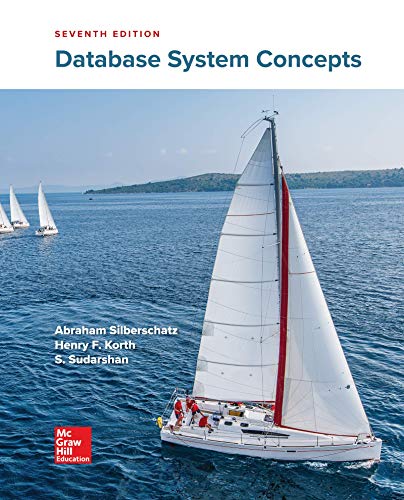
So for my project program in Python I'm suppose to code a BankApp in which the user is suppose to input their username and password to access their balance details and withdraw or deposit money. The program works though there is a small issue. See when testing I notice a problem with the withdraw and deposit function, see if I put a negative value rather than saying "invalid" and looping back to the menu it will do the opposite which I'll provide a screenshot to explain it better. Can you fix and explain how you did it(also label/number the lines you fix/added code)&(UserInfo will also be provided for code to work)-Thank you.
Code
def read_user_information(filename):
usernames = []
passwords = []
balances = []
try:
with open(filename, "r") as file:
lines = file.readlines()
for line in lines[2:]:
data = line.strip().split()
if len(data) == 3:
usernames.append(data[0])
passwords.append(data[1])
balances.append(float(data[2]))
except FileNotFoundError:
print("User information file not found. A new file will be created.")
return usernames, passwords, balances
def write_user_information(filename, usernames, passwords, balances):
with open(filename, "w") as file:
file.write("userName passWord Balance\n")
file.write("========================\n")
for i in range(len(usernames)):
file.write(f"{usernames[i]:<12} {passwords[i]:<12} {balances[i]:<}\n")
def login(usernames, passwords):
username_input = input("Enter username: ")
password_input = input("Enter password: ")
for i, username in enumerate(usernames):
if username == username_input and passwords[i] == password_input:
return i
print("Invalid username or password. Please try again.")
return None
def deposit(balances, user_index):
try:
amount = float(input("Enter the amount to deposit: "))
balances[user_index] += amount
print(f"Deposit successful. New balance: ${balances[user_index]:.2f}")
except ValueError:
print("Invalid amount entered. Deposit failed.")
def withdraw(balances, user_index):
try:
amount = float(input("Enter the amount to withdraw: "))
if amount > balances[user_index]:
print("Insufficient funds. Withdrawal failed.")
else:
balances[user_index] -= amount
print(f"Withdrawal successful. New balance: ${balances[user_index]:.2f}")
except ValueError:
print("Invalid amount entered. Withdrawal failed.")
def show_balance(balances, user_index):
print(f"Current balance: ${balances[user_index]:.2f}")
def add_new_user(usernames, passwords, balances):
new_username = input("Enter new username: ")
new_password = input("Enter new password: ")
try:
new_balance = float(input("Enter initial balance: "))
usernames.append(new_username)
passwords.append(new_password)
balances.append(new_balance)
print(f"New user {new_username} added with balance: ${new_balance:.2f}")
except ValueError:
print("Invalid balance entered. User addition failed.")
def main():
filename = "UserInformation.txt"
usernames, passwords, balances = read_user_information(filename)
user_index = None
while user_index is None:
user_index = login(usernames, passwords)
while True:
print("\nMenu:")
print("D - Deposit Money")
print("W - Withdraw Money")
print("B - Display Balance")
print("C - Change User")
print("A - Add New Client")
print("E - Exit")
choice = input("Enter your choice: ").upper()
if choice == 'D':
deposit(balances, user_index)
elif choice == 'W':
withdraw(balances, user_index)
elif choice == 'B':
show_balance(balances, user_index)
elif choice == 'C':
user_index = None
while user_index is None:
user_index = login(usernames, passwords)
elif choice == 'A':
add_new_user(usernames, passwords, balances)
elif choice == 'E':
write_user_information(filename, usernames, passwords, balances)
print("Exiting program. User information updated.")
break
else:
print("Invalid option. Please try again.")
