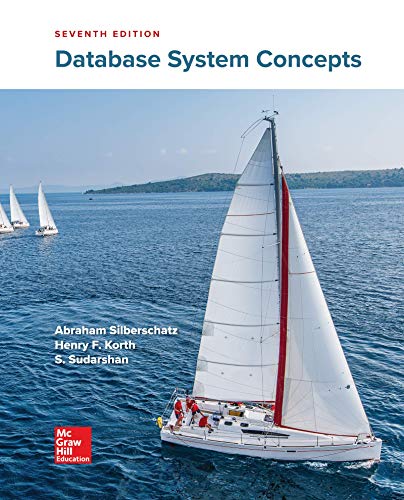
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Revise the following Course class implementation in the following c++ code
- When adding a new student to the course, if the array capacity is exceeded, increase the array size by creating a new larger array and copying the contents of the current array to it.
- Implement the dropStudent function.
- Add a new function named clear() that removes all students from the course.
- Implement the destructor and copy constructor to perform a deep copy in the class.
Write a test program that creates a course, adds three students, removes one, and displays the students in the course.
![#include <iostream>
#include "Course.h"
using namespace std;
Course::Course(const string& courseName, int capacity)
{
F
numberOfStudents = 8:
this->courseName = courseName;
this->capacity = capacity;
students = new string[capacity];
Course::~Course()
delete [] students;
string Course::getCourseName() const
{
}
void Course::addStudent (const string& name)
{
return courseName;
students [numberOfStudents]
numberOfStudents++;
Semnal
name:
}
void Course::dropStudent (const string& name)
X
// Left as an exercise
C
string* Course::getStudents() const
K
return students;
int Course::getNumberOfStudents() const
K
return numberOfStudents;
Course.cp](https://content.bartleby.com/qna-images/question/09af1c01-6e60-4588-9390-2a0cbfc62f20/d8e13eff-ce01-4c9e-a0ec-60b7a97c2f9d/tx4ata.jpeg)
Transcribed Image Text:#include <iostream>
#include "Course.h"
using namespace std;
Course::Course(const string& courseName, int capacity)
{
F
numberOfStudents = 8:
this->courseName = courseName;
this->capacity = capacity;
students = new string[capacity];
Course::~Course()
delete [] students;
string Course::getCourseName() const
{
}
void Course::addStudent (const string& name)
{
return courseName;
students [numberOfStudents]
numberOfStudents++;
Semnal
name:
}
void Course::dropStudent (const string& name)
X
// Left as an exercise
C
string* Course::getStudents() const
K
return students;
int Course::getNumberOfStudents() const
K
return numberOfStudents;
Course.cp
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- 10 Build the Sudoku Reviewer ( the testing program). The specification is below. Please note: you DO NOT have to build a Sudoku solver. To test you just need to build a class that generates a 9x9 2D array with the values you want to test. You can assume the value types are valid. (integers from 1-9). 1-The main purpose is to make sure the Sudoku solver actually solved it correctly! 2- You are also confirming the solver solves puzzle in no longer than a minute 3- Solver (not the reviewer) requires 7 INDICES (ELEMENTS) filled in the input array at least. 4- The reviewer receives both the original puzzle and the solution. The Reviewer needs to confirm the solution is not only a valid SudoKu solution but also the solution to the original puzzle. 5- Solver will return a 2d array filled with -1s if input array has an error/bad input (exception). Reviewer will check for this case.arrow_forwardPlease use easy logic with proper indentations and comments for understanding!. Coding should be in C++. 3. Define a class which stores up to 10 integer values in an array. The class should also define the following 4 public methods: setNumber – accepts an integer value to store in the array. The value is stored in the next available element of the array (first call to the method stores the value in element 0 with the second call storing the value in element 1.) The method returns true if there is room in the array and the integer was successfully stored. Returns false otherwise. clear – removes all values from the array so that the array can be reused. displayNumbers – displays the values currently stored in the array. getStats – determines the largest, smallest, and average of the values currently stored in the array. These values are returned to the caller via reference parameters. All methods should produce correct results regardless of the order in which…arrow_forwardJava - Gift Exchange *** Please include UML Diagram and notes in code Minimum requirements are: At least 1 loop An Array or ArrayList At least 3 Java classes Use methods I am trying to make it so that the program will: Prompt for the number of people included in exchange - If not even it will state that there has to be an even number and ask for the number of people included again (loops). Prompt to enter a participant's first name Prompt for the participant's age Print out the random matching of participants so that everyone gets a gift and everyone gives a gift. Please include UML Diagramarrow_forward
- In C# Use a one-dimensional array to solve the following problem. Write a class called ScoreFinder. This class receives a single dimensional integer array representing passer ratings for NFL players as an argument for its constructor. The test class will provides this argument. The test class then calls upon a method from the ScoreFinder class to start the process of finding a specific rating, which will be provided by the user. The method will iterate through the array and find any matching values. For all the matches that are found, the method will note the index of the cell along with its value. At completion, the method will print a list of all the indices and the associated scores along with a count of all the matching values.arrow_forwardcreate using c++ One problem with dynamic arrays is that once the array is created using the new operator the size cannot be changed. For example, you might want to add or delete entries from the array similar to the behavior of a vector . This project asks you to create a class called DynamicStringArray that includes member functions that allow it to emulate the behavior of a vector of strings. The class should have the following A private member variable called dynamicArray that references a dynamic array of type string. A private member variable called size that holds the number of entries in the array. A default constructor that sets the dynamic array to NULL and sets size to 0. A function that returns size . A function named addEntry that takes a string as input. The function should create a new dynamic array one element larger than dynamicArray , copy all elements from dynamicArray into the new array, add the new string onto the end of the new array, increment size, delete the…arrow_forwardQuèstion 17 when an individual array element of an array of type int is passed to a method, it is passed by reference. True Falsearrow_forward
- array of Payroll ObjectsDesign a PayRoll class that has data members for an employee’shourly pay rate and number of hours worked. Write a program withan array of seven PayRoll objects. The program should read thenumber of hours each employee worked and their hourly pay ratefrom a file and call class functions to store this information in theappropriate objects. It should then call a class function, once foreach object, to return the employee’s gross pay, so this informationcan be displayed.arrow_forwardJava:arrow_forwardPlease add an execution chart for this code like the example below. I have provided the code and the example execution chart. : Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,// and input and return value if any//open file//fill…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
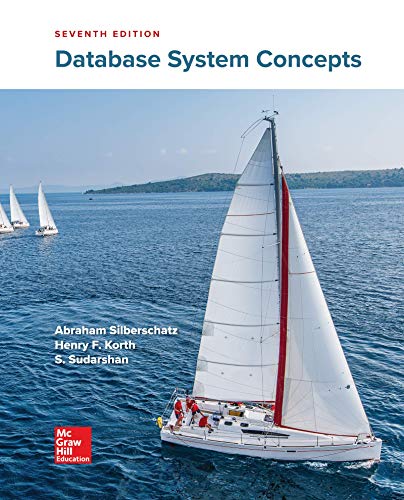
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
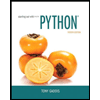
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
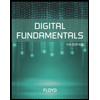
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
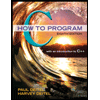
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
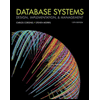
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
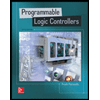
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education