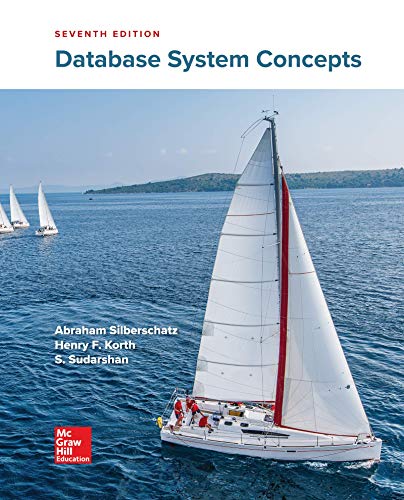
Concept explainers
Question 1.
Implement a computer
G, using Dijkstra’s algorithm.
Instructions
Given a picture of an arbitrary undirected, simple, weighted graph, G, with a maximum of 10 vertices:
a. Capture the graph, G=(V, E), into your program;
b. Nominate an initial vertex and a goal vertex;
c. Calculate the shortest path between those two vertices;
d. Display the shortest route between the two vertices;
e. Display the distance or cost of the route.
The following discussion points should be included:
Description of graph capture method
Implementation choices
Screenshot of program output
Relevant example or use in practice
Reflection
Hints
Make use of the graph test case provided to verify that your implementation is operating correctly
See the extra FAQ document for additional clarification on common questions
See section 6.3.1 Skiena 2nd edition and section 8.31 Skiena 3rd edition
There are numerous ways to solve the different parts of the problem, but in principle, the following
functions are required:
o Define a struct to house the graph information
o Input vertices (V) and edges (E) of graph G into the program
o Input initial- and goal- node/vertex
o Logic to visit each node and keep track of which nodes were already visited
o Logic to perform Dijkstra at each visited node
o Output route and cost

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- Write a program that outputs the nodes of a graph in a depth-first traversal. Write a program that outputs the nodes of a graph in a breadth-first traversal. Write a program to test the breadth-first topological ordering algorithmarrow_forwardAlice, a friend of Mr. Bernard’s, wants to compose a song. She asks Mr. Bernard for help. Although Mr. Barnard knows nothing about music, he finds that the sequence of notes in a numbered musical notation can be expressed as an FSM with input the alphabet {1, 2, 3, 4, 5, 6, 7} (“do”, “re”, “mi”, “fa”, “so”, “la”, “ti”). Alice wants to compose a song that contains the pattern “15” an even number of times. Please draw an FSM that only accepts a sequence of notes that satisfies the above pattern. Note that you should provide a detailed description of the FSMs in your design to show how it works. Specifically, you should describe the meaning of each state.arrow_forwardGiven a weighted graph class and a PQInt class, please complete the implementation of the Dijkstras algorithm according to the instructions in the screenshot provided. Be done in python 3.10 or later, please class WeightedGraph(Graph) : """Weighted graph represented with adjacency lists.""" def __init__(self, v=10, edges=[], weights=[]) : """Initializes a weighted graph with a specified number of vertexes. Keyword arguments: v - number of vertexes edges - any iterable of ordered pairs indicating the edges weights - list of weights, same length as edges list """ super().__init__(v) for i, (u, v) in enumerate(edges): self.add_edge(u, v, weights[i]) def add_edge(self, a, b, w=1) : """Adds an edge to the graph. Keyword arguments: a - first end point b - second end point """ self._adj[a].add(b, w) self._adj[b].add(a, w) def…arrow_forward
- b3arrow_forwardUsing C++. Assume your development team is tasked with writing a pathfinder ( solution finder ) or the childhood game of 8 sliding tiles game. Your task is to determine whether or not an initial board configuration is or is not solvable. Your algorithm should do this by counting inversions. If the number of inversions is even, then the board is solvable. If the number of inversions is odd, then the board is not solvable (there is NO sequence of moves that will transition from the initial board to the goal board).arrow_forwardJava programming.arrow_forward
- IN HASKELL PROGRAMMING LANGUAGE PLEASE In case you do not know it: the game is played on a 3x3 grid that is initially empty. Two players are playing, by alternatingly making moves. A move by a player places their token (an X for player 1, an O for player 2) into a cell that was empty. We are using algebraic notations for indexing the positions in the board, with A,B,C indexing the columns and 1,2,3 the rows. Specifically, these coordinates would be used in the implementation for moves made by a human player. If the X X O Figure 1: Sample board position same token appears 3 times in any of the three columns, three rows or two main diagonals the game is over and that player wins. If the grid is filled without that happening the game is a draw. For the depicted board, we have Xs in positions C3 and A2, and an O in position B1. It would be O’s turn to make a move; a legal move would be C2, but it is not a good move, because X can force a win by responding A1. O cannot force a win, but…arrow_forwardin java replit code pleasearrow_forwardMust be new solution and run on GNU Common Lisp! Using Lisp, write a program that solves the Missionaries and Cannibals problem that uses a DFS( depth first search). It should use (mac start end). Start is the current state (which can be (3 3 l) and End is the goal state (which can be (0 0 r). This should output the sequences of moves needed to reach the end state from the start state. This should print nil if there is no solution. For example, the call should be something like this! Call: (mac '(3 3 l) '(0 0 r)) Output: ((3 3 l) (2 2 r) (3 2 l) (3 0 r) (3 1 l) (1 1 r) (2 2 l) (0 2 r) (0 3 l) (0 1 r) (1 1 l) (0 0 r))arrow_forward
- Write a program in C that, given two points on a two-dimensional graph, outputs a message (string) if the line that connects them is horizontal or vertical, or if the slope is positive or negative.What would you modify or adjust in your code if we move from a 2D cartesian system to a 3D dimensional cartesian system?You need to take into consideration if the system is 3D and therefore you need to ask the user to insert X, Y , and Z.arrow_forwardIn Python the only import that may be used is Numpy Implement a function called page_rank which will take as input a numpy array M, which will represent the transition matrix of a directed graph, and a positive integer n. The output will be a numpy array which gives the page ranks of each vertex in the graph represented by M. You will iterate the update process n times.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
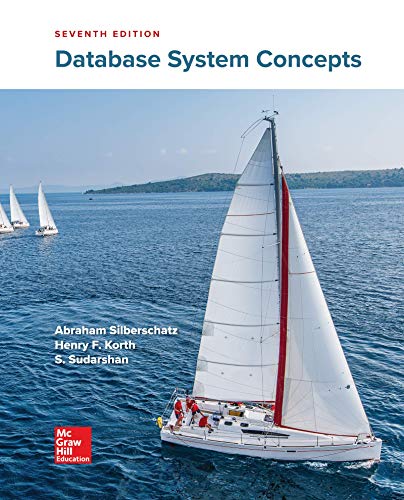
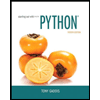
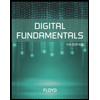
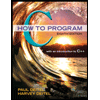
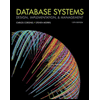
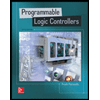