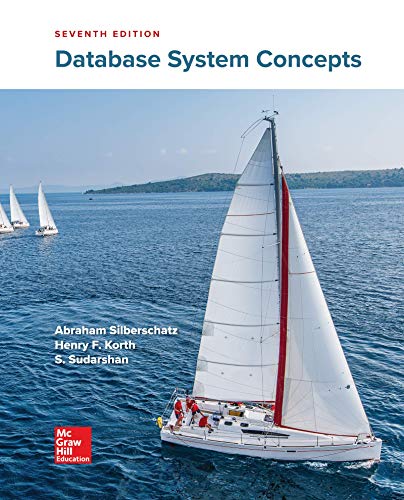
Concept explainers
Program C++
Consider the following class and member function implementation. Derive a class from GradedActivity named Presentation which has two member variable ints, content and delivery which determine a Presentation’s score. Implement the following member functions for Presentation:
- a default constructor which sets both member variables and the score to zero
- a void member function named set which takes two int parameters c and d, sets content to c and delivery to d, and sets the score to c+d. This function may assume that 0 <= c+d <=100, you don't have to check it.
Additionally, provide a simple main() function which creates an object of class Presentation, uses set() to set the object’s member data with some example values, and calls getLetterGrade() to print out the letter grade.
class GradedActivity { private: int score; public: GradedActivity() { score = 0.0; } GradedActivity(int s) { score = s; } void setScore(int s) { score = s; } int getScore() { return score; } char getLetterGrade(); }; |
char GradedActivity::getLetterGrade() { char letterGrade; if (score > 89) letterGrade = 'A'; else if (score > 79) letterGrade = 'B'; else if (score > 69) letterGrade = 'C'; else if (score > 59) letterGrade = 'D'; else letterGrade = 'F'; return letterGrade; } |

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- a. Python does not directly enforce public and private member access and so will not generate an error if you fail to respect the convention. O b. Python supports the concept of public and private through convention - by adding an underscore '_' at the beginning of the member function or variable. C. Just like other languages that support Object Oriented Programming, such as C++ and Java, Python strictly enforces the concept of public and private class members and will generate an error when it runs if you try to access a private member from outside of the class. O d. Python does not support the concepts of public and private members of a class.arrow_forwardUsing C++ and Visual Studios Using your own creativity, make a set of class templates that have these features: For this class template, put everything in one place--do not declare the member functions and have separate definition of the member functions elsewhere. Keep them in one place. Include a private variable. Include a constructor that loads the private variable when constructed. Include a destructor that clears the private variable to zero. Include set and get functions to set and get the private variable.arrow_forwardUsing C++ and Visual Studios Using your own creativity, make a set of class templates that have these features: For this class template, put everything in one place--do not declare the member functions and have separate definition of the member functions elsewhere. Keep them in one place. Include a private variable. Include a constructor that loads the private variable when constructed. Include a destructor that clears the private variable to zero. Include set and get functions to set and get the private variable.arrow_forward
- write the definition of a class statistics containing: three data members x, y, and z of type integer. a member function called setValues that accepts three parameters and assigns them to x, y, and z the function returns no value. a member function called getSum that accepts no parameters and returns the sum of the member variables x, y, and z. a member function called getAvg that accepts no parameters and returns the average of the member variables x, y, and z (the average should be returnsed as a double value. to do this you can use a convert function or just divide by 3.0) a member function called getMin that accepts no parameters and returns the smallest member variable. a member function called getMax that accepts no parameters and returns the largest member variable.arrow_forward6. Write a program to create a class Triangle with three member integer variables side1, side2, and side3. Write the parameterized constructor for the class and write a member function findArea that displays the area of the triangle in the output. In C++arrow_forwardQ2: Write a c++ program that implements the following UML diagram including functions and constructors' definitions. In addition to the user program code that declares object for each class and show how the derived class object uses the base class members. OOPCourse Student ID: int Grades[5]: int + setgrades(int arr[], int ): void + setID(int): int + setage(int): double + print():void + age:int + OOPstudent() OOPCourse OOPLAB OOPLab Reports[4]: int + setmarks(int arr[), int ): void + print():void + OOPLab()arrow_forward
- C++ Programmingarrow_forwardHelp me with C++ please: Rewrite the definitions of the function setDate and the constructor so that the values for the month, day, and year are checked before storing the date into the member variables. Add a member function, isLeapYear, to check whether a year is a leap year. Moreover, write a test program to test your class. Input should be format month day year with each separated by a space. Output should resemble the following: Date #: month-day-year An example of the program is shown below: Date 1: 3-15-2008 this is a leap year There are 3 tabs: dateType.h, dateTypelmp.cpp, main.cpp dateType.h: #ifndef date_H #define date_H class dateType { public: void setDate(int month, int day, int year); //Function to set the date. //The member variables dMonth, dDay, and dYear are set //according to the parameters //Postcondition: dMonth = month; dDay = day; // dYear = year int getDay() const; //Function to return the day.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
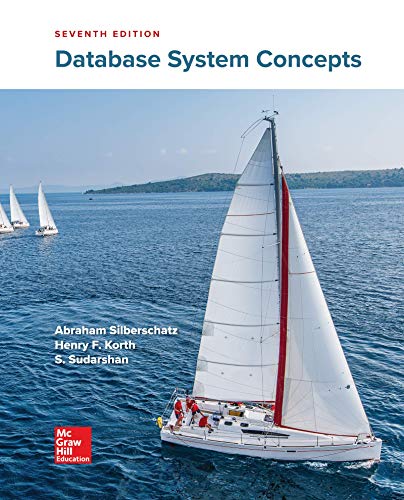
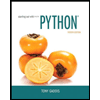
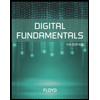
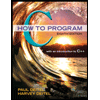
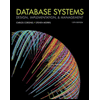
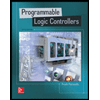