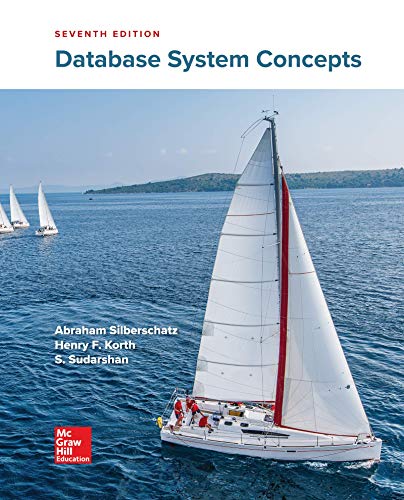
Concept explainers
Need help with a C++ commenting question:
Indicate where the different constructors, assignment operator= and desctructor will run with a comment under the relevant line of code.
Start in main and use the following tags to indicate the function that will run:
Default constructor <object name>
String constructor <object name>
Copy constructor <object name>
Assignment operator
Destructor <object name>
Substitute the variable name for the object constructed or destructed. Use a comma to separate if multiple functions are run on a line.
#include <iostream> | ||
2 | using namespace std; | |
3 | ||
4 | class Student { | |
5 | public: | |
6 | friend Student OutputDuplicate (Student obj); // friend function | |
7 | ||
8 | Student (); // Default constructor | |
9 | ||
10 | Student (string n); // String constructor | |
11 | ||
12 | Student (const Student & obj); // Copy constructor | |
13 | ||
14 | Student& operator= (const Student & right); // Assignment operator | |
15 | ||
16 | ~Student() ; // Destructor | |
17 | ||
18 | private: | |
19 | string name; | |
20 | ||
21 | }; | |
22 | ||
23 | // Default constructor | |
24 | Student::Student() : name("no name") | |
25 | { | |
26 | // Intentionally left blank | |
27 | } | |
28 | ||
29 | // String constructor | |
30 | Student::Student(string n) : name(n) | |
31 | { | |
32 | // Intentionally left blank | |
33 | } | |
34 | ||
35 | // Copy constructor | |
36 | Student::Student(const Student & obj) : name(obj.name) | |
37 | { | |
38 | // Intentionally left blank | |
39 | } | |
40 | ||
41 | // Assignment operator | |
42 | Student& Student::operator= (const Student & right) | |
43 | { | |
44 | name=right.name; | |
45 | return (*this); | |
46 | } | |
47 | ||
48 | // Destructor | |
49 | Student::~Student() | |
50 | { | |
51 | // Intentionally left blank | |
52 | } | |
53 | ||
54 | ////////////////////////////////////////////////////////////////////////////// | |
55 | // MARK UP FROM HERE DOWN | |
56 | ////////////////////////////////////////////////////////////////////////////// | |
57 | ||
58 | // friend function | |
59 | Student OutputDuplicate (Student obj) | |
60 | { | |
61 | Student tmp("Jack"); | |
62 | ||
63 | cout <<"Creating a new student Jame smith\n"; | |
64 | Student * anotherObj = new Student ("Jane Smith"); | |
65 | ||
66 | *anotherObj = obj; | |
67 | return tmp; | |
68 | } | |
69 | ||
70 | int main() | |
71 | { | |
72 | Student a, b ("John Smith"); | |
73 | ||
74 | cout <<"calling OutputDuplicate\n\n"; | |
75 | ||
76 | a = OutputDuplicate (b); | |
77 | ||
78 | cout <<"\nExiting\n"; | |
79 | } |

The given is a c++ code which consist of below features:
1. A class with name Student
2. A default consutructor
3. A String constructor
4. An assignment operator implementation
The requirement is to find the statements in the main function that calls constructor, destructor and other operators.
