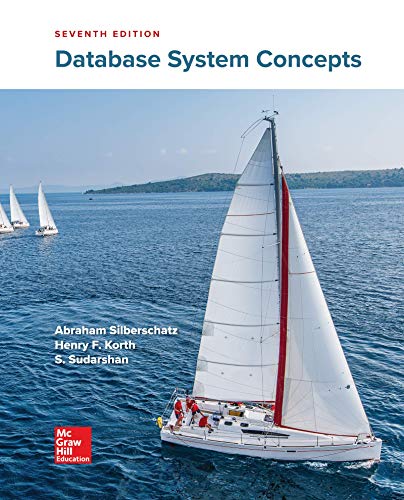
Python’s list method sort includes the keyword argument reverse, whose default
value is False. The programmer can override this value to sort a list in descending
order. Modify the selectionSort function so that it allows
the programmer to supply this additional argument to redirect the sort.
selectionSort function:
def selectionSort(lyst, profiler):
i = 0
while i < len(lyst) - 1:
minIndex = i
j = i + 1
while j < len(lyst):
profiler.comparison() # Count
if lyst[j] < lyst[minIndex]:
minIndex = j
j += 1
if minIndex != i:
swap(lyst, minIndex, i, profiler)
i += 1
def swap(lyst, i, j, profiler):
"""Exchanges the elements at positions i and j."""
profiler.exchange() # Count
temp = lyst[i]
lyst[i] = lyst[j]
lyst[j] = temp
Use this template in Python to modify the function above:
def selection_sort(input_list, reverse):
sorted_list = []
#TODO: Your work here
# Return sorted_list
return sorted_list
if __name__ == "__main__":
my_list = [1, 2, 3, 4, 5]
print(selection_sort(my_list, True)) # Correct Output: [5, 4, 3, 2, 1]

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Can u explain the thought process behind the code ?
Can u explain the thought process behind the code ?
- Implement a function void printAll(void) to print all the items of the list.arrow_forwardWrite a function called remove_odd that takes a list of numbers that have both even and odd numbers mixed.# Function should remove all the odd numbers and return a compact list which only contains the even numbers. Example1: Function Call:remove_odd ([21, 33, 44, 66, 11, 1, 88, 45, 10, 9])Output:[44, 66, 88, 10]arrow_forwardDuplicate Set This function will receive a list of elements with duplicate elements. It should add all of the duplicate elements to a set and return the set containing the duplicate elements. A duplicate element is an element found more than one time in the specified list. The order of the set does not matter. Signature: public static HashSet<Object> duplicateSet(ArrayList<Object> list) Example: INPUT: [2, 4, 5, 3, 3, 5] OUTPUT: {5, 3}arrow_forward
- Pythong help!arrow_forwardQUESTION 4 in phython language Write a program that creates a list of N elements (Hint: use append() function), swaps the first and the last element and prints the list on the screen. N should be a dynamic number entered by the user. Sample output: How many numbers would you like to enter?: 5 Enter a number: 34 Enter a number: 67 Enter a number: 23 Enter a number: 90 Enter a number: 12 The list is: [12, 67,23, 90, 34]arrow_forwardWrite a python program that takes two lists, merges thetwo lists, sorts the resulting list, and then finds the median of theelements in the two lists. You cannot use python build-in sort() function #todo#You can use math functions like math.floor to help the calculationimport math #todo#You can use math functions like math.floor to help the calculation import math def task7(list_in_1, list_in_2): # YOUR CODE HERE return median please use these variables no print function pleasearrow_forward
- Write a function called find_duplicates which accepts one list as a parameter. This function needs to sort through the list passed to it and find values that are duplicated in the list. The duplicated values should be compiled into another list which the function will return. No matter how many times a word is duplicated, it should only be added once to the duplicates list. NB: Only write the function. Do not call it. For example: Test Result random_words = ("remember","snakes","nappy","rough","dusty","judicious","brainy","shop","light","straw","quickest", "adventurous","yielding","grandiose","replace","fat","wipe","happy","brainy","shop","light","straw", "quickest","adventurous","yielding","grandiose","motion","gaudy","precede","medical","park","flowers", "noiseless","blade","hanging","whistle","event","slip") print(find_duplicates(sorted(random_words))) ['adventurous', 'brainy', 'grandiose', 'light', 'quickest', 'shop', 'straw', 'yielding']…arrow_forwardComplete the reverse_list() function that returns a new string list containing all contents in the parameter but in reverse order. Ex: If the input list is: ['a', 'b', 'c'] then the returned list will be: ['c', 'b', 'a'] Note: Use a for loop. DO NOT use reverse() or reversed(). This is my code so far: def reverse_list(letters): for x in (letters): letters.sort(reverse=True) return lettersif __name__ == '__main__': ch = ['a', 'b', 'c'] print(reverse_list(ch)) # Should print ['c', 'b', 'a'] When submitting I get 4/10 and it says: Test input list ['a', 'b', 'c'] returns ['c', 'b', 'a'] Your output reverse_list() function reversed array incorrectly: ['c', 'b', 'a'] reverse_list() function should have returned: ['a', 'b', 'c'] Test input list ['a', 'b', 'c', 'd'], first element of returned list is ['d'] Your output First character of returned array should be a instead of d Test empty input array TypeError: object of type 'NoneType' has no len()arrow_forwardNo explicit loops must be used. In C# Suppose variable nums contains a list of integers.List«Integer> nums = Arrays.asList(1, 1, 2, 3, 4, 1, 3, 2, 4, 3, 3, 1);var nums = new List<int> { 1, 1, 2, 3, 4, 1, 3, 2, 4, 3, 3, 1 }; // C#Using Java Stream API *or* LINQ, write a short code that prints the following.For each section indicate the platform (whether the solution is given in Java orC# ILINQ).A) Print all numbers.B) Print the sum() of the numbers.C) count how many times number '1' appears in the list.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
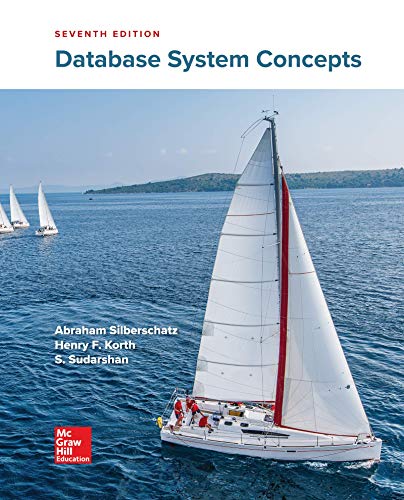
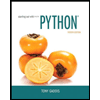
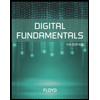
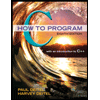
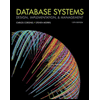
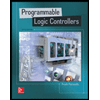