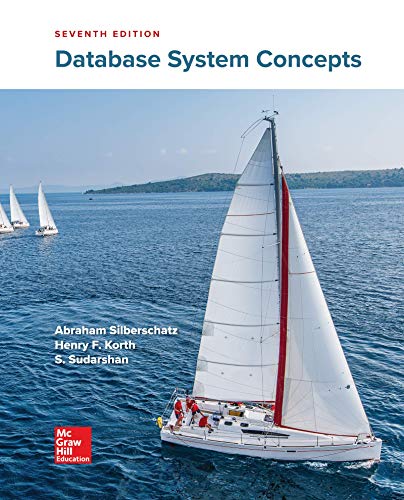
PYTHON ONLY AND PLEASE COMMENT CODE
1.
A) write a For loop that iterates through a list of products (any number of products) that are sold in a store and prints them. [examples: fan, fridge, freezer, washer, etc.].
b) copy the code from a) and use the same loop to add more products(any)to the list. You must use list methods to add.
c) Copy the code from c), and search for a specific product (any), if the product is found the loop is terminated. Make sure to use else in the for loop.
d) Create an empty dictionary, then add 4 entries (the key is a word, the value is word’s frequency in a book). [example: {‘street’: 23}].
e) Create a for loop to access the entries from d) and print the keys and values.
f) Copy the code from e) and search for a specific key in the dictionary and prints its value. The program prints a message “ the {key} is not found”, if the key does not exist.

Step by stepSolved in 2 steps with 1 images

- static List<String> findWinners(String pattern, int minLength, boolean even, Stream<String> stream) This function will receive a stream of strings and it will return a sorted list of strings that meet all of the following criteria: They must contain the pattern The strings length must be equal to or greater than the min length number The strings length must be an even or odd number This Function must take no longer 10000 milliseconds to return. pattern - A pattern that all the strings in the list returned must containminLength - The length that all the strings in the list returned must be equal to or greater thaneven - True if even false if odd. Denotes a condition that all the strings in the list returned must have a length that is even or odd.stream - A stream of strings to be filteredreturn - A list of strings that meets the criteria outlined above sorted by their length from small to largearrow_forwardWrite a Do While loop that counts from 12-24. This loop will produce a list box that will contain those values. Submit code only.arrow_forward6. sum_highest_five This function takes a list of numbers, finds the five largest numbers in the list, and returns their sum. If the list of numbers contains fewer than five elements, raise a ValueError with whatever error message you like. Sample calls should look like: >>> sum_highest_five([10, 10, 10, 10, 10, 5, -17, 2, 3.1])50>>> sum_highest_five([5])Traceback (most recent call last): File "/usr/lib/python3.8/idlelib/run.py", line 559, in runcode exec(code, self.locals) File "<pyshell#44>", line 1, in <module> File "/homework/final.py", line 102, in sum_highest_five raise ValueError("need at least 5 numbers")ValueError: need at least 5 numbersarrow_forward
- Lab Goal : This lab was designed to teach you more about list processing and algorithms.Lab Description : Write a program that will search a list to find the first odd number. If an odd number is found, then find the first even number following the odd number. Return the distance between the first odd number and the LAST even number. Return -1 if no odd numbers are found or there are no even numbers following an odd numberarrow_forward# cannot contain syntax error def replace(keyv:int,newv:int,S:set)->set:"Find a number keyv from a given set S" # note S is a set"As soon as found, replace the number keyv with a number newv""return the resultant set with the other numbers unchanged""Input keyv to be found, if found replace with newv else no change to the set""S contains a set of int, output the resultant set" if __name__ == "__main__":# testing cod goes inside this ifarrow_forwardPOEM List[POEM_LINE] PRONUNCIATION_DICT Dict[str, PHONEMES] Do not add statements that call print, input, or open, or use an import statement. Do not use any break or continue statements.arrow_forward
