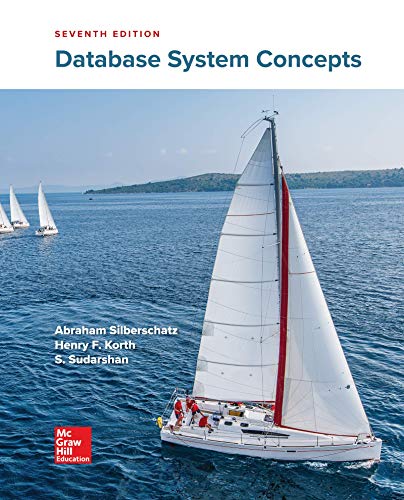
Concept explainers
Select all true statements
import java.io.File;
import java.util.Scanner;
public class DirectorySize {
public static void main(String[] args) {
// Prompt the user to enter a directory or a file
System.out.print("Enter a directory or a file: ");
Scanner input = new Scanner(System.in);
String directory = input.nextLine();
// Display the size
System.out.println(getSize(new File(directory)) + " bytes");
}
public static long getSize(File file) {
long size = 0; // Store the total size of all files
if (file.isDirectory()) {
File[] files = file.listFiles();
for (int i = 0; files != null && i < files.length; i++) {
size += getSize(files[i]); // Recursive call
}
}
else { // Base case
size += file.length();
}
return size;
}
}
a. |
The length() method returns the size of a file. |
|
b. |
The listFiles() method returns an array of File objects and excludes each directory. |
|
c. |
The length() method returns a value of -1 if the file is a directory. |
|
d. |
The listFiles() method returns an array of File objects under a directory. |

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- you must create the data files with a text tool, such as Notepad exampleThree In the while loop, this program reads fileLoop.txt until all items are read package hasnext; import java.util.Scanner; import java.io.*; // public class Hasnext { public static void main(String[] args) throws IOException { File loopfile = new File("fileLoop.txt"); Scanner getAll = new Scanner( loopfile ); // connect a Scanner to the file int num = 0, square = 0; while(getAll.hasNextInt()) { num = getAll.nextInt(); square = num * num ; System.out.println("The square of " + num + " is " + square); } getAll.close(); } }arrow_forwardWrite a program that reads a file one character at a time and prints out how many characters are uppercase letters, lowercase letters, digits, white space, and something else. Characters.java 1 import java.io.File; 2 import java.io.FileNotFoundException; 3 import java.io.PrintWriter; 4 import java.util.Scanner; 5 6 public class Characters 7 { 8 public static void main(String[] args) throws FileNotFoundException { Scanner console = new Scanner(System.in); System.out.print("Input file: "); String inputFileName int uppercase int lowercase 9 10 11 12 console.next( ); 0; 0; 0; = 0; 0; 13 %3D 14 %3D int digits int whitespace int other 15 16 17 %D 18 while (. { 19 .) 20 21 if (. else if (. else if (. else if (. else other++; 22 .) { uppercase++; } .) { lowercase++; } .) { digits++; } .) { whitespace++; } 23 24 25 26 27 } 28 System.out.println("Uppercase: System.out.println("Lowercase: System.out.println("Digits: System.out.println("Whitespace: System.out.println("0ther: } + uppercase); +…arrow_forwardcreateDatabaseOfProfiles(String filename) This method creates and populates the database array with the profiles from the input file (profile.txt) filename parameter. Each profile includes a persons' name and two DNA sequences. 1. Reads the number of profiles from the input file AND create the database array to hold that number profiles. 2. Reads the profiles from the input file. 3. For each person in the file 1. creates a Profile object with the information from file (see input file format below). 2. insert the newly created profile into the next position in the database array (instance variable).arrow_forward
- help me rewrite the code without using bufferReader pleasearrow_forwardpublic static void connectToInputFile() {Scanner inputStream = null;String inputFileName = getFileName("Enter name of input file: ");try{inputStream = new Scanner(new File("SortedA.txt"));}catch(FileNotFoundException e){System.out.println("File " + inputFileName + " not found. ");}catch(IOException e){System.out.println("Error opening input file: " + inputFileName);}}private static String getFileName(String prompt){String fileName = null;System.out.println(prompt);Scanner keyboard = new Scanner (System.in);fileName = keyboard.next();return fileName;}public static void connectToOutputFile(){String outputFileName = getFileName("Enter name of output file: ");try{outputStream = new ObjectOutputStream(new FileOutputStream("SortedArray.csv"));}catch(IOException e){System.out.println("Error opening output file" + outputFileName);System.out.println(e.getMessage());}}public static void closeFiles(){try{inputStream.close();outputStream.close();}catch(IOException e){System.out.println("Error…arrow_forwardCan information be read from a text file using a predetermined kind of object?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
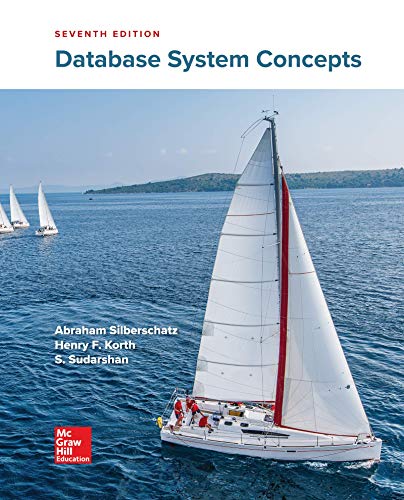
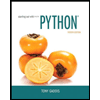
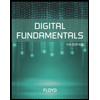
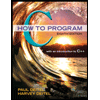
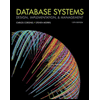
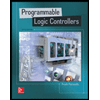