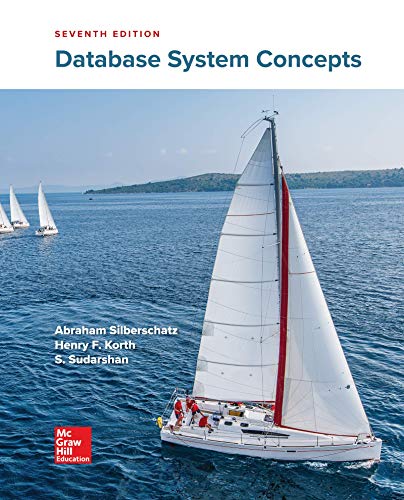
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
There are a few errors in this java code: can you fix it please, its basically a debugging exercise:
public static int sum(int n){
int n;
for (int i=1; i<=n;i--){
sum++;
}
return sum;
}
}
![The image shows a screenshot of the Eclipse IDE with Java code editor open. Below is the transcription and explanation based on the visible content:
### Project Explorer Panel
- **Lab 9**
- JRE System Library [JavaSE-14]
- `src (default package)`
- `Apples.java`
- `DoWhileLoops.java`
- `ForLoops.java`
- `WhileLoops.java`
- **Quiz**
- JRE System Library [JavaSE-14]
- `src (default package)`
- `Blue.java`
- `Pumpkins.java`
### Java Code Editor
The file `Blue.java` is open in the editor with the following code:
```java
public class Blue {
public static int sum(int n){
int sum;
for (int i = 1; i <= n; i--){
sum++;
}
return sum;
}
}
```
### Error in Console
The console displays an error message:
```
Error: Main method must return a value of type void in Class Blue, please define the main method as:
public static void main(String[] args)
```
### Explanation
- The code defines a `public class Blue` with a `static int` method called `sum`.
- The method `sum` takes an integer `n` as a parameter, initializes an integer `sum`, and attempts to increment it in a `for` loop with a decrementing iterator, which will result in an infinite loop if `i` starts at 1.
- The method returns the value of `sum`, but `sum` is not initialized, which would lead to a compilation error.
- The console error indicates that the `main` method is missing, which is necessary to execute the program.
### Recommendations for Correction
1. **Initialization Error:** Initialize the `sum` variable.
2. **Loop Direction:** Correct the loop condition and iterator modification to `i++` if intended increment within the loop.
3. **Main Method:** Add a `main` method to execute the program, as per console message guidance:
```java
public static void main(String[] args) {
// Example execution
int result = sum(5);
System.out.println("Sum: " + result);
}
```
This setup is suitable for educational purposes to understand common beginner errors in Java programming.](https://content.bartleby.com/qna-images/question/7d848391-a5b1-45af-b291-d5739fd6d88e/eb841c67-91ab-4e50-b7ba-efc06f55b1f5/s5hmdj_thumbnail.png)
Transcribed Image Text:The image shows a screenshot of the Eclipse IDE with Java code editor open. Below is the transcription and explanation based on the visible content:
### Project Explorer Panel
- **Lab 9**
- JRE System Library [JavaSE-14]
- `src (default package)`
- `Apples.java`
- `DoWhileLoops.java`
- `ForLoops.java`
- `WhileLoops.java`
- **Quiz**
- JRE System Library [JavaSE-14]
- `src (default package)`
- `Blue.java`
- `Pumpkins.java`
### Java Code Editor
The file `Blue.java` is open in the editor with the following code:
```java
public class Blue {
public static int sum(int n){
int sum;
for (int i = 1; i <= n; i--){
sum++;
}
return sum;
}
}
```
### Error in Console
The console displays an error message:
```
Error: Main method must return a value of type void in Class Blue, please define the main method as:
public static void main(String[] args)
```
### Explanation
- The code defines a `public class Blue` with a `static int` method called `sum`.
- The method `sum` takes an integer `n` as a parameter, initializes an integer `sum`, and attempts to increment it in a `for` loop with a decrementing iterator, which will result in an infinite loop if `i` starts at 1.
- The method returns the value of `sum`, but `sum` is not initialized, which would lead to a compilation error.
- The console error indicates that the `main` method is missing, which is necessary to execute the program.
### Recommendations for Correction
1. **Initialization Error:** Initialize the `sum` variable.
2. **Loop Direction:** Correct the loop condition and iterator modification to `i++` if intended increment within the loop.
3. **Main Method:** Add a `main` method to execute the program, as per console message guidance:
```java
public static void main(String[] args) {
// Example execution
int result = sum(5);
System.out.println("Sum: " + result);
}
```
This setup is suitable for educational purposes to understand common beginner errors in Java programming.
Expert Solution

arrow_forward
Step 1
- To resolve this error you have to declare the main method which is the initial method to be executed.
- In the sum function, you've already defined int n and you're declaring the same variable inside the sum function again.
- Initialize the sum variable.
