This program determines if an airline passenger is // eligible for a 25% discount.
This program determines if an airline passenger is // eligible for a 25% discount.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
// Airline.cpp - This program determines if an airline passenger is
// eligible for a 25% discount.
// Harvey Mark, ITSE 1329#include <iostream>
#include <string>
using namespace std;
int main()
{
string passengerFirstName = ""; // Passenger's first name
string passengerLastName = ""; // Passenger's last name
int passengerAge = 0; // Passenger's age
// This is the work done in the housekeeping() function
cout << "Enter passenger's first name: ";
cin >> passengerFirstName;
cout << "Enter passenger's last name: ";
cin >> passengerLastName;
cout << "Enter passenger's age: ";
cin >> passengerAge;
// This is the work done in the detailLoop() function
// Test to see if this customer is eligible for a 25% discount
if(passengerAge <= 6)
{
cout << "You are eligable for a discount!\n";
}
if(passengerAge >= 65)
{
cout << "You are eligable for a discount!\n";
}
if(passengerAge >= 7 && passengerAge <= 64)
{
cout << "You are not eligable for any discounts.\n";
}
// This is the work done in the endOfJob() function
return 0;
}
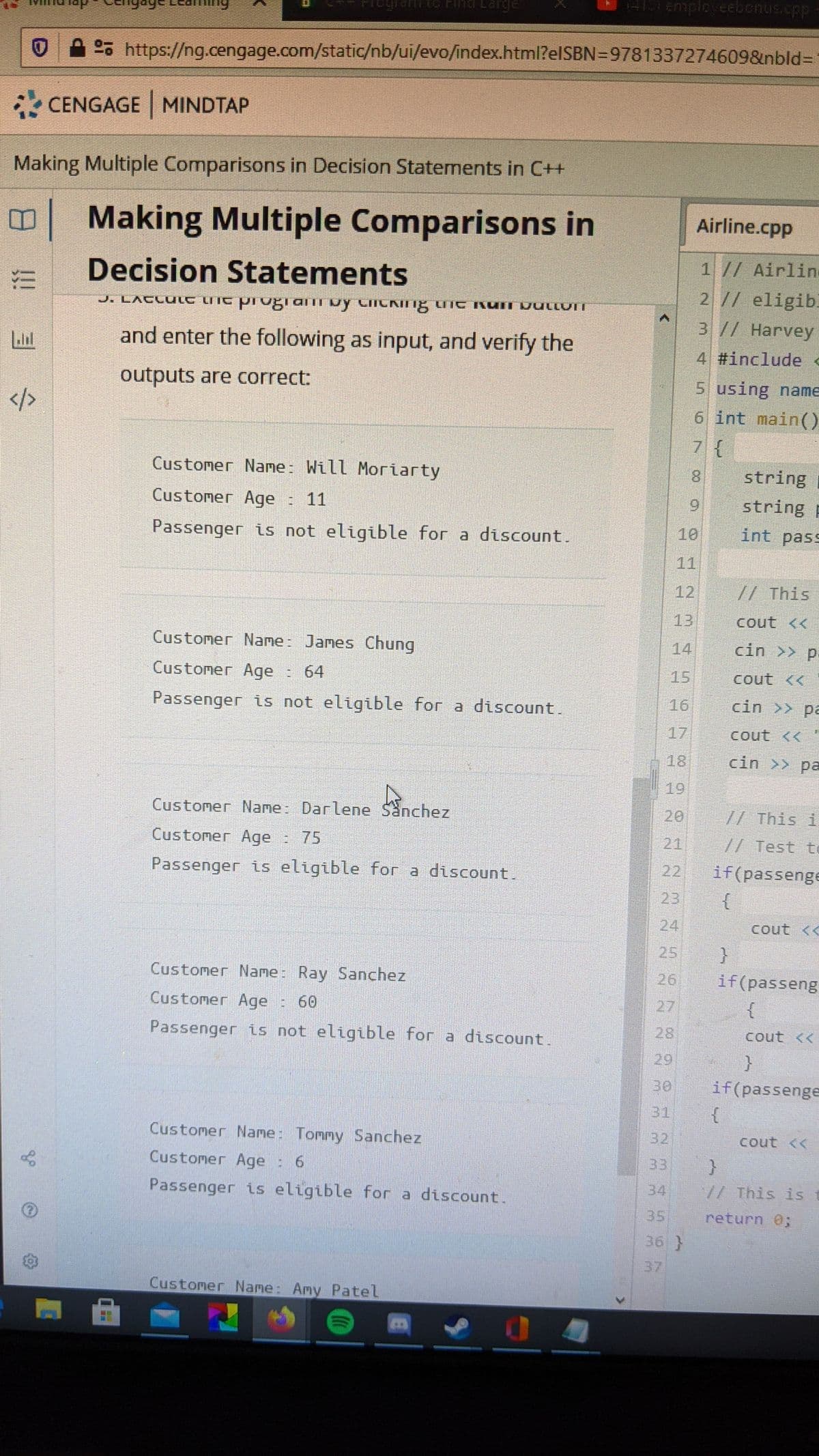
Transcribed Image Text:FiegrantG Find Lare
o https://ng.cengage.com/static/nb/ui/evo/index.html?elSBN=9781337274609&nbld%3D
* CENGAGE MINDTAP
Making Multiple Comparisons in Decision Statements in C++
Making Multiple Comparisons in
Airline.cpp
1// Airlin
Decision Statements
2 1/ eligib.
