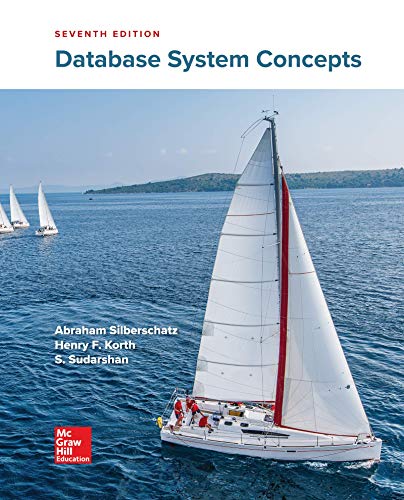
PLEASE WRITE IN JAVA USING ECLIPSE IED
Objective:
Write a
Requirements:
- Functionality. (20pts)
- No Syntax Errors. (20pts*)
- *Code that cannot be compiled due to syntax errors is nonfunctional code and will receive no points for this entire section.
- User Inputs the size and values of the array. (5pts)
- The program must ask the user for the size of the array. This value must be strictly greater than zero, otherwise the program must print “Invalid Array Size” and immediately terminate. Otherwise, the program must create an array of integers of that size.
- The program must ask the user to input each value, and then must store those values into the array.
- All must apply for full credit.
- Find the Minimum Even Value. (5pts)
- The program must then determine the minimum even value found in the array.
- The program must not assume that the first value in the array is either even or odd but must instead find the first even value – if it exists.
- Assume the value 0 is even.
- All must apply for full credit.
- Find the Minimum Odd Value. (5pts)
- The program must then determine the minimum odd value found in the array.
- The program must not assume that the first value in the array is either even or odd but must instead find the first odd value – if it exists.
- All must apply for full credit.
- Print the Results. (5pts)
- The program must clearly print the minimum even or odd value found in the array.
- In the even there were either no even or odd values (or both), then the program must print “No Even Values” or “No Odd Values” respectively. HINT: It may be a good idea to keep track of how many even or odd values are found in the array.
- All must apply for full credit.
- Coding Style. (2pts)
- Readable Code
- Meaningful identifiers for data and methods.
- Proper indentation that clearly identifies statements within the body of a class, a method, a branching statement, a loop statement, etc.
- All the above must apply for full credit.
- Comments. (3pts)
- Your name in the file. (1pts)
- At least 5 meaningful comments in addition to your name. These must describe the function of the code it is near. (2pts)
Solution Tests:
- Does the solution compile?
- Does the solution have your name in the comments and 5 meaningful comments that describe the code?
- If the array has the values {9,1,8,2,7,3,6,4,5} does the program print out:
Min Even: 2
Min Odd: 1
- If the array has the values {1,2,3,4,5,6,-7,-8} does the program print out:
Min Even: -8
Min Odd: -7
- If the array has the values {1,1,1,1,1} does the program print out:
Min Even: No Even Values
Min Odd: 1
- If the array has the values {2,-4,6,8,10} does the program print out:
Min Even: -4
Min Odd: No Odd Values

Java Program:
import java.util.Scanner;
import java.lang.*;
public class Main
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
int n; //Size of the array
System.out.print("Enter size of the array: ");
n = sc.nextInt();
if(n <= 0)
{
System.out.print("Invalid Array Size");
System.exit(0);//Terminate
}
int arr[] = new int[n]; //create an array of integers of that size.
System.out.print("Enter array values:\n");
for(int i=0;i<n;i++)
arr[i] = sc.nextInt();
//Find the Minimum Even Value.
int minEven = 9999; //In case no minimum even value is found.
int minOdd = 9999; //In case no minimum odd value is found.
for(int i=0;i<n;i++)
{
if(arr[i]%2 == 0)
{
if(minEven > arr[i])
minEven = arr[i];
}
else
{
if(minOdd > arr[i])
minOdd = arr[i];
}
}
if(minEven == 9999)
System.out.print("\nNo Even Values");
else
System.out.print("\nMinimum Even Value = " + minEven);
if(minOdd == 9999)
System.out.print("\nNo Odd Values");
else
System.out.print("\nMinimum Odd Value = " + minOdd);
}
}
Output:
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Please complete this program in C++ Please enusre that there is plenty of comments. PLENTY even if unessessary. Please include screenshots of actual code, screenshots of output, and code in the browser so I can copy and paste. Thank you.arrow_forwardWrite a Java to do the following USING ARRAYS (NO HARD CODING) MUST USE METHODS Arrays And Functions (Methods) hint write and test one module at the time 1) Input 15 integers (input validation , all numbers must be between -20 and 50 inclusive ) ,create a method/function. 2 methods/functions one for inputand one for input validation 2) Find and display all negative number in the array, create a method/function. 3) Display every other element in the array, create a method/function 4) Find the Sum of all positive numbers in the Array, create a method/function 5) a method/function to display the original array 6) include a menu using the switch statement to enable the user to select one of the the options (2,3,4). 7) include a loop to restart the menuarrow_forwardIn JAVA PROGRAMMING please read details on pictures thank you. (You will need to read in the student data first and then read in the scores) You will open each file and read the information into their respective classes/arrays For each student, you will calculate their lowest score, their highest score, their average, and their grade. For each quiz, you will calculate the lowest score, the highest score, and the average You will create and print an Honor Role. The Honor Role comprises students who received an ‘A’ grade. REPORT WILL LOOK LIKE THIS LastName FirstName StudentID Score 1 Score 2 Score 3 Score 4 Score 5 High Low Average Grade High…arrow_forward
