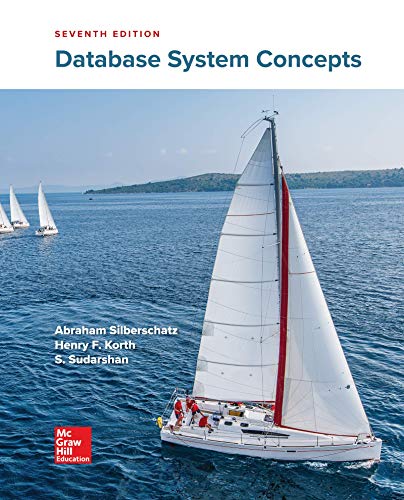
The following code is implementing a Treasure map use the A*
start place = (0, 0)
Treasure location: (29, 86)
Path to treasure:
(0, 0)
Python Code:
import heapq
import random
class Tile:
def __init__(self, x, y):
self.x = x
self.y = y
self.is_obstacle = False
self.g_cost = 0
self.h_cost = 0
self.f_cost = 0
self.parent = None
# Define comparison methods for Tile objects based on their f_cost
def __lt__(self, other):
return self.f_cost < other.f_cost
def __eq__(self, other):
return self.f_cost == other.f_cost
class Map:
def __init__(self, width, height):
self.width = width
self.height = height
self.tiles = [[Tile(x, y) for y in range(height)] for x in range(width)]
self.start_tile = self.get_tile(0, 0)
self.treasure_tile = None
self.generate_treasure()
def generate_treasure(self):
self.treasure_tile = self.get_random_tile()
self.treasure_tile.is_obstacle = True
def get_random_tile(self):
x = random.randint(0, self.width - 1)
y = random.randint(0, self.height - 1)
return self.get_tile(x, y)
def get_tile(self, x, y):
return self.tiles[x][y]
def get_adjacent_tiles(self, tile):
adjacent_tiles = []
for x in range(tile.x - 1, tile.x + 2):
for y in range(tile.y - 1, tile.y + 2):
if x == tile.x and y == tile.y:
continue
if x < 0 or x >= self.width or y < 0 or y >= self.height:
continue
adjacent_tiles.append(self.get_tile(x, y))
return adjacent_tiles
class AStar:
def __init__(self, game_map, start_tile, treasure_tile):
self.game_map = game_map
self.start_tile = start_tile
self.treasure_tile = treasure_tile
def find_path(self):
open_list = []
closed_list = []
start_tile = self.start_tile
treasure_tile = self.treasure_tile
heapq.heappush(open_list, (start_tile.f_cost, start_tile))
while len(open_list) > 0:
current_tile = heapq.heappop(open_list)[1]
closed_list.append(current_tile)
if current_tile == treasure_tile:
path = []
while current_tile != start_tile:
path.append((current_tile.x, current_tile.y))
current_tile = current_tile.parent
path.append((start_tile.x, start_tile.y))
path.reverse()
return path
adjacent_tiles = self.game_map.get_adjacent_tiles(current_tile)
for tile in adjacent_tiles:
if tile.is_obstacle or tile in closed_list:
continue
g_cost = current_tile.g_cost + 1
h_cost = abs(tile.x - treasure_tile.x) + abs(tile.y - treasure_tile.y)
f_cost = g_cost + h_cost
if (tile.f_cost, tile) in open_list:
if tile.g_cost > g_cost:
tile.g_cost = g_cost
tile.h_cost = h_cost
tile.f_cost = f_cost
tile.parent = current_tile
heapq.heapify(open_list)
else:
tile.g_cost = g_cost
tile.h_cost = h_cost
tile.f_cost = f_cost
tile.parent = current_tile
heapq.heappush(open_list, (tile.f_cost, tile))
return None
# Create the game board
game_board = Map(100, 100)
# starting_place = Tile(0, 0)
starting_place = game_board.start_tile
treasure_place = game_board.treasure_tile
print("Treasure location:", (treasure_place.x, treasure_place.y))
# Find the path using A* algorithm
path_finder = AStar(game_board, starting_place, treasure_place)
path = path_finder.find_path()
if path:
print("Path to treasure:")
for step in path:
print(step)
else:
print("No path to treasure found.")

