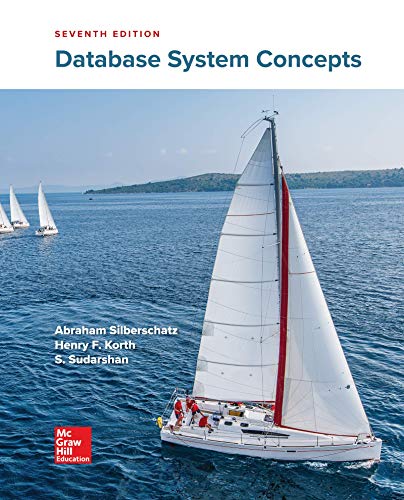
The Caesar Cipher
Recall that the Caesar Cipher uses alphabets as the primary source of information but shifted a certain number of letters to the left or right as a key to create the encrypted data. The Caesar Cipher is easily hacked because of the simple technique used to build the key that encrypts and decrypts the data.
In this lab, the key is the number of alphabets shifted, and the direction is always toward the increasing alphabetical order. This provides 26 ways of encrypting a message and therefore makes it easier to be hacked by guessing the key within the range of 0 - 25.
Write the function caesar_hack( ) that takes three parameters: a Caesar Cipher encrypted message, an alphabet list, and the original message. Function caesar_hack( ) uses a Brute Force Attack to find the key that decrypts the encrypted message correctly. This would typically be a technique used by an unauthorized user.
Use a nested for loop in the function caesar_hack( ) to try all the possible keys. For each key, check the encrypted letter position based on the alphabet letter and attempt to decrypt the message. If the decrypted message is the same as the original message, return the key value and the decrypted message. If a key is not found, return 99 as the key and "Error: Key not found!" as the message. Note: The decrypted message is always in upper case due to the uppercase letters in the alphabet list. Convert the original message to upper case before being compared.
In main: Add a call to the caesar_hack() function. Make sure you capture both of your returned variables. Then add control structures that output the returned error message if the returned key is 99, or output "Successful attempt found! Key = the returned key" followed by "Secret message: the returned message" in a new line.
Ex: If the input is:
4
then the output is:
Brute Force Hack Attempt: Encrypted Message: XLMW MW E WIGVIX QIWWEKI Successful Attempt found! Key = 4 Secret message: THIS IS A SECRET MESSAGE
#Caesar Cipher Encryption function
def caesar_cipher_encrypt(text, key, a):
text = text.upper()
encrypted_Text = ""
for i in text:
if i in a:
index = (a.find(i) + key) % len(a)
encrypted_Text = encrypted_Text + a[index]
else:
encrypted_Text = encrypted_Text + i
return encrypted_Text
#Caesar Cipher Decrypt function
def caesar_cipher_decrypt(text, key, a):
text = text.upper()
decrypted_Text = ""
for i in text:
if i in a:
index = (a.find(i) - key) % len(a)
decrypted_Text = decrypted_Text + a[index]
else:
decrypted_Text = decrypted_Text + i
return decrypted_Text
# TODO Create a function called caesar_hack( text, a, check ) that takes a Caesar Cipher encrypted message, an alphabet list, and the original message in main.