if __name__ == "__main__":
main()
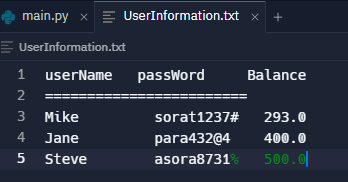
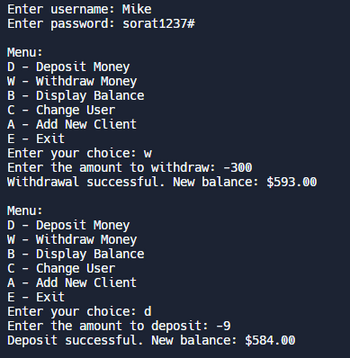

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Give a concrete example of a function, where blackbox testing might give the impression that “everything’s OK,” while whitebox testing might uncover an error. Give another concrete example of a function where the opposite is true. You need to support both examples with test cases.arrow_forward9 from breezypythongui import EasyFrame 10 11 class TemperatureConverter(EasyFrame): 12 ""A termperature conversion program.""" II II II 13 def -_init__(self): "I"Sets up the window and widgets.""" EasyFrame._init_-(self, title = "Temperature Converter") 14 15 II IIII 16 17 18 # Label and field for Celsius self.addLabel(text = "Celsius", 19 20 row = 0, column = 0) 21 self.celsiusField = self.addFloatField(value = 0.0, 22 row = 1, column = Ø, 23 24 precision = 2) 25 # Label and field for Fahrenheit self.addLabel(text = "Fahrenheit", 26 27 28 row = 0, column = 1) 29 self.fahrField = self.addFloatField(value = 32.0, 30 row = 1, 31 column = 1, 32 precision = 2) 33 # Celsius to Fahrenheit button self.addButton(text = ">>>>", 34 35 row = 2, column = 0, command = self.computeFahr) 36 37 38 39 # Fahrenheit to Celsius button 40 self.addButton(text = "<«««", 41 row = 2, column = 1, 42 command = self.computeCelsius) 43 44 # The controller methods def computeFahr(self): "I"Inputs the Celsius…arrow_forwardSo for my project program in Python I'm suppose to code a BankApp in which the user is suppose to input their username and password to access their balance details and withdraw or deposit money. The program works though there is a small issue. See when testing I notice a problem with the withdraw and deposit function, see if I put a negative value rather than saying "invalid" and looping back to the menu it will do the opposite which I'll provide a screenshot to explain it better. Can you fix and explain how you did it(also show a sceenshot on where you fix/added code)&(UserInfo will also be provided for code to work)-Thank you. Code def read_user_information(filename): usernames = [] passwords = [] balances = [] try: with open(filename, "r") as file: lines = file.readlines() for line in lines[2:]: data = line.strip().split() if len(data) == 3: usernames.append(data[0])…arrow_forward
- Write the algorithm in a flow chart. The arithmetic operations are limited to only addition, subtraction, multiplication, and division. You can use chart software such as Lucidchart, powerpoint, or Visio. Please export it to a pdf file (e.g., Q3.pdf) Implement the algorithm in C. Again the arithmetic operations are limited to only addition, subtraction, multiplication (in Q3 not in Q4), and division (in Q4 not in Q3). (e.g., Q3.c) Implement the algorithm for MARIE architecture (e.g., Q3_MARIE.mas) The numbers of instructions in Q3_INSTRS.docx (Word document) Q4. Logarithm (Iterative algorithm) - Use Q2 Get a user input of two positive integer numbers: A and B where A is a base and B is an exponent of A Calculate C= Log base A of B Print C Test case: A=2, B=16 Output should be 4arrow_forwardWrite a Python program that asks the user to input 2 numbers. add the 2 numbers together print a statement that shows the 2 numbers that were entered and their sum. For instance, if I input 2 and 4 the output should say, "The sum of 2 and 4 is 6". It is probably easiest to use the repl.it web site....you can write your code and run it there - BUT - you have to turn in the code to Canvas. Just copy and paste the code into the assignmentarrow_forwardIt is a python program. Make sure it works and run in IDLE 3.10. Please show the code screenshot and output screenshot.arrow_forward
- I need help figuring out what i need to include in my code for the Simulation Statistics part?arrow_forwardYOU SHOULD NOT USE LOOPS (for, while,….) OR CONDITIONALS (if..else,….),For this question, you should use Math function for doing the “power” and “π”. When printingthe volume and the area values, DON’T ENTER THEM MANUALLY. Only print 2 numbers after the decimal points.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
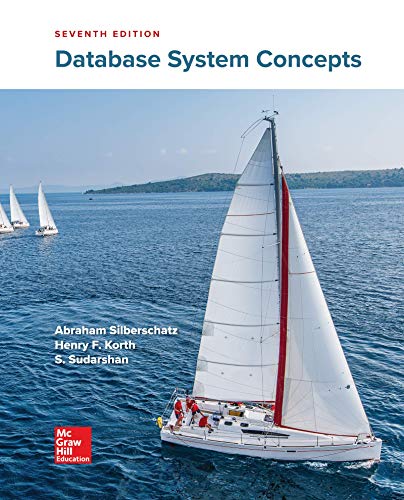
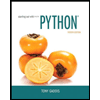
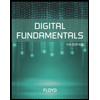
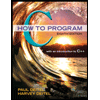
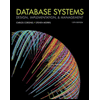
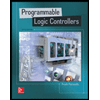