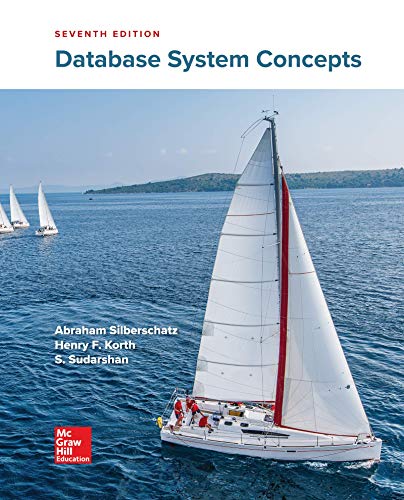
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
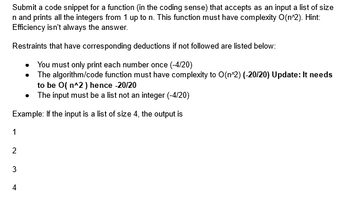
Transcribed Image Text:Submit a code snippet for a function (in the coding sense) that accepts as an input a list of size
n and prints all the integers from 1 up to n. This function must have complexity O(n^2). Hint:
Efficiency isn't always the answer.
Restraints that have corresponding deductions if not followed are listed below:
You must only print each number once (-4/20)
The algorithm/code function must have complexity to O(n^2) (-20/20) Update: It needs
to be O(n^2) hence -20/20
• The input must be a list not an integer (-4/20)
Example: If the input is a list of size 4, the output is
1
2
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- The Fibonacci sequence begins with 0 and then 1 follows. All subsequent values are the sum of the previous two, ex: 0, 1, 1, 2, 3, 5, 8, 13. Complete the fibonacci() function, which has an index n as parameter and returns the nth value in the sequence. Any negative index values should return -1. Ex: If the input is: 7 the output is: fibonacci (7) is 13 Note: Use a for loop and DO NOT use recursion. 461710.3116374.qx3zqy7 LAB ACTIVITY 1 def fibonacci(n): 1234567 4 7.34.1: LAB: Fibonacci sequence 6 #Type your code here. 5 if __name__ start_num = '__main__': int(input()) main.py print(f'fibonacci ({start_num}) is {fibonacci (start_num)}') 0/10 Load default template...arrow_forwardDefine function print_popcorn_time() with parameter bag_ounces. If bag_ounces is less than 3, print "Too small". If greater than 10, print "Too large". Otherwise, compute and print 6 * bag_ounces followed by "seconds". End with a newline. Remember that print() automatically adds a newline. Sample output with input: 7 42 secondsarrow_forwardThe Fibonacci sequence begins with 0 and then 1 follows. All subsequent values are the sum of the previous two, ex: 0, 1, 1, 2, 3, 5, 8, 13. Complete the fibonacci() function, which has an index n as parameter and returns the nth value in the sequence. Any negative index values should return -1. Ex: If the input is: 7 the output is: fibonacci (7) is 13 Note: Use a for loop and DO NOT use recursion.arrow_forward
- 1. Design an algorithm to find the weighted sum of four test scores (https://en.wikipedia.org/wiki/Weight_function). Assume that the weights have been accurately calculated in advance such that their sum equals one. Your algorithm must-read in the four test scores and four corresponding weights in the following order: score1 weight1 score2 weight2 score3 weight3 score4 weight4 Write your algorithm such that it can be run with any set of data values. However, you can test your algorithm on the following sample data to verify that the result is 70: 90 0.10 80 0.20 70 0.30 60 0.40 2. Write an algorithm that prompts the user for the radius, in inches, and price of a pizza, and then reads in those values. Finally, have the algorithm compute and output the pizza’s cost per square inch. 3. Sports exercise advisor algorithm. In this algorithm you will start out with a temperature value in Celsius, so you do not need to ask the user for it. First, convert the temperature to Fahrenheit.…arrow_forwardIn python, The function decodeFromAscii takes a list L of integers in the range 32 through 126. Each item in L is an ASCII code representing a single printable character. The function accumulates the string of characters represented by the list of ASCII codes and returns that string. For example, decodeFromAscii([78, 105, 99, 101, 33]) returns "Nice!" because N is chr(78), i is chr(105), c is chr(99), etc. Note: ASCII was created in the 1960's so programmers could have a standard encoding for common keyboard characters as numbers. It was later expanded to create Unicode so that characters from languages other than English, mathematical symbols, and many other symbols could also be represented by numerical codes. For example: Test Result print(decodeFromAscii([36, 49, 44, 48, 48, 48])) $1,000 print(decodeFromAscii([115, 110, 111, 119])) snowarrow_forwardGiven the following pseudocode: function fun(n) { var outer_count=0; var inner_count=0; var sum = 0; for (var i=0; i 0, as an expression of normal arithmetic and n, what is the value of outer_count that counts the number of times the outer loop executes? Enter an expression Fun.1.inner: Assuming n EN and n > 0, as an expression of normal arithmetic and n, what is the value of inner_count that counts the number of times the inner loop executes? Enter an expressionarrow_forward
