To understand the value of recursion in a programming language, write a program that implements quicksort, first using recursion and then without recursion
To understand the value of recursion in a

First ,some fundamentals about quicksort,
1. It is similar to merge sort algorithm in which the array is being partitioned with respect to a pivot element
and the sorted recursively to get the final result.
2. I have used the function quickSort to recursively sort ,then I have used the partition function to implement the pivot concept ,then inbuilt swap function of C++.
3. I have given description on each side of code to get the idea.
#include <bits/stdc++.h>
using namespace std;
//this function takes last element as pivot element and keep it at its correct position in sorted array,then after this it places all smaller eleemnts to the left of pivot and greater to right of pivot
int partition (int arr[], int l, int h)
{
int pivot = arr[h]; // pivot
int i = (l - 1); // to get current right position of pivot
for (int j = l; j <= h- 1; j++)
{
// If current element is smaller than the pivot
if (arr[j] < pivot)
{
i++;
swap(arr[i], arr[j]);//inbuilt swap function of C++
}
}
swap(arr[i + 1], arr[h]);//inbuilt swap function of C++
return (i + 1);
}
void quickSort(int arr[], int l, int h)
{
if (l < h)
{
//p is partitioning index from where the array will be divided into two parts
int p = partition(arr, l, h);
// Separately sort elements before partition
quickSort(arr, l, p - 1);
quickSort(arr, p + 1, h);// sort after partition
}
}
//to print the array
void printArray(int arr[], int n)
{
for (int i = 0; i < n; i++)
{
cout << arr[i] << " ";
}
cout << endl;
}
int main()
{
int arr[] = {10, 7, 8, 9, 1, 5};//example to make it clear
int n = 6;
quickSort(arr, 0, 5);
cout << "Quick Sort Implementation recursively : Following is the sorted array \n";
printArray(arr, n);
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

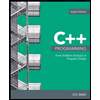
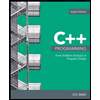