- This is warning you, don't use any AI platform to generate answer.arrow_forwardConsider the following program specification: Write a program that will prompt the user for 10 integer values and stores them in an array. The values entered by the user only be between 0 and 100 and the program should validate these appropriately. The program should then: Display the average of the values Display the highest value in the array Display the lowest value in the array Discuss how the values should be validated. What control structures could be used? Using eclipsearrow_forward10 Build the Sudoku Reviewer ( the testing program). The specification is below. Please note: you DO NOT have to build a Sudoku solver. To test you just need to build a class that generates a 9x9 2D array with the values you want to test. You can assume the value types are valid. (integers from 1-9). 1-The main purpose is to make sure the Sudoku solver actually solved it correctly! 2- You are also confirming the solver solves puzzle in no longer than a minute 3- Solver (not the reviewer) requires 7 INDICES (ELEMENTS) filled in the input array at least. 4- The reviewer receives both the original puzzle and the solution. The Reviewer needs to confirm the solution is not only a valid SudoKu solution but also the solution to the original puzzle. 5- Solver will return a 2d array filled with -1s if input array has an error/bad input (exception). Reviewer will check for this case.arrow_forward
- Arrays of objects. Java programming: How to create and work with an array of objects in Java I wanted to know that supppose that I have 100 employees who are paid on an hourly basis and I need to keep track of their arrival and departure times, and I have also created the class Clock to implement the time of day in a program. I also know that I will use two arraays; one called arrivalTime and the other array called departure time. Each of these arrays will have 100 elements and each element will be a reference variable of Clock type.. For example Clock[] arrival = new Clock[100]. My qeustion is how do I create the objects arrival and departure. Then how could I use the methods f the class clokc. Thank youarrow_forwardCODE IN JAVA design a program that grades arithmetic quizzes as follows: (Use the below startup code for Quizzes.java and provide code as indicated by the comments) 1. Ask the user how many questions are in the quiz. 2. Use a for loop to load the array. Ask the user to enter the key (that is, the correct answers). There should be one answer for each question in the quiz, and each answer should be an integer. They can be entered on a single line, e.g., 34, 7, 13, 100, 8 might be the key for a 5-question quiz. You will need to store the key in an array called "key". 3. Ask the user to enter the student's answers for the quiz to be graded. There needs to be one answer for each question. Note that each answer can simply be compared to the key as it is entered. If the answer is correct, add 1 to a correct answer counter. need this in a separate for loop from the loop used to load the array. 4. When the user has entered all of the answers to be graded, print the number correct…arrow_forwardExercise #1: Cumulative sum: Write a Java program that prompts the user to enter an integer number (n) that indicates the number of elements of an array (the maximum value for n is 100). Then the user enters n double numbers. The program creates another array of cumulative sums of the first array and outputs it. One decimal place should be used to output the cumulative array. Sample inputs/outputs: Enter the array size: 4 Enter the array elements: 5.0 6.5 7.3 10.2 Cumulative Array: 5.0 11.5 18.8 29.0 Enter the array size: 101 The maximum size is 100! Enter the array size: 5 Enter the array elements: 2.34 5.61 4.67 0.897 Cumulative Array: 2.3 8.0 12.6 19.6 20.4arrow_forward
- java languageCreate a new main fileCreate an array of size 3. The array must be of class mammal.Create an object of each class.(feline, domestic)Assign values to each of the created objects.Assign each of the objects to each of the positions in the array.Using a loop, it displays each of the positions in the array.arrow_forwardin c++ write a code to return the value of fake coin, the fake coin is The different element of the value in the array ،and need for way solution using 1) brute force approaches 2) divide and conquer 3) decrease and conquer 4) transform and conquer and write the time of each one and what the best time of them. in c++ write a code to return the value of fake coin, the fake coin is The different element of the value in the array and be less value (minimum) , need four way solution using 1) brute force approaches 2)divide and conquer 3)decrease and conquer 4) transform and conquer and write the time of each one and what the best time of them.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
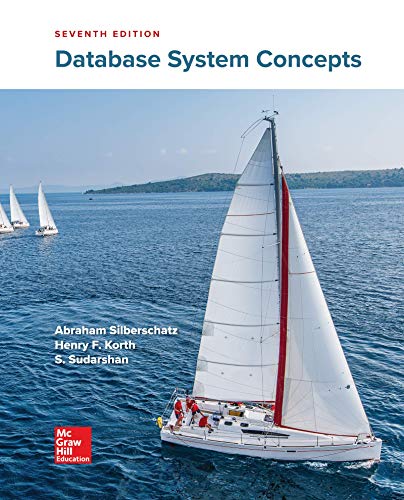
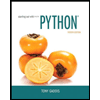
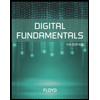
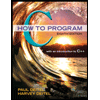
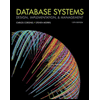
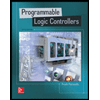