Step by stepSolved in 5 steps

- Use Python for this question: Implement a function findMixedCase that accepts a single argument, a list of words, and returns the index of the first mixed case word in the list, if one exists, (a word is mixed case if it contains both upper and lower case letters), and returns -1 if the all words are either upper or lower case Output for this question is in the attached image:arrow_forwardSolved in pythonarrow_forwardPlease send me answer of this question immediately and i will give you like sure sirarrow_forward
- In Python, Compute the: sum of squares total (SST), sum of squares regression (SSR), sum of squares error(SSE), and the r, R^2 values. You can use the sum() and mean() built-in functions. Here are the x and y values to use. x = [-5,-1,3,7,5,10] y = [-10,-3,5,8,7,10]arrow_forwardWrite using pythonarrow_forwardMake A Dice Simulator in Python. First, we import the library that allows us to choose random numbers. import random Now, we can generate a random number and save it in a variable, We will call it rolled Python library random has a function called randint (). The randint (min number, max number) requires 2 parameters (the lowest number and the highest number between we will pick our number randomly). In this case, our dice goes between 1-6. rolled = random.randint (1,6) If we want to show our selected number, we can use print (). Your code should look like this: import random rolled - random.randint(1,6) print(rolled) Nice, we already have our main engine working, now it's time to make it look more appealing. To do that we will add some improvements (we will use a new variable to store random generated numbers - rolled_num) import random rolled num - random.randint(1,6) print("You rolled: ", rolled num) If we run the code again, we should see a little message and the random number. Our…arrow_forward
- Implement the following function: Code should be written in python.arrow_forwardWrite a Python program that removes the duplicates from a list. Use inputs a list of integers from the user, and removes the duplicate list elements, plus outputs their min and max values. (the program should have more than 1 function and leave comments as explanations of what does the code does). Ex (in pictures below):arrow_forwardWhat Python code will solve the following: The goal of this section is to estimate the probabilities Pij (transition probabilities) associated with finding food from any location (i, j) inside the maze. A rat will be considered successful in finding food if the probability of finding food from a given location exceeds 0.5. 1. Use Equation (1) to write down equations for each probability Pij for i = 1, 2, · · · , 6, j = 1, 2, 3, · · · , 5. Equation 1 is : Pij = 1/4Pi-1j + 1/4 Pi+1j +1/4 Pij-1 + 1/4Pij+1 2. Notice that your equations constitutes a system of linear equations with 30 equations and 30 unknowns, with the unknowns as the transition probabilities. Identify the coefficient matrix for the system of equations and write your system in the form Ap = b, where b is some constant vector and p the vector of transition probabilities, ordered from the upper left hand corner of the maze to the lower right hand corner. The goal is to solve the system for p. 2 3. How would you characterize…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
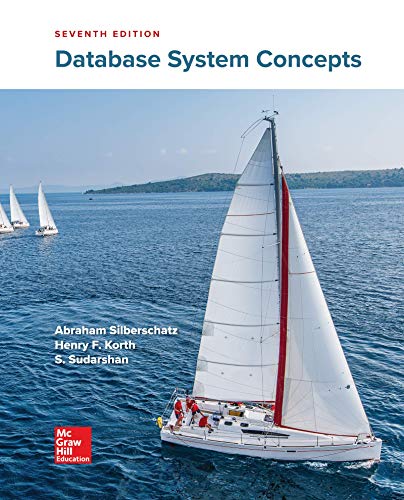
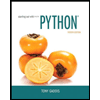
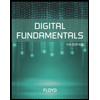
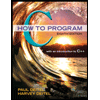
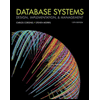
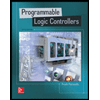