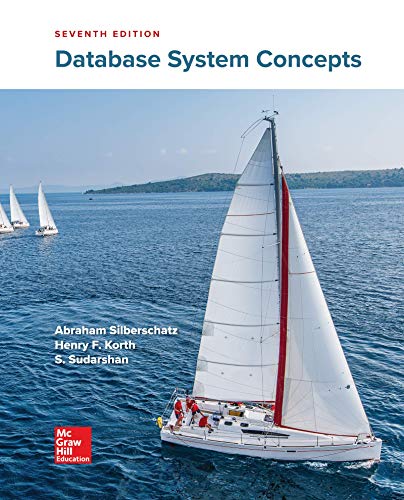
TOPICS: Using Classes and Objects
MUST BE IN JAVA. PLEASE USE COMMENTS AND WRITE THE CODE IN SIMPLEST FORM.
3. Write an application that reads the radius of a sphere then calculates and displays the circumference, volume and surface area. Use the following formulas, in which r represents the sphere’s radius. Print the output to four decimal places.
Circumference = 2 π r
Volume = 4/3 π r3
Surface Area = 4 π r2
Hint: Section 3.5 of the textbook describes the many methods of the Math class and how they are used. The Math class also contains pre-defined constants like π. Search on the web for how you can use these.
Testing: Include test exhibits for inputs that you choose. Make sure you check the results either with a calculator or some other independent source. Hint: Try Google!

Step by stepSolved in 3 steps with 2 images

- Java Questions - (Has 2 Parts). Based on each code, which answer out of the choices "A,B,C,D,E" is correct. Each question has one correct answer. Thank you. Part 1 - 9. Given the following code, the output is the date and time of __.Calendar cal = Calendar.getInstance(); TimeZone tz = TimeZone.getTimeZone("Europe/Rome");TimeZone tz = TimeZone.getTimeZone("America/Los_Angeles");TimeZone tz = TimeZone.getTimeZone("Asia/Istanbul");System.out.println(cal.getTime()); A. the "Europe/Rome" time zone.B. the time zone is computer is currently set to use.C. the "America/Los_Angeles" time zone.D. the "Asia/Istanbul" time zone.E. All of the three listed time zones. Part 2 - 10. Given the following code, the output is the date and time of __. Calendar cal = Calendar.getInstance();TimeZone tz = TimeZone.getTimeZone("Europe/Rome");cal.setTimeZone(TimeZone.getTimeZone("America/Los_Angeles"));TimeZone tz = TimeZone.getTimeZone("Asia/Istanbul");System.out.println(cal.getTime()); A. the "Europe/Rome"…arrow_forwardThe game of Nim starts with a random number of stones between 15 and 30. Two players alternate turns and on each turn may take either 1, 2, or 3 stones from the pile. The player forced to take the last stone loses. Create a nim application in python that allows the user to play against the computer. In this version of the game, the application generates the number of stones to begin with, the number of stones the computer takes, and the user goes first. The Nim application code should: prevent the user and the computer from taking an illegal number of stones. For example, neither should be allowed to take three stones when there are only 1 or 2 left. include an is_valid_entry() function to check user input. include a draw_stones() function that generates a random number from 1 to 3 for the number of stones the computer draws. include separate functions to handle the user’s turn and the computer’s turn. My problem is that the code is not outputting like it's supposed to.…arrow_forwarddef area(side1, side2): return side1 * side2s1 = 12s2 = 6Identify the statements that correctly call the area function. Select ALL that apply. Question options: area(s1,s2) answer = area(s1,s2) print(f'The area is {area(s1,s2)}') result = area(side1,side2)arrow_forward
- The Freemont Automobile Factory has discovered that the longer a worker has been on the job, the more parts the worker can produce. Write an application that computes and displays a worker’s anticipated output each month for 24 months assuming the worker starts by producing 4,000 parts and increases production by 6 percent each month. Also display the month in which production exceeds 7,000 parts (when the worker deserves a raise!) as follows: The month in which production exceeds 7000.0 is month X. This is what i have but isnt right public class IncreasedProduction { publicstaticvoidmain (String[] args) { // final int month = 24; double parts =4000.0; double production =0.06; double raise =7000.0; int counter; System.out.println("The month in which production exceeds 7000.0 is month " + month); counter = 1; while(counter >= parts) { System.out.print(counter + " "); counter = counter + 1; } System.out.println(); } }arrow_forwardnumber = input() while number!=id: print("This is not your ID number.") number = input() print("This is your ID number:",number) Need to have a space between This is your ID number and number. How shall I do that?arrow_forwardJava Questions - (Has 2 Parts). Based on each code, which answer out of the choices "A, B, C, D, E" is correct. Each question has one correct answer. Thank you. Part 1 - Given the following code, what is the height of "label1"? label1.setBounds(10, 15, 200, 30); A. 10B. 15C. 200D. 30E. Not specified Part 2 - Given the following code, which can add "label1" to a JPanel named "panel1"? JLabel label1 = new JLabel("Hello World !"); A. panel1.Controls.add(label1);B. panel1.add(label1);C. panel1.add("label1");D. panel1.add.Controls(label1);E. label1.add(panel1);arrow_forward
