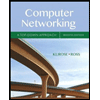
This program requires the main function and a custom value-returning function.
In the main function, code these steps in this sequence:
- use a list comprehension to generate 50 random integers all from -40 to 40, inclusive. These represent Celsius temperatures.
- use an f_string to report the lowest and highest Celsius temperature in the list.
- determine if 0C is in the list. If it is report the index where it first occurs. If it isn't in the list, report that, too.
- use a random module method to create a sublist of 10 unique Celsius temperatures.
- sort this sublist in ascending order.
- pass the Celsius sublist as the sole argument to the custom value-returning function.
In the custom function:
- use either a loop or a list comprehension to create a list of 10 Fahrenheit temperatures equivalent to the Celsius temperatures. A bonus of 3 points will be awarded if a list comprehension is employed.
- return the Fahrenheit list to main.
Back in main:
- use a for loop and the range function to print a table showing the equivalent Celsius and Fahrenheit temperatures in columns with widths that you choose.
- include column headings and display the averages as shown below.
Sample Output
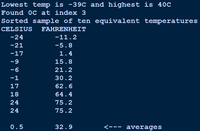

import random
def CelsiusToFahrenheit(lstCel):
lstFah = []
for i in lstCel:
x = (i * 1.8) + 32
lstFah.append(x)
return lstFah
def main():
lst = [random.randint(-40,40) for i in range(50)]
x = min(lst)
y = max(lst)
print(f"Lowest temp is {x}C and highest is {y}C")
if 0 in lst:
ind = lst.index(0)
print("Found 0C at index", ind)
else:
print("0C is not in the list")
lstCel = []
x = random.randint(-40, 40)
for i in range(10):
while x in lstCel:
x = random.randint(-40, 40)
lstCel.append(x)
lstCel.sort()
print("Sorted list of ten equivalent temperatures")
lstFah = CelsiusToFahrenheit(lstCel)
print("CELSIUS\t\tFahrenheit")
sum1 = 0
sum2 = 0
for i in range(10):
sum1 = sum1 + lstCel[i]
sum2 = sum2 + lstCel[i]
x = "{:.2f}".format(lstFah[i])
print(lstCel[i], "\t\t", x)
print((sum1/10), "\t\t", (sum2/10), "\t\t<--- averages")
if __name__=="__main__":
main()
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- How to write reporting function on this question This program implements the buying and selling of stocks. It starts by printing a welcome message Welcome to mystocks.com Then a main menu of choices for the user is output. Reporting, buying or selling? (0=quit, 1=report, 2=buy, 3=sell): The program ends with a goodbye message. Thank you for trading with mystocks.com Use doubly linked list of stock_t structures. stock_t structure looks like: #define MAX_TICKER_LENGTH 6 typedef struct stock_t { char ticker[MAX_TICKER_LENGTH]; date_t date; // date bought int numShares; double pricePerShare; } stock_t; date_t structure looks like: typedef struct date_t { int month, day, year; } date_t; Create the following files in a directory: date.h: contains the above date_t structure • stock.h and stock.c: contain the stock structure and any constants and stock function prototypes. Eg: a print stock function. • node.h, node.c: contain at least the node typdef and initNode function •…arrow_forwardPython programming only NEED HELP PLEASEarrow_forwardAssume that the variable data refers to the list [5, 3, 7]. Write the expressions that perform the following tasks: a. Replace the value at position o in data with that value's negation. b. Add the value 10 to the end of data. c. Insert the value 22 at position 2 in data. d. Remove the value at position 1 in data. e. Add the values in the list newData to the end of data. f. Locate the index of the value 7 in data, safely. g. Sort the values in data.arrow_forward
- python LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forward1c) Average sentence length We will create a function ( avg_sentence_len ) to calculate the average sentence length across a piece of text. This function should take text as an input parameter. Within this function: 1. sentences : Use the split() string method to split the input text at every '.'. This will split the text into a list of sentences. Store this in the variable sentences . To keep things simple, we will consider every "." as a sentence separator. (This decision could lead to misleading answers. For example, "Hello Dr. Jacob." is actually a single sentence, but our function will consider this 2 separate sentences). 