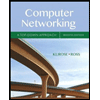
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
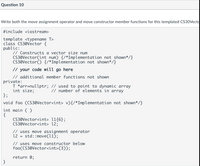
Transcribed Image Text:### Question 10
**Task:** Write both the move assignment operator and move constructor member functions for this templated `CS30Vector` class.
```cpp
#include <iostream>
template <typename T>
class CS30Vector {
public:
// Constructs a vector size num
CS30Vector(int num) {/*Implementation not shown*/}
CS30Vector() {/*Implementation not shown*/}
// your code will go here
// additional member functions not shown
private:
T *arr=nullptr; // used to point to dynamic array
int size; // number of elements in array
};
void foo (CS30Vector<int> v){/*Implementation not shown*/}
int main ( ) {
CS30Vector<int> l1{6};
CS30Vector<int> l2;
// uses move assignment operator
l2 = std::move(l1);
// uses move constructor below
foo(CS30Vector<int>(3));
return 0;
}
```
### Explanation
This code provides a partially implemented template class `CS30Vector`, which represents a simple dynamic array (vector). Two constructors are declared but not implemented:
1. **Parameterized Constructor:** `CS30Vector(int num)` which initializes a vector of size `num`.
2. **Default Constructor:** `CS30Vector()` which serves as a default initializing constructor.
### Private Members
- **arr:** A pointer of type `T` (template type), intended to point to a dynamic array.
- **size:** An integer to store the number of elements in the array.
### Tasks to Implement
You are required to implement:
- **Move Assignment Operator:** Responsible for allowing an object to be moved rather than copied, useful to optimize performance when working with temporary objects.
- **Move Constructor:** An essential function to transfer resource ownership from one object to another (also optimizing performance), especially for temporary and rvalue objects.
### Usage in `main`
- **Move Assignment Operator** is demonstrated by:
```cpp
l2 = std::move(l1);
```
This line shows how the resources from `l1` are moved to `l2`.
- **Move Constructor** is utilized in:
```cpp
foo(CS30Vector<int>(3));
```
This line implicitly creates a temporary object of `CS30Vector<int>` which is then moved into the function `foo`.
This task
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- PART ONE In C# create a Contestant class with the following characteristics: The Contestant class contains public static arrays that hold talent codes and descriptions. Recall that the talent categories are Singing, Dancing, Musical instrument, and Other. Name these fields talentCodes and talentStrings respectively. The class contains an auto-implemented property Name that holds a contestant’s name. The class contains fields for a talent code (talentCode) and description (talent). The set accessor for the code assigns a code only if it is valid. Otherwise, it assigns I for Invalid. The talent description is a read-only property that is assigned a value when the code is set. Modify the GreenvilleRevenue.cs program (See Program Below) so that it uses the Contestant class and performs the following tasks: The program prompts the user for the number of contestants in this year’s competition; the number must be between 0 and 30. The program continues to prompt the user until a valid value…arrow_forwardIf a base class has a non-virtual member function named print, and a pointer variable of that class is pointing to an object of a derived class, then the code ptr->print(); callsarrow_forwardUsing C++ Define the class called Student. The Student class has the following: Part 1) Private data members: name(string), age(int), units(int). The units represent the number of quarter units student is enrolled in. Define a default constructor as well as a constructor with parameters for the class Student. The class must have get and set functions for all private data members. The set function for the data member units must throw “out_of_range” exception if the number of units is not between 1 and 15. Include a function called tuition (double feePerUnit) that computes and returns the cost of registering for the number of units (in the private data member). The function receives the cost per unit as a parameter. Overload the operator (<<) to display student name and age. Test the class Student by writing a main program in which a Student object is created and displayed. Call the function tution(), you may pass any value as feePerUnit parameter to this function and display the…arrow_forward
- Javascript Question: Part 1 Design a Pet object. A Pet is created with a language argument string and a stomach represented by an array. The Pet should support functions: eat() and speak(). Use the prototype property to define your object's interface. eat(food) will store the passed argument into its stomach and return what it has eaten thus far. speak(sentence) will simply return the passed in phrase. function Pet(language) {// create stomach// set language}// takes a food_item STRING and returns everything eaten so far ARRAYPet.prototype.eat = function(food_item) {// ... complete }// takes in a sentence STRING and returns the passed in sentence STRING with no changePet.prototype.speak = function(sentence) {// ... complete} You may also use the newer class notation. Part 2 Create an object, Dog, that implements the Pet interface and takes the same argument language. Dogs eat the same way all pets do. Dogs speak differently. Dogs replace each word in a sentence with its only known…arrow_forwardbriefly explain this code #include<iostream>#include<string.h> using namespace std; class bank_account{int acno;char nm[100], acctype[100];float bal;public:bank_account(int acc_no, char *name, char *acc_type, float balance){acno=acc_no;strcpy(nm, name);strcpy(acctype, acc_type);bal=balance;}void deposit();void withdraw();void display();};void bank_account::deposit(){int damt1;cout<<"\n Enter Deposit Amount = ";cin>>damt1;bal+=damt1;}void bank_account::withdraw(){int wamt1;cout<<"\n Enter Withdraw Amount = ";cin>>wamt1;if(wamt1>bal)cout<<"\n Cannot Withdraw Amount";bal-=wamt1;}void bank_account::display(){cout<<"\n Name : "<<nm;cout<<"\n Account Type : "<<acctype;cout<<"\n Balance : "<<bal;}int main(){int acc_no;char name[100], acc_type[100];float balance;cout<<"\n Name : ";cin>>name;cout<<"\n Account Type : ";cin>>acc_type;cout<<"\n Balance : ";cin>>balance;bank_account…arrow_forwardQ2: Write a c++ program that implements the following UML diagram including functions and constructors' definitions. In addition to the user program code that declares object for each class and show how the derived class object uses the base class members. OOPCourse Student ID: int Grades[5]: int + setgrades(int arr[], int ): void + setID(int): int + setage(int): double + print():void + age:int + OOPstudent() OOPCourse OOPLAB OOPLab Reports[4]: int + setmarks(int arr[), int ): void + print():void + OOPLab()arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
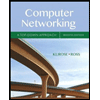
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
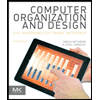
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
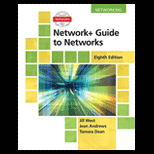
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
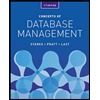
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
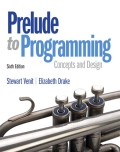
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
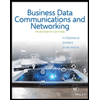
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY