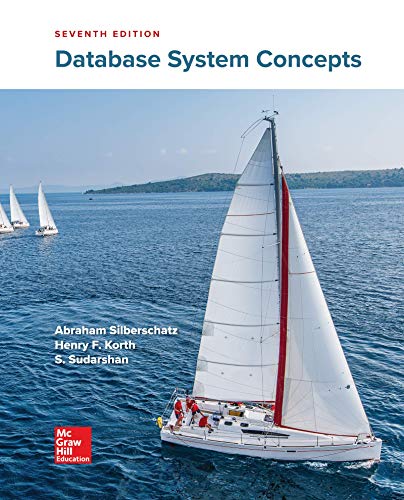
Concept explainers
Sentinel Value- Python Coding
This program runs to figure out if numbers the user enters are odd or even.
The While loop within the try block is supposed to repeat until the user types in "1". However, the while loop does not continue to run, it just exits after 1 run through.
Below is my coding so far:
# Description:Give instructions for the game and a tell them to input a 3 digital number ONLY, use a while loop. determine if numbers are odd or even.
#give instructions using print statements
print("In this Odd and Even game, you will enter a three digit number (eg.145) and we will tell you if it is odd or even!")
print("Type '1' to quit")
while True:
#try block
try:
#enter a three-digit number
num=int(input('Enter a three-digit number only (type "1" to quit): '))
#check if number of digits in number is 3 or not
oddList=[]
evenList=[]
while num !=1:
if len(str(num))==3:
#check if number is even
if num%2==0:
print("Your number,%s, is even"%(num))
evenList.append(int(num))
#if number is not even then print odd
else:
print("Your number,%s, is odd"%(num))
oddList.append(int(num))
#first number odd or even
#make number a list
listNum=list(str(num))
firstNum=listNum[0]
#if and else statements for odd or even
if int(firstNum)%2==0:
print("%s is even."%(firstNum))
evenList.append(int(firstNum))
else:
print("%s is odd"%(firstNum))
oddList.append(int(firstNum))
secondNum=listNum[1]
if int(secondNum)%2==0:
print("%s is even"%(secondNum))
evenList.append(int(secondNum))
else:
print("%s is odd"%(secondNum))
oddList.append(int(secondNum))
thirdNum=listNum[2]
if int(thirdNum)%2==0:
print("%s is even"%(thirdNum))
evenList.append(int(thirdNum))
else:
print("%s is odd"%(thirdNum))
oddList.append(int(thirdNum))
#sum of all digits
sum=0
#find sum
#make string numbers integers
firstNum=int(firstNum)
secondNum=int(secondNum)
thirdNum=int(thirdNum)
sum=firstNum+secondNum+thirdNum
print("The sum of all individual numbers: %s+%s+%s=%s"%(firstNum,secondNum,thirdNum,sum))
if sum%2==0:
print("%s is an even number"%(sum))
evenList.append(int(sum))
else:
print("%s is an odd number"%(sum))
oddList.append(int(sum))
print("odd numbers:%s"%(oddList))
print("even numbers:%s"%(evenList))
#if length of number is not 3 then raise value error exception
else:
raise ValueError
break
print("your odd numbers: %s"%(oddList))
print("your even numbers: %s"%(evenList))
exit()
#except block
except ValueError:
#print statement if number is not 3 digits
print('Not a three-digit number')

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- #1 Name Use a loop to write your name ten times #7 Namecount Redo #1 but every time you print your name out also print out the value of the loop counter(for example the first three lines might look like this) do this ten times Patti K 0 Patti K 1 Patti K 2arrow_forwardWrite a do...while loop that uses the loop control variable to take on the values 0 through 10.arrow_forwardin phython langage Write a while loop that will print all the numbers between 200 and 300 (200 and 300 included) that are divisible by 3 but not divisible by 6. Your output should include the following numbers: 201 207 213 219 225 231 237 243 249 255 261 267 273 279 285 291 297arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
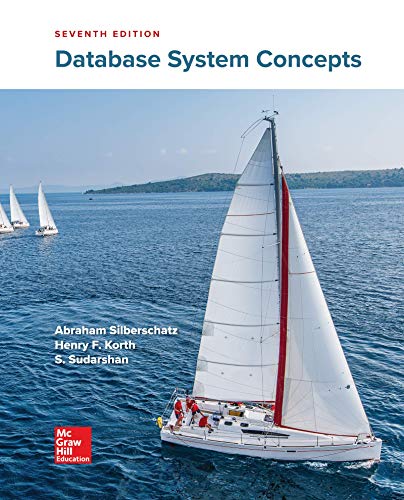
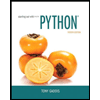
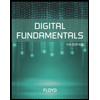
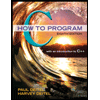
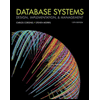
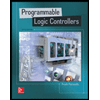