2. words : Use the split() method to split the input text into a list of separate words, storing this in words . Again, to limit complexity, we will assume that all words are separated by a single space (" "). (So, while "I am going.to see you later" actually has 7 words, since there is no space after the ".", so we will assume the this to contain 6 separate…arrow_forwardPYTHON Complete the function below, which takes two arguments: data: a list of tweets search_words: a list of search phrases The function should, for each tweet in data, check whether that tweet uses any of the words in the list search_words. If it does, we keep the tweet. If it does not, we ignore the tweet. data = ['ZOOM earnings for Q1 are up 5%', 'Subscriptions at ZOOM have risen to all-time highs, boosting sales', "Got a new Mazda, ZOOM ZOOM Y'ALL!", 'I hate getting up at 8am FOR A STUPID ZOOM MEETING', 'ZOOM execs hint at a decline in earnings following a capital expansion program'] Hint: Consider the example_function below. It takes a list of numbers in numbers and keeps only those that appear in search_numbers. def example_function(numbers, search_numbers): keep = [] for number in numbers: if number in search_numbers(): keep.append(number) return keep def search_words(data, search_words):arrow_forward
- PYTHON PLEASE Write a function that finds the smallest element in a list of float values using the following header: def min(listNum):arrow_forwardplease follow the instrcuers and use Pythonarrow_forwardCreate a list L containing at least 10 values ranging from j to k. Using a for loop, code a function that will compute and display the following list: L_out[0] = L[0] L_out[i] = L[i] + L[i-1] if i is odd and i is not equal to 0 L_out[i] = L[i] * 3 if i is even and i is not equal to 0 where L[i] represents the value stored in L at the position i. Example: L ‘(2 3 4 5) L_out ‘(2 5 12 9)arrow_forward
- In this program, your task is to calculate average of grades and then display the final grade. Your tasks are as follows: Create a function called get_average() that takes a list containing grades of five subjects and calculates the average and returns it. Create a function called get_grade() that takes average of the five subjects from the get_average() function and displays the grade as per the table below:arrow_forwardOutput values in a list below a user defined amount - functions Write a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, and output all integers less than or equal to that value. Ex: If the input is: 5 50 60 140 200 75 100 the output is: 50 60 75 The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75. Such functionality is common on sites like Amazon, where a user can filter results. Your code must define and call the following two functions:def get_user_values()def output_ints_less_than_or_equal_to_threshold(user_values, upper_threshold) Utilizing functions will help to make your main very clean and intuitive. Note: This is a lab from a previous chapter that now requires the use of functions. My…arrow_forwardprogram7.pyThis assignment requires the main function and a custom value-returning function. The value-returning function takes a list of random Fahrenheit temperatures as its only argument and returns a smaller list of only the temperatures above freezing. This value-returning function must use a list comprehension to create the list of above freezing temperatures.In the main function, code these steps in this sequence: create an empty list that will the hold random integer temperatures. use a loop to add 24 random temperatures to the list. All temperatures should be between 10 and 55, inclusive. Duplicates are okay. sort the list in ascending order. use another loop to display all 24 sorted temperatures on one line separated by spaces. 32 could be in the list. If it is, report the index of the first instance of 32. if 32 isn't in the list, add it at index 4. assign a slice consisting of the first six temperatures to a new list. Then print it. execute the custom value-returning…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
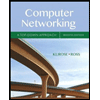
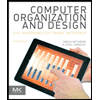
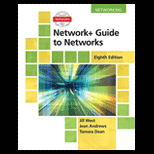
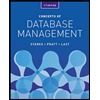
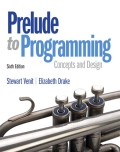
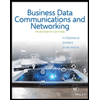