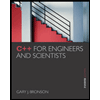
String inquiredItem and integer numData are read from input. Then, numData alphabetically sorted strings are read from input and each string is appended to a
- If inquiredItem is alphabetically before middleValue (the element at index middleIndex of the vector), output "Search lower half" and recursively call FindMiddle() to find inquiredItem in the lower half of the range.
- Otherwise, output "Search upper half" and recursively call FindMiddle() to find inquiredItem in the upper half of the range.
End each output with a newline.
Click here for example Ex: If the input is:
ill 8 gif gum ill old pen pit pun ten
then the output is:
Search lower half Search upper half ill is found at index 2Note: string1 < string2 returns true if string1 is alphabetically before string2, and returns false otherwise.
You can only change the line "Your code goes here".
''
#include <iostream>
#include <vector>
#include <string>
using namespace std;
void FindMiddle(vector<string> allWords, string inquiredItem, int startIndex, int endIndex) {
int middleIndex;
int rangeSize;
string middleValue;
rangeSize = (endIndex - startIndex) + 1;
middleIndex = (startIndex + endIndex) / 2;
middleValue = allWords.at(middleIndex);
if (inquiredItem == middleValue) {
cout << inquiredItem << " is found at index " << middleIndex << endl;
}
else if (rangeSize == 1) {
cout << inquiredItem << " is not in the list" << endl;
}
else {
/* Your code goes here */
}
}
int main() {
string inquiredItem;
vector<string> dataList;
int numData;
int i;
string item;
cin >> inquiredItem;
cin >> numData;
for (i = 0; i < numData; ++i) {
cin >> item;
dataList.push_back(item);
}
FindMiddle(dataList, inquiredItem, 0, dataList.size() - 1);
return 0;
}
'''

Step by stepSolved in 2 steps

- (Numerical) Given a one-dimensional array of integer numbers, write and test a function that displays the array elements in reverse order.arrow_forward(Data processing) The answers to a true-false test are as follows: T T F F T. Given a twodimensional answer array, in which each row corresponds to the answers provided on one test, write a function that accepts the two-dimensional array and number of tests as parameters and returns a one-dimensional array containing the grades for each test. (Each question is worth 5 points, so the maximum possible grade is 25.) Test your function with the following data:arrow_forward(Numerical) Write a program that tests the effectiveness of the rand() library function. Start by initializing 10 counters to 0, and then generate a large number of pseudorandom integers between 0 and 9. Each time a 0 occurs, increment the variable you have designated as the zero counter; when a 1 occurs, increment the counter variable that’s keeping count of the 1s that occur; and so on. Finally, display the number of 0s, 1s, 2s, and so on that occurred and the percentage of the time they occurred.arrow_forward
- (Numerical) a. Define an array with a maximum of 20 integer values, and fill the array with numbers input from the keyboard or assigned by the program. Then write a function named split() that reads the array and places all zeros or positive numbers in an array named positive and all negative numbers in an array named negative. Finally, have your program call a function that displays the values in both the positive and negative arrays. b. Extend the program written for Exercise 6a to sort the positive and negative arrays into ascending order before they’re displayed.arrow_forward(Electrical eng.) Write a program that specifies three one-dimensional arrays named current, resistance, and volts. Each array should be capable of holding 10 elements. Using a for loop, input values for the current and resistance arrays. The entries in the volts array should be the product of the corresponding values in the current and resistance arrays (sovolts[i]=current[i]resistance[i]). After all the data has been entered, display the following output, with the appropriate value under each column heading: CurrentResistance Voltsarrow_forwardMark the following statements as true or false. A double type is an example of a simple data type. (1) A one-dimensional array is an example of a structured data type. (1) The size of an array is determined at compile time. (1,6) Given the declaration: int list[10]; the statement: list[5] - list[3] * list[2]; updates the content of the fifth component of the array list. (2) If an array index goes out of bounds, the program always terminates in an error. (3) The only aggregate operations allowable on int arrays are the increment and decrement operations. (5) Arrays can be passed as parameters to a function either by value or by reference. (6) A function can return a value of type array. (6) In C++, some aggregate operations are allowed for strings. (11,12,13) The declaration: char name [16] = "John K. Miller"; declares name to be an array of 15 characters because the string "John K. Miller" has only 14 characters. (11) The declaration: char str = "Sunny Day"; declares str to be a string of an unspecified length. (11) As parameters, two-dimensional arrays are passed either by value or by reference. (15,16)arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
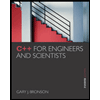
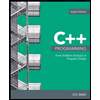
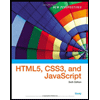
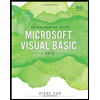
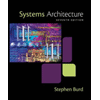