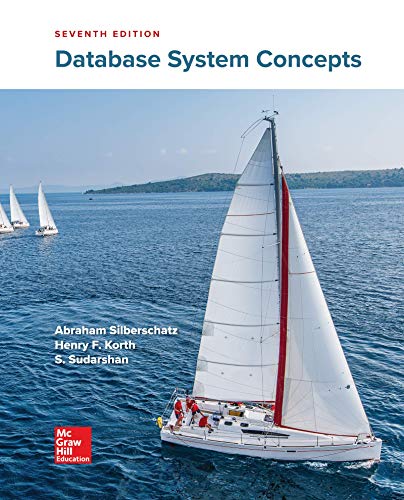
Using arrays, write a C++ program that will let a user input the array size (how many elements). After inputting the the array size, it should ask the user for the elements of the array. After inputting all the elements of the array, It will prompt the user to choose the following options:
1. Get the sum of all the array elements
2. Sort the numbers from largest to smallest
3. Sort the numbers from smallest to largest
4. Get the average of the array elements.
Sample Output:
Enter array Size: 5
Enter number 1: 5
Enter number 2: 4
Enter number 3: 3
Enter number 4: 2
Enter number 5: 1
Please choose from the following options:
1. Get the sum of all the array elements
2. Sort the numbers from largest to smallest
3. Sort the numbers from smallest to largest
4. Get the average of the array elements.
Your Choice: 1
You chose 1.
The sum of the elements is 15.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Do the following lab by one dimensional array(not vector) Write a C++ program that: part a) for 3 students find the average of a student by asking him how many grades do you have. To answer to this question, determine the size of the array(number of grades) and then ask the user to enter grades(the number of the grades is equal to the size of the array) then calculate the average for each student. Hence, you will calculate 3 averages. to calculate the average, use the array accumulator. Also when you get each grade validate it. validation for grade means that you check whether a grade is between 0 and 100. if the grade is negative or a grade is more than 100 then it is not valid and you have to ask the user to enter a grade again. Part b) Find the maximum and minimum grades for each student. Part c) Print the sorted list of the grades for each student. example: if grades user entered are: 60 78 98 23 45 then sorted list is:23 45 60 78 98arrow_forwardC languagearrow_forwardTopic: Array covered in Chapter 7 Do not use any topic not covered in lecture. Write a C++ program to store and process an integer array. The program creates an array (numbers) of integers that can store up to 10 numbers. The program then asks the user to enter up to a maximum of 10 numbers, or enter 999 if there are less than 10 numbers. The program should store the numbers in the array. Then the program goes through the array to display all even (divisible by 2) numbers in the array that are entered by the user. Then the program goes through the array to display all odd (not divisible by 2) numbers in the array that are entered by the user. At the end, the program displays the total sum of all numbers that are entered by the user.arrow_forward
- Variables Primitive Write a java program that asks the user to enter 3 integers. Add the integers and display “The total is “ the result Reference Write a java program that asks the user for their first name and last name. Display “Your full name is “ the first name and last name Arrays One-dimensional Write a java program that creates an array of integers called numbers and contains five elements. Then use a for loop to print them out using println(). Two-dimensional submit your code for the total of rows and total of columns herearrow_forwardProblem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forwardIn C write a grading program as follows.- Ask the user for the number of students and store it in an integer variable.- Create an array of floats with four rows and columns equal to the number of students storedearlier.- Initialize the array to zeros.Create a menu with the following options (use a do-while loop and repeatedly display the menu):A or a to add student info one student at a timeT or t to display class average for homeworkS or s to display class average for quizzesB or b to display class average for examsZ or z to exit program (program repeats until this exit command is entered)arrow_forward
- Exercise #1: Cumulative sum: Write a Java program that prompts the user to enter an integer number (n) that indicates the number of elements of an array (the maximum value for n is 100). Then the user enters n double numbers. The program creates another array of cumulative sums of the first array and outputs it. One decimal place should be used to output the cumulative array. Sample inputs/outputs: Enter the array size: 4 Enter the array elements: 5.0 6.5 7.3 10.2 Cumulative Array: 5.0 11.5 18.8 29.0 Enter the array size: 101 The maximum size is 100! Enter the array size: 5 Enter the array elements: 2.34 5.61 4.67 0.897 Cumulative Array: 2.3 8.0 12.6 19.6 20.4arrow_forwardWrite a program that reads student scores into an array, gets the best score, and then assigns grades based on the following scheme: • Grade is A if score is • Grade is B if score is • Grade is C if score is • Grade is D if score is • Grade is F otherwise. best - 10 best - 20; best - 30; best - 40; The program prompts the user to enter the total number of students, then prompts the user to enter all of the scores, and concludes by displaying the gradesarrow_forwardHello, Stuck on this question. All programming is in Python. 2.) Design a program that generates a 7-digit lottery number. The program should have an INTEGER array with 7 elements. Write a loop that steps through the array, randomly generating a number in the range of 0 through 9 for each element. Then write another loop that displays the contents of the array. All programming is in Python.arrow_forward
- Write a program in C as follows:- Create an array of integers named “toy” that has 120 rows and 4 columns.- The program should repeatedly display the following menu:A or a to add a toy to the bagV or v to calculate and display the total value of the toysW or w to calculate and display the total weight of the toysD or d to delete a toy from the arrayM or m to calculate and display the number of small toysN or n to calculate and display the number of medium toysL or l to calculate and display the number of large toysX or x to start filling a new bagP or p to exit program - Santa’s bag can hold 30 large toys or 60 medium toys or 120 small toys or any combination ofsizes that satisfy this requirement (ex: 29 large + 1 medium + 2 small would be max capacity).Also, the total weight of toys cannot exceed 620 Kgs. All values are entered in centimeters andgrams. (Hint: the return values of the size function should help you in calculating the bagcapacity.)The…arrow_forwardWrite a C code which asks a user how many numbers (up to 100) they want to enter. The program then stores the data in an array and sends it to a function to compute the average of the numbers of the array. The function should then return the average and the program should then print the average.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
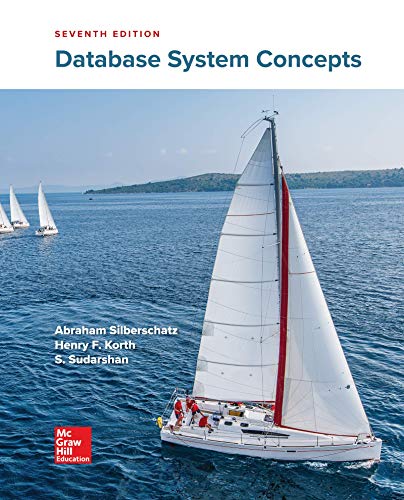
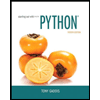
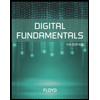
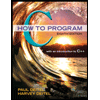
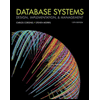
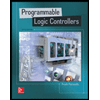