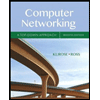
This question is in java
The Sorts.java file is the sorting program we looked at . You can use either method listed in the example when coding.
You want to add to the grades.java and calculations.java files so you put the list in numerical order. Write methods to find the median and the range.
Have a toString method that prints the ordered list, the number of items in the list, the mean, median and range.
Ex
List: 20 , 30 , 40 , 75 , 93
Number of elements: 5
Mean: 51.60
Median: 40
Range: 73
Grades.java is the main file
import java.util.Scanner;
public class grades
{
public static void main(String[] args)
{
int n;
calculation c = new calculation();
int array[] = new int[20];
System.out.print("Enter number of grades that are to be entered: ");
Scanner scan = new Scanner(System.in);
n = scan.nextInt();
System.out.println("Enter the grades: ");
for(int i=0; i<n; i++)
{
array[i] = scan.nextInt();
if(array[i] < 0)
{
System.out.println("Invalid: enter again");
i--;
}
}
System.out.println("\nThe average of your " +n+" grades is "+c.average(array, n));
}
}
calculations.java is used to find the average
import java.util.*;
class calculation
{
double average(int[] arr, int n)
{
double av;
int sum=0;
for(int i=0; i<n; i++)
{
sum = sum + arr[i];
}
av = (double)sum / (double) n;
return av;
}
}
sorts.java is an example file, in which the question asks to use either method from this code.
//********************************************************************
// Sorts.java Author: Lewis/Loftus/Cocking
//
// Demonstrates the selection sort and insertion sort algorithms,
// as well as a generic object sort.
//********************************************************************
public class Sorts
{
//-----------------------------------------------------------------
// Sorts the specified array of integers using the selection
// sort
//-----------------------------------------------------------------
public static void selectionSort (int[] numbers)
{
int min, temp;
for (int index = 0; index < numbers.length-1; index++)
{
min = index;
for (int scan = index+1; scan < numbers.length; scan++)
if (numbers[scan] < numbers[min])
min = scan;
// Swap the values
temp = numbers[min];
numbers[min] = numbers[index];
numbers[index] = temp;
}
}
//-----------------------------------------------------------------
// Sorts the specified array of integers using the insertion
// sort algorithm.
//-----------------------------------------------------------------
public static void insertionSort (int[] numbers)
{
for (int index = 1; index < numbers.length; index++)
{
int key = numbers[index];
int position = index;
// shift larger values to the right
while (position > 0 && numbers[position-1] > key)
{
numbers[position] = numbers[position-1];
position--;
}
numbers[position] = key;
}
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 3 images

- In java i have some code for a method that finds the index of an integer that is passed in thet int is (target) the method also has the array called (array) and the first and last element of the array. also for this method to work the array has to be in descending order "static int find(int [] array, int first, int last, int target)" thats the method with all the variables that are passed to it my guess is that the first if statement just will have a short line that is "return -1;" because since the array has to be in descending order the first element cant be greater then the last element in the array but im confused on the rest because i cant find a way to make them one line codes with the conditions that i have to meet in the comments any help would be aprreaciatedarrow_forwardhow would you do this in a simple way? this is a non graded practice labarrow_forwardI need help with this code in Javaarrow_forward
- Given a long string use recursion to traverse the string and replace every vowel (A,E,I,O,U) with an @ sign. You cannot change the method header. public String encodeVowels(String s){ // Your code here }arrow_forwardPlease answer in python Write a method called add_racer which takes in a Boat object and adds it to the end of the racers list. The function does not return anything. Write a method called print_racers which loops through racers and prints the Boat objects. This function takes in no parameters (other than self) and returns nothing. Write a method called count that returns the number of racers. Write a method called race. The race function calls the move function for all of the racers in the BoatRace. Once all the racers have moved, call the print_racers method to display information about the progress of each boat. Then, check if any of the racer’s current_progress is greater than or equal to the race’s distance. If so, then return a list of all of the racers whose current_progress is greater than or equal to distance. If no racer has finished the race then repeat the calls to move and check until at least one racer has finished the race. Examples: Copy the following if…arrow_forwardJAVAarrow_forward
- Please write a java code using the photo below. The second photo is the output of my program that I am writingarrow_forward4. Now examine the ArrayList methods in the above table, a) Which method retrieves elements from the ArrayList? b) Which method replaces the value of an element that already exists in the ArrayList? c) Which two methods initializes the value of an element? d) How do the two methods in (c) differ? Which method(s) would be appropriate in the above Java program (after we convert it to work with ArrayLists)?arrow_forwardI need the code from start to end with no errors and the explanation for the code ObjectivesJava refresher (including file I/O)Use recursionDescriptionFor this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA7 9# # ###### # # ## # # #### # ## ##### ## ########## RequirementsWrite a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the valid exits found in any order. See the examples below for exact output requirements. Also, record…arrow_forward
- Needs to be written in java and completed using "for loops" or "nested for loops" and in one program just seperate methods: Write a program that calls these three methods. method 1 should print: 5 4 3 2 1 4 3 2 1 3 2 1 2 1 1 method 2 should print: 1 2 3 4 5 1 2 3 4 1 2 3 1 2 1 method 3 should create and array and store 10 multiples of 5 and print it out. Use for loop to generate the multiples and then print them out using a second for loop.arrow_forwarduse java to create a card game with the implements listed for the program. If you are able to do the first couple of steps that would be helpful and for step one use an array list with the following code. The code below should create 52 cards. String[] suits = {"Hearts", "Clubs", "Spades", "Diamonds"}; String[] numbers = {"A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K"}; for(String oneSuit : suits){ for(String num : numbers){ System.out.println(oneSuit + " " + num); } }arrow_forwardSorting an array of batting averages would be useful only if you sort another array in the same sequence in Java. Explain.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
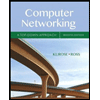
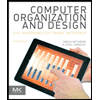
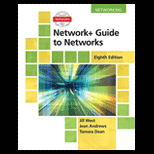
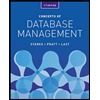
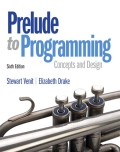
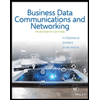