J. LACLULC UIC Pi Ugiaii by CIICKING UIC KUII JULLUIT
3 // Harvey
and enter the following as input, and verify the
4 #include c
outputs are correct:
5 using name
6 int main()
7 {
Customer Name: Will Moriarty
8.
string
Customer Age : 11
96.
string p
Passenger is not eligible for a discount.
10
int pass
11
12
//This
13
cout <<
Customer Name: James Chung
14
cin >> p
Customer Age
E 64
15
cout <<
Passenger is not eligible for a discount.
16
cin >> pa
17
cout <<"
18
cin >> pa
19
Customer Name: Darlene Sanchez
20
7/ This i
Customer Age : 75
21
// Test t
if(passenge
{
Passenger is eligible for a discount.
22
23
24
cout <<
25
Customer Name: Ray Sanchez
26
if(passeng
Customer Age
e: 60
27
Passenger is not eligible for a discount.
28
cout <<
29
30
if(passenge
31
Customer Name: Tommy Sanchez
32
cout <<
Customer Age : 6
33
Passenger is eligible for a discount.
34
/ This is t
35
return 0;
36 }
37
Customer Name: Amy Patel
!!
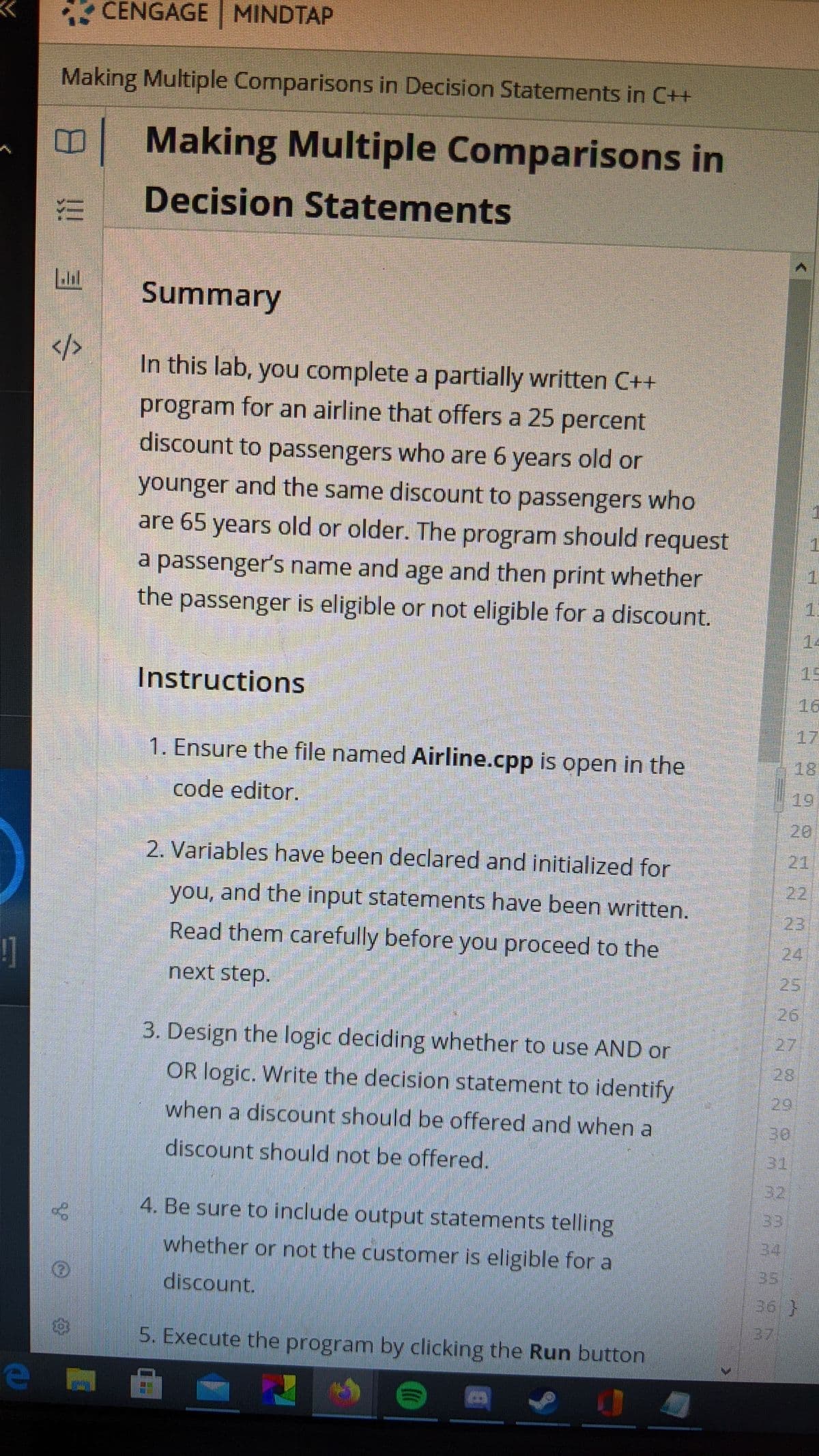
Transcribed Image Text:* CENGAGE MINDTAP
Making Multiple Comparisons in Decision Statements in C++
BMaking Multiple Comparisons in
Decision Statements
Summary
</>
In this lab, you complete a partially written C++
program for an airline that offers a 25 percent
discount to passengers who are 6 years old or
younger and the same discount to passengers who
are 65 years old or older. The program should request
1.
a passenger's name and age and then print whether
the passenger is eligible or not eligible for a discount.
1.
14
Instructions
15
16
