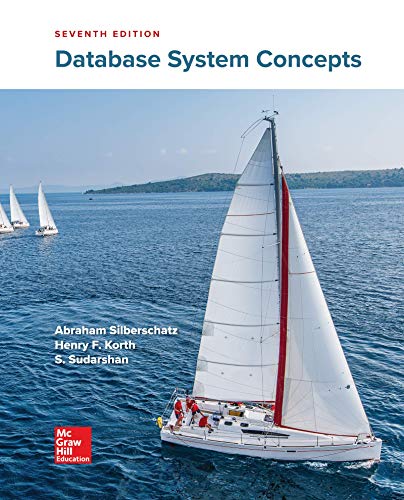
Pseudo-random numbers
Randomly generating numbers is a crucial subroutine of many
The linear congruential method is a simple method for generating pseudo-random numbers. Let m be a positive integer and a be an integer 2 < a <m, and c be an integer 0 ≤ c<m. A linear congruential method uses the following recurrence relation to define a sequence of pseudo-random numbers:
In+1=a+c mod m
(a) Use the linear congruence method with a = 8, c=5, and m = 14, to compute the first 15 pseudo-random numbers when co= 1. That is, compute zo,X1, X 14-
(b) From part (a) we should notice that, with m = 14, the sequence does not contain all 14 numbers in the set Z14. In particular because the sequence is periodic. Using m = 8, determine the value of a which does give all 8 possible numbers before repeating. For your choice of a, which choices of c give all 8 possible numbers? For each a, c pair which give all 8 possible numbers, give the terms of the sequence To...., 7 with 16 = 1
(c) When c = 0, a linear congruence generator is called a purely multiplicative generator. Consider the generator defined by:
Fn+1=65539 mod 231
Show that, modulo 231, this purely multiplicative generator is equivalent to the recurrence relation:
Hint: 65539 216 +3 =

Step by stepSolved in 4 steps

- Blackout Math is a math puzzle in which you are given an incorrect arithmetic equation. The goal of the puzzle is to remove two of the digits and/or operators in the equation so that the resulting equation is correct. For example, given the equation 6 - 5 = 15 ^ 4/2 we can remove the digit 5 and the / operator from the right-hand side in order to obtain the correct equality 6 - 5 = 1 ^ 42. Both sides of the equation now equal to 1. Observe how removing an operator between two numbers (4 and 2) causes the digits of the numbers to be concatenated (42). Here is a more complicated example: 288 / 24 x 6 = 18 x 13 x 8 We can remove digits and operators from either side of the equals sign (either both from one side, or one on each side). In this case, we can remove the 2 from the number 24 on the left-hand side and the 1 from the number 13 on the right-hand side to obtain the correct equality 288 / 4 x 6 = 18 x 3 x 8 Both sides of the equation now equal to 432. Here is another puzzle for you…arrow_forwardjava problem: 1.predict future precipitation pattern for one month: oneMonthGenerator 2.find the number of wet or dry days in a given month’s forecast: numberOfWetDryDays 3.find the longest wet or dry spell in a given month’s forecast: lengthOfLongestWetDrySpellarrow_forwardThe Polish mathematician Wacław Sierpiński described the pattern in 1915, but it has appeared in Italian art since the 13th century. Though the Sierpinski triangle looks complex, it can be generated with a short recursive function. Your main task is to write a recursive function sierpinski() that plots a Sierpinski triangle of order n to standard drawing. Think recursively: sierpinski() should draw one filled equilateral triangle (pointed downwards) and then call itself recursively three times (with an appropriate stopping condition). It should draw 1 filled triangle for n = 1; 4 filled triangles for n = 2; and 13 filled triangles for n = 3; and so forth. API specification. When writing your program, exercise modular design by organizing it into four functions, as specified in the following API: public class Sierpinski { // Height of an equilateral triangle whose sides are of the specified length. public static double height(double length) // Draws a filled equilateral…arrow_forward