- Assume that the population of Mexico is 128 million and that the population increases 1.01 percent annually. Assume that the population of the United States is 323 million and that the population is reduced 0.15 percent annually. Write an application that displays the populations for the two countries every year until the population of Mexico exceeds that of the United States, and display the number of years it took. Save the file as Population.java.arrow_forwardScientists measure an objects mass in Kilograms and its weight in newtons. If you know the mass of an object, you can calculate its weight in newtons, with the following formula weight = mass X 9.8 . Design a program using pseudocode and comments that asks the user to enter an objects mass, and then calculates its weight. If the object weighs more than 1000 newtons display a message indicating that it is too heavy. If the object weighs less than 10 newtons, display a message saying it is to light. Here are some necessary parameters to follow to help solve the problem.a. Create a global constant for the multiplier b. Create a main module. It should declare two local variables and call three other modules c. Create a module to get the input from the software user d. Create a module to calculate the weight e. Create a module to display the answer. //Please write line numbers before each line of the algorithm, include blank numbered lines between modules and write commentsarrow_forwardAssume that the population of Mexico is 128 million and the population of the United States is 323 million. Write a program called Population that accepts two values from a user: an assumption of an annual increase in the population of Mexico and an assumption for an annual decrease in the U.S. population. Accept both figures as percentages; in other words, a 1.5 percent decrease is entered as 0.015. Write an application that displays the populations of the two countries every year until the population of Mexico exceeds that of the United States, and display the number of years it took. An example of the program is shown below: Enter the percent annual increase for Mexico population Enter as a decimal. For example, 0.5% is entered as 0.005 Enter the value >> 0.008 Enter the percent annual decrease for U.S. population Enter as a decimal. For example, 0.5% is entered as 0.005 Enter the value >> 0.002 Mexico population U.S. Population 1 129.024 million 322.354 million 2…arrow_forward
- John the farmer has tasked his son with collecting the eggs from the chicken coop each morning and putting them in boxes of 1 dozen eggs each. Unfortunately, John’s son Joe is having trouble working out how many boxes he will need each morning. You have been asked to develop a java application to allow Joe to enter the number of eggs he has collected. The application should then calculate and output how many boxes can be filled and how many eggs Joe will have leftover. Your application should use instantiable classes to separate the calculations from the user input and output.arrow_forwardobject oriented programming need the full code as soon as possible.arrow_forwardPython GUI tkinter: Write a program where the GUI display is My favorite soda is Coca-Cola. Whe the user clicks on soda, they have these following options in a menu: beverage drink refresher When the user clicks on Coca-Cola, the following are listed as menu options: Pepsi Mountain Dew Once a selection is made, the entire sentence changes. For example, the user selects beverage and Pepsi: My favorite beverage is Pepsi.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
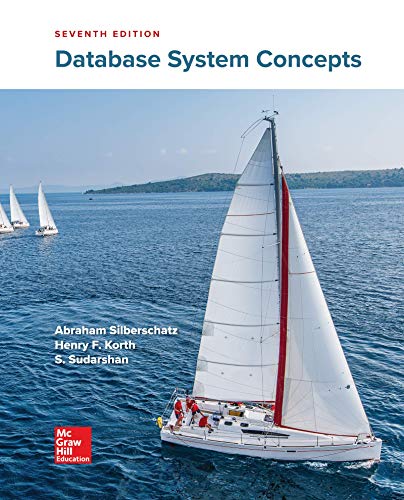
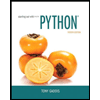
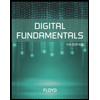
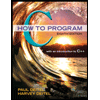
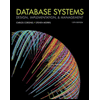
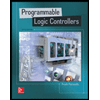