1. Ensure the file named Airline.cpp is open in the
17
18
code editor.
19
20
2. Variables have been declared and initialized for
211
you, and the input statements have been written.
22
Read them carefully before you proceed to the
23
24
next step.
25
26
3. Design the logic deciding whether to use AND or
27
OR logic. Write the decision statement to identify
28
when a discount should be offered and when a
29
30
discount should not be offered.
31
32
4. Be sure to include output statements telling
33
whether or not the customer is eligible for a
34
discount.
35
36 }
5. Execute the program by clicking the Run button
!!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
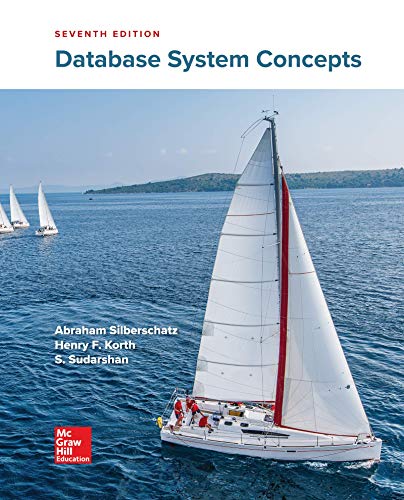
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
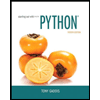
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
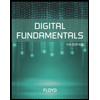
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
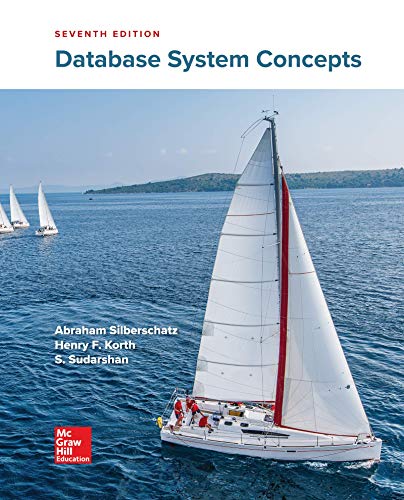
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
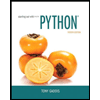
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
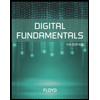
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
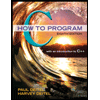
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
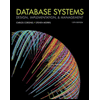
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
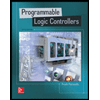
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education