Step by stepSolved in 2 steps with 1 images

- When defining an array of a classtype, a C++ programmer has no way to specify which overloaded constructor is used on each declared array element, as the default no-argument constructor will always be used no matter what. True O Falsearrow_forwardC++arrow_forwardUsing C++ and Visual Studios Using your own creativity, make a set of class templates that have these features: For this class template, put everything in one place--do not declare the member functions and have separate definition of the member functions elsewhere. Keep them in one place. Include a private variable. Include a constructor that loads the private variable when constructed. Include a destructor that clears the private variable to zero. Include set and get functions to set and get the private variable.arrow_forward
- // TYPEDEFS and MEMBER CONSTANTS for the sequence class:// typedef ____ value_type// sequence::value_type is the data type of the items in the sequence.// It may be any of the C++ built-in types (int, char, etc.), or a// class with a default constructor, an assignment operator, and a// copy constructor.//// typedef ____ size_type// sequence::size_type is the data type of any variable that keeps// track of how many items are in a sequence.//// static const size_type DEFAULT_CAPACITY = _____// sequence::DEFAULT_CAPACITY is the default initial capacity of a// sequence that is created by the default constructor.//// CONSTRUCTOR for the sequence class:// sequence(size_type initial_capacity = DEFAULT_CAPACITY)// Pre: initial_capacity > 0// Post: The sequence has been initialized as an empty sequence.// The insert/attach functions will work efficiently (without// allocating new memory) until this capacity is reached.// Note: If Pre is not…arrow_forwardIt is necessary to outline the regulations that apply to functions with default parameters.arrow_forwardC++YOU ONLY NEED TO EDIT date.h, dateOFYear.h, and dateOFYear.cpp date.h | date.cpp | dateOfYear.h | dateOfYear.cpp | dateTest.cpp You have been provided a Date class. Each date contains day and month parameters, a default and a custom constructor, mutator and accessor functions for the parameters, and input and output functions called within operator >> and <<. You will be writing a DateOfYear class, which expands on the Date class by adding a year parameter. This will be achieved via inheritance – DateOfYear is the derived class of the Date base class. In DateOfYear, will need to write… The include guards for the class A default constructor that initializes all parameters to 1 A custom constructor that takes in a month, day, and year to initialize the parameters Mutator and accessor for the year parameter Redefined versions of inputDate and outputDate that takes into account the new year parameter. The driver program, dateTest.cpp, is already set for use with both Date…arrow_forward
- structions: Write the following programs in C# using concepts learnt in this chapter and abmit the .cs file with the screenshot of your output for each question in the Lab Assignment 2 Submission page. 1. FRENCH TRANSLATOR Look at the following list of French words and their meanings: In French: gauche milieu droite middle In English: right left Create an application that translates the French words to English. The form should have three buttons, one for each French word. When the user clicks a button, the application should display the English translation in a Label control.arrow_forwardPLease write the code in C++. Thank you so much. Write A Class Declare a class/struct named NutritionData that contains these private fields foodName (string) servingSize (int) calFromCarb (double) calFromFat (double) calFromProtein (double) Use the data types in parentheses for the fields. Note that you need to use the proper C++ syntax for the fields. Each field should have a comment documenting what it is for. Place one comment above each field. Add the public default constructor. Read the textbook for the syntax of the default constructor. Write the body of the default constructor inline. The default constructor initializes the fields so that the food name is an empty string and all other fields are 0 for int and 0.0 for double. Add public mutator member functions to set the fields. One mutator member function for each field. Each mutator member function's name should begin with the word 'set' followed by the field name with the first letter changed to uppercase. Each member…arrow_forward/ CONSTANT// static const int DEFAULT_CAPACITY = ____// IntSet::DEFAULT_CAPACITY is the initial capacity of an// IntSet that is created by the default constructor (i.e.,// IntSet::DEFAULT_CAPACITY is the highest # of distinct// values "an IntSet created by the default constructor"// can accommodate).//// CONSTRUCTOR// IntSet(int initial_capacity = DEFAULT_CAPACITY)// Post: The invoking IntSet is initialized to an empty// IntSet (i.e., one containing no relevant elements);// the initial capacity is given by initial_capacity if// initial_capacity is >= 1, otherwise it is given by// IntSet:DEFAULT_CAPACITY.// Note: When the IntSet is put to use after construction,// its capacity will be resized as necessary.//// CONSTANT MEMBER FUNCTIONS (ACCESSORS)// int size() const// Pre: (none)// Post: Number of elements in the invoking IntSet is returned.// bool isEmpty() const// Pre: (none)//…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
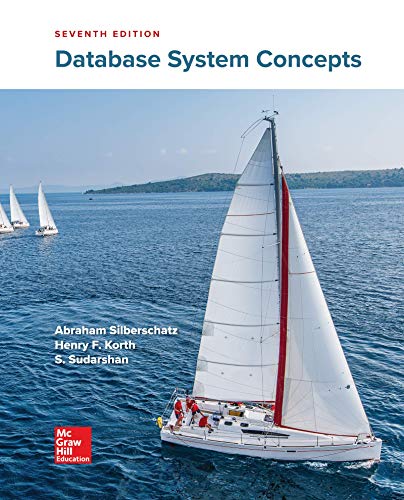
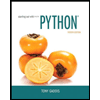
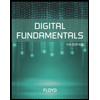
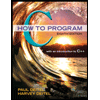
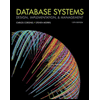
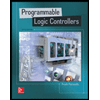