PROGRAM DESCRIPTION: In this assignment, you will write a Python program to process an input file. Download the file minor5.tsv. Each row of this file contains a data record separated by a single tab. The fields are: state, street address, city, zip code. Your need to write a python program that allows users to search for entries using the city or zip code. (a) Write a python function called load_records that given a filename as input, opens the file and reads in the data. Each data record should be represented as a tuple of strings. The function should return two objects: A dictionary mapping zip codes to lists of such tuples and a dictionary mapping cities to sets of zip codes. (b) Write a python program that first reads in the data file once (using the function from part (a)), and then asks the user repeatedly to enter a zip code or a city name (in a while loop until the user types “quit”). For each request, the program prints all data records for this city or zip code. If city names are ambiguous (duplicated city names), all entries should be printed. If no records can be found, you need to print according information such as : No records found in this town. or No records found in this zip code. (c) The format for printed out information should be: Street address Town, state, zipcode minor5.tsv Arkansas 705 E. Union Ave Wynne 72396 California Main Street Redding 96099 California Calabasas & Mullholland Drive Tarzana 91356 Colorado Buffalo Street Dillon 80435 Colorado 802 W. Drake Rd Fort Collins 80526 New Mexico 6th & University Las Vegas 98765 New Mexico corner of bookout and central avenue tularosa 88352 New York 112th Madison Avenue NY 10029 New York W. 57th St. & Ninth Ave at Balsley Park NY 10037 SAMPLE OUTPUT: hz0099@cse02:~/3600/python$ python minor5.py Enter input:10037 W. 57th St. & Ninth Ave at Balsley Park NY, New York, 10037 Enter input:88352 corner of bookout and central avenue tularosa, New Mexico, 88352 Enter input:Wynne 705 E. Union Ave Wynne, Arkansas, 72396 Enter input:Fort Collins 802 W. Drake Rd Fort Collins, Colorado, 80526 Enter input:denton No records found in this town. Enter input:76207 No records found in this zip code. Enter input:NY W. 57th St. & Ninth Ave at Balsley Park NY, New York, 10037 112th Madison Avenue NY, New York, 10029 Enter input:quit hz0099@cse02:~/3600/python$
In this assignment, you will write a Python program to process an input file.
Download the file minor5.tsv. Each row of this file contains a data record separated by a single
tab. The fields are: state, street address, city, zip code.
Your need to write a python program that allows users to search for entries using the city
or zip code.
(a) Write a python function called load_records that given a filename as input, opens the file
and reads in the data. Each data record should be represented as a tuple of strings. The
function should return two objects: A dictionary mapping zip codes to lists of such tuples
and a dictionary mapping cities to sets of zip codes.
(b) Write a python program that first reads in the data file once (using the function from part
(a)), and then asks the user repeatedly to enter a zip code or a city name (in a while loop
until the user types “quit”). For each request, the program prints all data records for this
city or zip code. If city names are ambiguous (duplicated city names), all entries should be
printed. If no records can be found, you need to print according information such as :
No records found in this town.
or
No records found in this zip code.
(c) The format for printed out information should be:
Street address
Town, state, zipcode
minor5.tsv
Arkansas |
705 E. Union Ave |
Wynne |
72396 |
California |
Main Street |
Redding |
96099 |
California |
Calabasas & Mullholland Drive |
Tarzana |
91356 |
Colorado |
Buffalo Street |
Dillon |
80435 |
Colorado |
802 W. Drake Rd |
Fort Collins |
80526 |
New Mexico |
6th & University |
Las Vegas |
98765 |
New Mexico |
corner of bookout and central avenue |
tularosa |
88352 |
New York |
112th Madison Avenue |
NY |
10029 |
New York |
W. 57th St. & Ninth Ave at Balsley Park |
NY |
10037 |
SAMPLE OUTPUT:
hz0099@cse02:~/3600/python$ python minor5.py
Enter input:10037
W. 57th St. & Ninth Ave at Balsley Park
NY, New York, 10037
Enter input:88352
corner of bookout and central avenue
tularosa, New Mexico, 88352
Enter input:Wynne
705 E. Union Ave
Wynne, Arkansas, 72396
Enter input:Fort Collins
802 W. Drake Rd
Fort Collins, Colorado, 80526
Enter input:denton
No records found in this town.
Enter input:76207
No records found in this zip code.
Enter input:NY
W. 57th St. & Ninth Ave at Balsley Park
NY, New York, 10037
112th Madison Avenue
NY, New York, 10029
Enter input:quit
hz0099@cse02:~/3600/python$

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

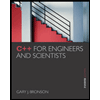
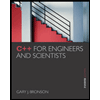