- python help, please show all steps and include comments Write a function largerLst() that takes a list of numbers (i.e., int or float values) as a parameter and returns a new list constructed out of it. The list returned has all the values in the parameter list that are larger than the element immediately to their right, that is, larger than their right neighbor. If the parameter list is empty or there are no values larger than their right neighbor, then the function returns an empty list. Note that the last element in the list will never be included in the list returned since it does not have a right neighbor. The function should not change the parameter list in any way. Note that a correct solution to this problem must use an indexed loop That is, one that makes a call to the range() function to generate indices into the list which are used to solve the problem (Review lecture notes 8-1). A solution that does not use an indexed loop will not be accepted. Note in particular that you…arrow_forwardLottery number generator: Write a program that generates aseven-digit lottery number. The program should have a loopto generate seven random numbers, each in the range 0through 9 and assign each number to a list element.2. Write another loop to display the contents of the list.3. Tip: You will need to create/initialize your list before you canassign numbers to it.4. Use program 7-1 sales_list as an example. You will start witha seven-digit lottery number that contains all zeros. Then inyour loop, you will assign a random number instead ofgetting the data from the user.Turn in your program to the practice assignment link in coursecontent.arrow_forwardAssume that the variable data refers to the list [5, 3, 7]. Write the expressions that perform the following tasks: a. Replace the value at position o in data with that value's negation. b. Add the value 10 to the end of data. c. Insert the value 22 at position 2 in data. d. Remove the value at position 1 in data. e. Add the values in the list newData to the end of data. f. Locate the index of the value 7 in data, safely. g. Sort the values in data.arrow_forward
- M4Lab1ii.py - C:\Users\speed\Downloads\M4Lab1ii.py (3.10.7) File Edit Format Run Options Window Help #Calculator Program. #M4Lablii.py for M4 Assignment 1 # C. Calongne, 01/19/19 #Seven steps to complete in this lab: # Define a list to store the choices for +, -, *, / # Define 3 functions with local variables #Define three elif statements that call the functions for -, *, and / # Local variables are visible in the functions #Pass the input values into them. # Continue calculating until the user presses "n" print ("Welcome to your Calculator program") # The add function adds two numbers. def add(x, y): return x + y #local scope; only the add () function sees x and y #write the other three functions for subtract, multiply and divide #Step 1: Write a subtract function that subtracts two numbers. #Step 2: Write a multiply function that multiplies two numbers #Step 3: Write a divide function that divides two numbers # Start calculating #Step 4: define a list called choice with + - * / in it…arrow_forward1c) Average sentence length We will create a function ( avg_sentence_len ) to calculate the average sentence length across a piece of text. This function should take text as an input parameter. Within this function: 1. sentences : Use the split() string method to split the input text at every '.'. This will split the text into a list of sentences. Store this in the variable sentences . To keep things simple, we will consider every "." as a sentence separator. (This decision could lead to misleading answers. For example, "Hello Dr. Jacob." is actually a single sentence, but our function will consider this 2 separate sentences). 2. words : Use the split() method to split the input text into a list of separate words, storing this in words . Again, to limit complexity, we will assume that all words are separated by a single space (" "). (So, while "I am going.to see you later" actually has 7 words, since there is no space after the ".", so we will assume the this to contain 6 separate…arrow_forwardPythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
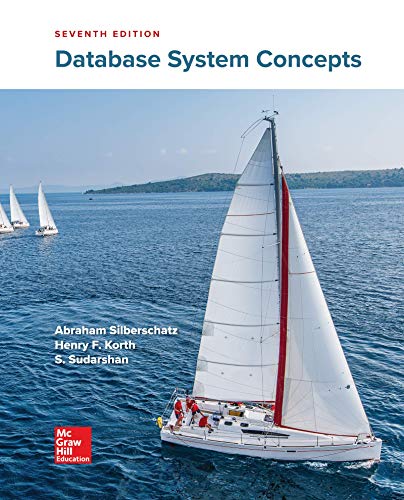
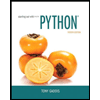
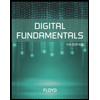
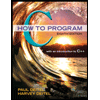
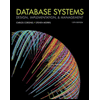
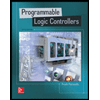