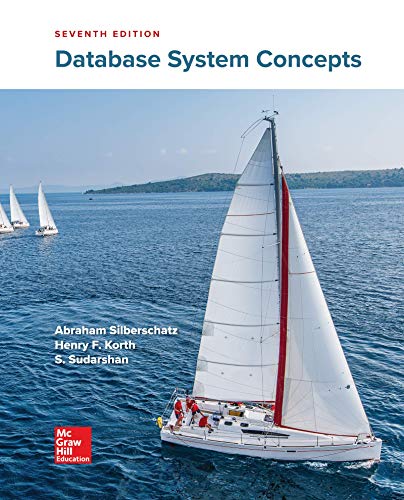
This C++ assignment uses the same account class created in Lab #4 with a more generally useful interface. It reads commands from a bank.txt file. Your program must process the following
four commands:
Create account amount
Deposit account amount
Withdraw account amount
Balance account
In all of the above commands, account is an integer and amount is a double.
The following program behavior is required –
1. Valid account numbers are 1-9. This requires the program to have an array of references
to Account objects.
2. If the first word on any line contains a command other than the four listed above, an
error message should be displayed and that line ignored.
3. The create command creates a new account object with the given account number and
initial balance. If an account already exists with that number an error message should be
displayed and the command ignored.
4. The Deposit and Withdraw commands perform the indicated operation on an existing
account. If no account with that number has been created, an error message is
displayed and the command ignored.
5. The Balance command displays the balance of the requested account. No change to the
account occurs. If no account with that number has been created, an error message is
displayed and the command ignored.
----
Previous account class used in previous lab:
#ifndef Account_h
#define Account_h
using namespace std;
class Account
{
private:
int id;
double balance;
public:
//default constructor
Account()
{
id = 0;
balance = 0;
}
//overload constructor
Account(int newID, double initialBalance)
{
this->id = newID;
this->balance = initialBalance;
}
//sets id
void setId(int newID)
{
this->id = newID;
}
//returns id
int getId()
{
return this->id;
}
//sets balance
void setBalance(double newBalance)
{
this->balance = newBalance;
}
//returns balance
double getBalance()
{
return this->balance;
}
//withdraws from balance
void withdraw(double amount)
{
this->balance = this->balance - amount;
}
//adds to balance
void deposit(double amount)
{
this->balance = this->balance + amount;
}
};
#endif
----
bank.txt file:
Create 1 1000.01
Create 2 2000.02
Create 3 3000.03
Deposit 1 11.11
Deposit 2 22.22
Withdraw 4 5000.00
Create 4 4000.04
Withdraw 1 0.10
Balance 2
Withdraw 2 0.20
Deposit 3 33.33
Withdraw 4 0.40
Bad Command 65
Balance 1
Balance 2
Balance 3
Balance 4

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- For C++ I am kinda suck for this part and for pseudocode tooAssume that you have a file that contains the weekly average prices for a gallon of gas in the United States for the past 3 years. The data is stored in the file as records. Each record contains the average price of a gallon of gas on a specific date. Each record contains the following fields: The month, stored as an integer. January = 1, February = 2, etc. The day of the month, stored as an integer. The year, stored as an integer. The average price of a gallon of gas on the specified date, stored as a real number, rounded to 3 decimal places. Design a program that reads the file and displays the lowest and highest gas prices, as well as the dates for those prices.arrow_forwardX609: Magic Date A magic date is one when written in the following format, the month times the date equals the year e.g. 6/10/60. Write code that figures out if a user entered date is a magic date. The dates must be between 1 - 31, inclusive and the months between 1 - 12, inclusive. Let the user know whether they entered a magic date. If the input parameters are not valid, return false. Examples: magicDate(6, 10, 60) -> true magicDate(50, 12, 600) –> falsearrow_forwardPlease written by computer source Unit 4 - Lab – Individual Retirement Account Calculation Assignment An Individual Retirement Account (IRA) is a special bank / brokerage account that a person can use to save money for retirement. An individual can put up to $5,500 per year tax free and the money grows tax free until the person is ready to retire. The tax rules related to IRA’s are complex, but for this assignment, we’ll assume that the person puts in the same amount of money every year (up to $5,500) and the money grows at a constant interest rate. Write a Flowgorithm program that asks the user to input an annual IRA contribution (in dollars), and interest rate (percent), and a time period (years). Calculate the value of the IRA every year for the duration of the time period provided by the user. Be sure and provide user friendly prompts. Include a comment at the beginning of the program with your name, date, and a short description of the program.arrow_forward
- In Python, grades_dict = {'Wally': [87,96,70], 'Eva': [100,87,90], 'Sam': [94,77,90], 'Katie': [100,81,82], 'Bob': [83, 65, 85]} write your own describe function that produces thesame 8 statistical results, for each one of the columns, that the built-in describe() function does.Note 1: Use the sample standard deviation formula (that is, the denominator is: N-1)Note 2: Your algorithm should work for any number of columns not just for 5Note 3: You can use the np.percentile() for the 25% and 75% percentile as well as the sort()built-in functionsarrow_forwardProject: Two Formulas in Separate Class Write two formulas your choice in separate file and you call it in some manner from your from main program. In Java the pic for examplearrow_forwardin C# i need to Write a program named InputMethodDemo2 that eliminates the repetitive code in the InputMethod() in the InputMethodDemo program in Figure 8-5. Rewrite the program so the InputMethod() contains only two statements: one = DataEntry("first");two = DataEntry("second") I'm getting the error Method DataEntry is defined to eliminate repetitive code 0 out of 1 checks passed. Method DataEntry prompts the user to enter an integer and returns the integer Build Status Build Failed Build Output Compilation failed: 1 error(s), 0 warnings InputMethodDemo2.cs(30,1): error CS1035: End-of-file found, '*/' expected Test Contents [TestFixture] public class DataEntryMethodTest { [Test] public void DataEntryTest() { string consoleInput = "97"; int returnedValue; string expectedString = "Enter third integer"; using (var inputs = new StringReader(consoleInput)) { Console.SetIn(inputs); using (StringWriter sw = new StringWriter()) {…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
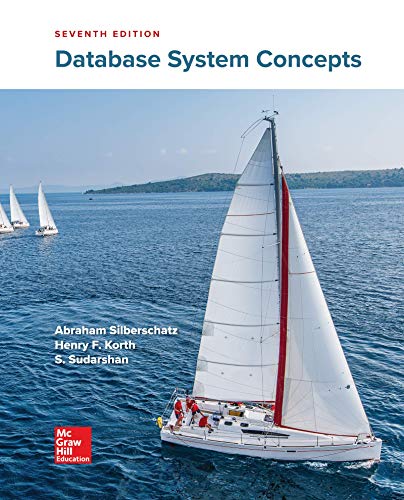
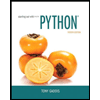
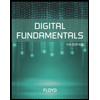
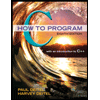
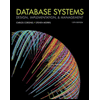
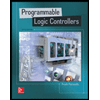