- Compute and Plot the ROC Curve Write a function from scratch called roc_curve_computer that accepts (in this exact order): a list of true labels a list of prediction probabilities (notice these are probabilities and not predictions - you will need to obtain the predictions from these probabilities) a list of threshold values. The function must compute and return the True Positive Rate (TPR, also called recall) and the False Positive Rate (FPR) for each threshold value in the threshold value list that is passed to the function. Important: Be sure to reuse functions and code segments from your work above! You should reuse two of your above created functions so that you do not duplicate your code. The function you will write behaves identically to Scikit-Learn's roc_curve function, except that it will take the list of thresholds in as input rather than return them as output. Your function must calculate one value of TPR and one value of FPR for each of the threshold values in the list.…arrow_forwardUp for the count def counting_series (n): The Champernowme word 1234567891011121314151617181920212223., also known as the counting series, is an infinitely long string of digits made up of all positive integers written out in ascending order without any separators. This function should return the digit at position n of the Champernowne word. Position counting again starts from zero for us budding computer scientists. Of course, the automated tester will throw at your function values of n huge enough that those who construct the Champernowne word as an explicit string will run out of both time and space long before receiving the answer. Instead, note how the fractal structure of this infinite sequence starts with 9 single-digit numbers, followed by 90 two-digit numbers, followed by 900 three-digit numbers, and so on. This observation gifts you a pair of seven league boots that allow their wearer skip prefixes of this series in exponential leaps and bounds, instead of having to crawl…arrow_forwardUsing recursion, write a function sum that takes a single argument n and computes the sum of all integers between 0 and n inclusive. Do not write this function using a while or for loop. Assume n is non-negative. def sum(n): """Using recursion, computes the sum of all integers between 1 and n, inclusive. Assume n is positive. >>> sum(1) 1 >>> sum(5) # 1 + 2 + 3+ 4+ 5 15 "*** YOUR CODE HERE ***"arrow_forward
- Please follow all the steps givenarrow_forwardIN JAVA SCRIPT Create a function that returns the thickness (in meters) of a piece of paper after folding it n number of times. The paper starts off with a thickness of 0.5mm. Examples numLayers (1) "0.001m" // Paper folded once is 1mm (equal to 0.001m) numLayers (4) "0.008m" // Paper folded 4 times is 8mm (equal to 0.008m) numLayers (21) "1048.576m" // Paper folded 21 times is 1048576mm (equal to 1048.576m) (Ctrl)arrow_forwardEstimated Completion Time: 1 minute Given the following function header: def longest_chain(1st: list[int]) -> int: Given a list of integers, return the length of the longest chain of 1's that start from the beginning. Precondition: 1st is non-empty which one of the cases below is the least useful to check? Select the best answer. O longest_chain([0]) O longest_chain([0) O longest_chain([1, 1, 0]) O longest_chain([1, 1, 0, 1) O longest_chain([1, 0, 1, 1))arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
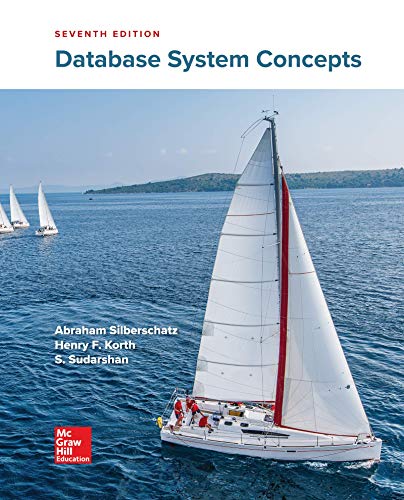
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
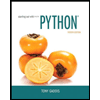
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
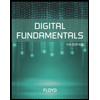
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
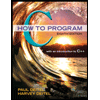
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
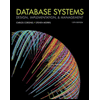
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
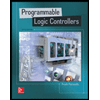
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education