# This function will attempt to hack an encrypted message. Use a nested for loop to find the key between 0 & 25.
# Check where the letter is found in the alphabet list based on the encrypted text letter sent to this function.
# Check if the decrypted message is the same as the original message.
# If a key is found , return the key value and the decrypted message.
# If not found return 99 as the key and "Error: Key not found!" as the message.
def caesar_hack(text, a, check):
alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
text = "This is a secret message"
shift = int(input()) #Number between 1 & 25
encrypted_data = caesar_cipher_encrypt(text, shift, alphabet)
print('Brute Force Hack Attempt: ')
print ('Encrypted Message: ', encrypted_data)
# TODO: Add a call to caesar_hack (encrypted_data, alphabet, text) return it to two variables k (key) & h (hacked message)
# TODO: If k == 99 print the returned message
# Else - print 'Successful Attempt found! Key =' k variable
# Add a print 'Secret message:' h variable

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Both parts pleasearrow_forwardBob has sent his secret number m to Alice via secure RSA encryption. Break his ciphertext to find m. To factorize the number N, you can use the program. Alice's public key (e, N) = (17, 29329): Bob's ciphertext c = 16469arrow_forwardAssume Alice and Bob both have public and private keys in a public key setting. If Alice wants to send Bob a signed message, Alice first hashes her message and the hash using _'s key. decrypts/ Alice/ private encrypts/Alice/private decrypts/ Bob/ private encrypts/ Bob/publicarrow_forward
- On Groundhog Day, the groundhog randomly chooses and sends one of two possible messages: m0 = SIXMOREWEEKSOFWINTER m1 = SPRINGARRIVESINMARCH. To add to the mystery, the groundhog encrypts the message using one of the following methods: shift cipher, Vigenère cipher with key length 4 (using four distinct shifts), one-time pad. For each of the following ciphertexts, determine which encryption methods could have produced that ciphertext, and for each of these possible encryption methods, decrypt the message or explain why it is impossible to decrypt. a. ABCDEFGHIJKLMNOPQRST b. UKZOQTGYGGMUQHYKPVGT c. UQUMPHDVTJYIUJQQCSFL.arrow_forwardPlz don't use chat gptarrow_forwardIs it possible to use software on a desktop computer to make secret keys for use in cryptographic protocols in a way that keeps them safe and lets them be used?arrow_forward
- suppose the RSA cryptosystem is used for sending secret messages with the private key (15,3) and the cipher text "4" is received. what is the plaintext?arrow_forwardAlice sets up an RSA public/private key, but instead of using two primes, she chooses three primes p, q, and r and she uses n=pqr as her RSA-style modulus. She chooses an encryption exponent e and calculates a decryption exponent d. Encryption and Decryption are defined: C ≡ me mod n and m ≡ Cd mod n where C is the ciphertext corresponding to the message m. Decryption: de ≡ 1 mod φ(n) | Let p = 5, q = 7, r = 3, e = 11, and the decryption exponent d = -13. n = 105 & φ(n) = 48 Q: Alice upgrades to three primes that are each 200 digits long. How many digits does n have?arrow_forwardIn this problem, you will implement a transposition cipher that, instead of dividing the original text into odd and even characters, separates it into three sets of characters. As an example, we will use this 1977 quote by Digital Equipment Corp. president Ken Olson -- "There is no reason anyone would want a computer in their home." (Spaces are represented with the square u-like character.) There is no reason anyone would want a computer in their home. Rail 1: T r S d i t i h a C. e Rail 2: e a u a u in h Rail 3: a W n. m m Example three-rail transposition. The resulting encrypted text is produced by reading the text horizontally, i.e., adding the three rails: Rail 1 + Rail 2 + Rail 3 = **“Trinrs yeoda cpeitihehesoeoao u naournhro.e annnwlwt mt e m" (without the quotes). Requirements: Implement the three-rail transposition cipher above, placing your code in the file p4_2.py (template I provided, replace comment at the beginning of the file with your own code). Feel free to develop your…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
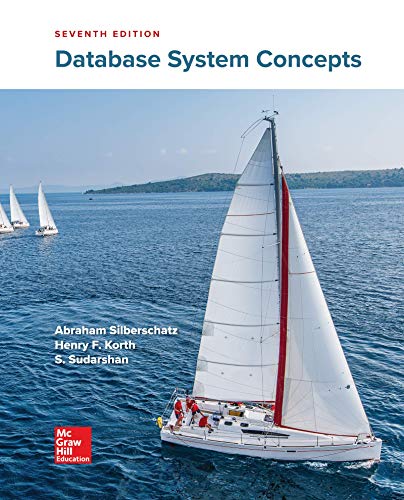
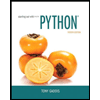
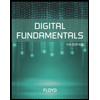
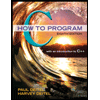
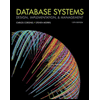
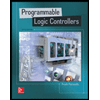