target to guess, and lets the user attempt to guess the target word. Each time the user makes a guess, the function will print out the result of the previous function to tell the user how close they are. If the user guesses correctly, then the game is over. Have the function return the number of guesses that it took the user to get the correct answer. Examples (text in bold is returned, text in red is user input.): >>> durdle_game('trick') Welcome to Durdle! Enter a guess:touch GBBGB Enter a guess:trash GGBBB Enter a guess:truck GGBGG Enter a guess:trick GGGGG Congratulations, you got it in 4 guesses! 4 >>> durdle_game('stoop') Welcome to Durdle! Enter a guess:chart BBBBY Enter a guess:tones YYBBY Enter a guess:togas YYBBY Enter a guess:tofus YYBBY Enter a guess:stock GGGBB Enter a guess:stops GGGYY Enter a guess:stoop GGGGG Congratulations, you got it in 7 guesses! 7
Problem D. Durdle Game
Using the function from the previous problem, write a function durdle_game(target) which takes in as an argument a single string that will be the target to guess, and lets the user attempt to guess the target word. Each time the user makes a guess, the function will print out the result of the previous function to tell the user how close they are. If the user guesses correctly, then the game is over. Have the function return the number of guesses that it took the user to get the correct answer.
Examples (text in bold is returned, text in red is user input.):
>>> durdle_game('trick')
Welcome to Durdle!
Enter a guess:touch
GBBGB
Enter a guess:trash
GGBBB
Enter a guess:truck
GGBGG
Enter a guess:trick
GGGGG
Congratulations, you got it in 4 guesses!
4
>>> durdle_game('stoop')
Welcome to Durdle!
Enter a guess:chart
BBBBY
Enter a guess:tones
YYBBY
Enter a guess:togas
YYBBY
Enter a guess:tofus
YYBBY
Enter a guess:stock
GGGBB
Enter a guess:stops
GGGYY
Enter a guess:stoop
GGGGG
Congratulations, you got it in 7 guesses!
7

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

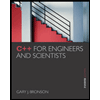
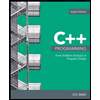
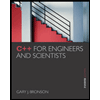
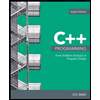