- You need to increment the i variable in for loop in order to loop over n elements. (i++)
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- public static int countIt (int n) { int count = 0; for (int i = n; i > 0; i /= 2) for (int j = 0; j < 10; j++) count += 1; return count; } Big-O notation: Explanation:arrow_forwardwrite a code in java (using recursion)arrow_forwardDetermine whether a string is a palindromeA palindrome is a string of characters that reads the same from right to left as it does from left to right, regardless of punctuation and spaces.The specifications for this assignment are: •Write and test a non-recursive solution in Java that determines whether a string is a palindrome •Your program should consist of at least two methods: (1) the main method (2) the method which performs the task of determining whether the specified string is a palindrome. You should name this method isPalindrome. You should name the class that contains your “main” method and the isPalindrome method FindPalindrome. •You must use a Stack and a Queue in your solution: Write your own Stack and Queue based on the Vector in the Java API and use those in your solution. You should name those classes StackVector and QueueVector respectively. You already have access to the relevant exception classes and interfaces for the above ADTs. •All of your belong to a Java…arrow_forward
- Complete the convert() method that casts the parameter from a double to an integer and returns the result.Note that the main() method prints out the returned value of the convert() method. Ex: If the double value is 19.9, then the output is: 19 Ex: If the double value is 3.1, then the output is: 3 code: public class LabProgram { public static int convert(double d){ /* Type your code here */ } public static void main(String[] args) { System.out.println(convert(19.9)); System.out.println(convert(3.1)); }}arrow_forward// The language is java Part I: Use of recursive method (4 points for students who need recursion/extracredit) Description: Code a class called RecursiveMultiply in which it will accept two integer arguments entered from user; pass these integers to a recursive method that returns the multiplication result of these two integers. The multiplication can be performed as repeated addition, for example, if two integers are 7 and 4: 8 * 4 = 4 + 4 + 4 + 4 + 4 + 4 + 4 + 4 You will write the application with GUI and recursion to display the result in a noneditable text field, based on the user’s entry from two text fields, while an OK button is pressed. You will utilize the exception handling code as you did in your Lab 4 and Lab 5 to verify if data entered in the text fields are valid (numerical and positive data only). You will make your own decision if there is any explanation that is not described in this specification. Finally, code a driver program that will test your class…arrow_forwardimport java.util.Scanner; public class LabProgram { // Recursive method to draw the triangle public static void drawTriangle(int baseLength, int currentLength) { if (currentLength <= 0) { return; // Base case: stop when currentLength is 0 or negative } // Calculate the number of spaces needed for formatting int spaces = (baseLength - currentLength) / 2; if (currentLength == baseLength) { // If it's the first line, don't output spaces before the first '*' System.out.println("*".repeat(currentLength) + " "); } else { // Output spaces and asterisks System.out.println(" ".repeat(spaces) + "*".repeat(currentLength) + " "); } // Recursively call drawTriangle with the reduced currentLength drawTriangle(baseLength, currentLength - 2); } public static void drawTriangle(int baseLength) { drawTriangle(baseLength, baseLength); } public static…arrow_forward
- Question #4: Re-write the program of Question #3 using data files. The input data numbers.txt contains (positive) integer numbers. Each time the program reads a number, check whether it is a prime and stores the result in the output file Results.txt. 373 373 is Prime!! 11 11 is Prime!! 2552 is NOT prime!! 5 is Prime!! 3561 is NOT prime!! 2552 3561 numbers.txt Results.txtarrow_forwardFix the code below so that there is a function that calculate the height of the triangle. package recursion; import javax.swing.*;import java.awt.*; /** * Draw a Sierpinski Triangle of a given order on a JPanel. * * */public class SierpinskiPanel extends JPanel { private static final int WIDTH = 810; private static final int HEIGHT = 830; private int order; /** * Construct a new SierpinskiPanel. */ public SierpinskiPanel(int order) { this.order = order; this.setMinimumSize(new Dimension(WIDTH, HEIGHT)); this.setMaximumSize(new Dimension(WIDTH, HEIGHT)); this.setPreferredSize(new Dimension(WIDTH, HEIGHT)); } public static double height(double size) { double h = (size * Math.sqrt(3)) / 2.0; return h; } /** * Draw an inverted triangle at the specified location on this JPanel. * * @param x the x coordinate of the upper left corner of the triangle * @param y the y…arrow_forwardHow do I code: public static int sum(int[] arr) public static int sum(int[] arr, int firstIndex, int lastIndex) public static double average(int[] arr) in java?arrow_forward
- Write a Non tail recursion and a tail recursion method in Java with a test class that does the following: The factorial of a positive integer n —which we denote as n!—is the product of n and the factorial of n 1. The factorial of 0 is 1. Write two different recursive methods in Java that each return the factorial of n. Please and Thank youarrow_forwardPROBLEM STATEMENT: Add two numbers together and return the resulting sum. public class AddTwoTogether{public static int solution(int x, int y){// ↓↓↓↓ your code goes here ↓↓↓↓return 0; Can you help me write this in JAVAarrow_forwardQuestion: Your task is to find the number of ways that you can put those brackets in such a way that they will be always balanced after taking the n pair of brackets from the user in Java Language.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
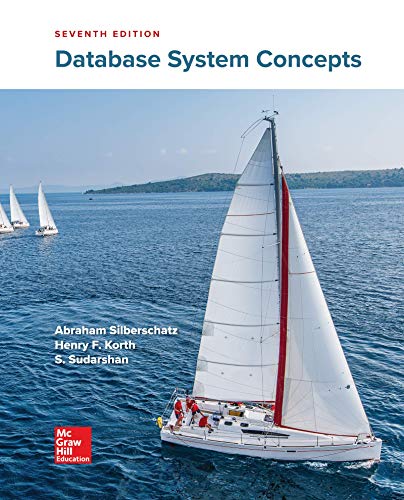
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
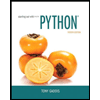
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
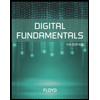
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
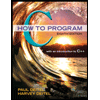
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
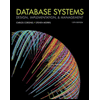
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
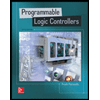
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education