- The use of computers in education is referred to as computer-assisted instruction (CAI). One problem that develops in CAI environments is student fatigue. This problem can be eliminated by varying the computer's dialogue to hold the student's attention. For each correct answer and each incorrect an- swer. The set of responses for correct answers is as follows: Very good! Excellent! Nice work! Keep up the good work! The set of responses for incorrect answers is as follows: No. Please try again. Wrong. Try once more. Don't give up!No. Keep trying. Use random number generation to choose a number from 1 to 4 that will be used to select an appropriate response to each answer. Use a switch statement to issue the responses. Please write the code in Javascript HTML5arrow_forwardAny problem that can be solved recursively can also be solved with a .arrow_forwardWrite Algorithm for Synchronized simulation for Guess a Number.in: set of human participants H; set of computer participants Cout: winner wconstant: minimum number nmin; maximum number nmaxlocal: guessed number garrow_forward
- Write a recursive implementation of Euclid’s algorithm for finding the greatest common divisor (GCD) of two integers. Descriptions of this algorithm are available in algebra books and on the Web. Write a test program that calls your GCD procedure five times, using the following pairs of integers: (5,20), (24,18), (11,7), (432,226), (26,13). After each procedure call, display the GCD.arrow_forwardJava:arrow_forwardConsider a grid of size (x, y) where x and y are positive integers. The following figure shows an example grid of size (2,3) and two points p1 and p2 in the grid. Assume that x and y of p2 is greater than x and y of p1. p2(2,2) ectangular Snip p1(0,0) Write a recursive method named countPaths that returns the number of distinct shortest paths from p1 to p2. The method takes 4 ints as the parameters – i.e., x and y values of the two points: x1, y1, x2, and y2. Note that you do not need to print the paths. Count only. To be shortest, a path will only consist of only upward and rightward movements where each movement increases x- or y-coordinate value by 1. In the figure above, |(right, up, right, up) or (right, right, up, up) are both shortest paths from p1 to p2. For example, countPaths (0, 0, 2, 2) returns 6 countPaths (0, 0, 1, 1) returns 2 countPaths (2, 1, 2, 2) returns 1arrow_forward
- Write the algorithm for the problem that works in constant space and time complexity. Input: N OUTPUT: 1 if Tom wins, 0 if Jerry wins.arrow_forwardAssume that you were given N cents (N is an integer) and you were asked to break up the N cents into coins consisting of 1 cent, 2 cents and 5 cents. Write a dynamic programming-based recursive algorithm, which returns the smallest (optimal) number of coins needed to solve this problem. For example, if your algorithm is called A, and N = 13, then A(N) = A(13) returns 4, since 5+5+2+1 = 13 used the smallest (optimal) number of coins. In contrast, 5+5+1+1+1 is not an optimal answer. Draw the recursion tree for the algorithm where N = 7. Derive the complexity bound of the algorithm. Do not need to prove the complexity bound formally, just derive it by analyzing each component in your algorithm.arrow_forwardA recursive algorithm has been developed as shown below: Input: List A = (a₁,..., an) such that N > 1 Output: value x foo(A) if length(A) == 1 return a₁ A' = (a₁,..., aN-1) q = foo(A') if (q> an) else return q return an State what problem this algorithm solves and write down the two recurrence equations that characterise this recursive algorithm.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
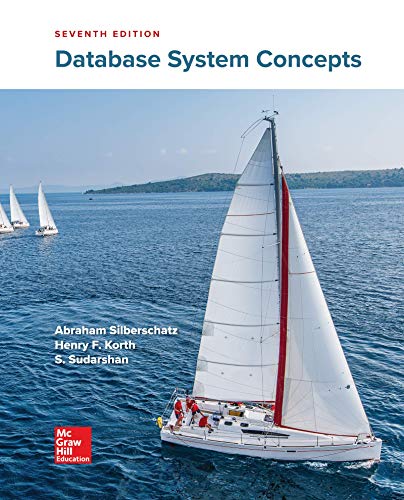
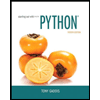
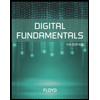
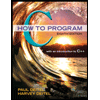
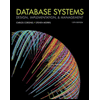
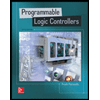