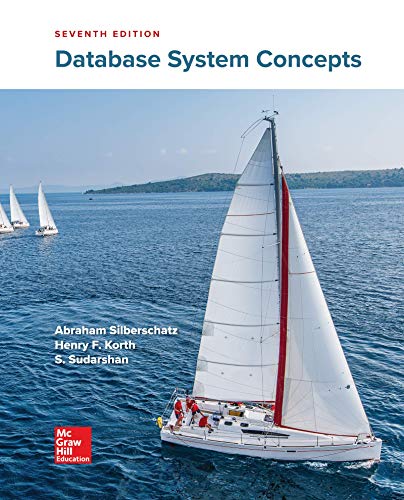
Concept explainers
Translate the following C++ program into assembly language:
#include <iostream>
using namespace std;
int main() {
char letter;
int countA = 0,
countB = 0,
countC = 0;
cin >> letter;
do {
switch (letter) {
case 'A' : countA++; break;
case 'B' : countB++; break;
case 'C' : countC++; break;
}
cin >> letter;
} while (letter != 'X');
cout << "Number of A's " << countA << endl
<< "Number of B's " << countB << endl
<< "Number of C's " << countC << endl;
return 0;
}
Use local variables (except for messages, of course) and the branch indexed for the switch statement. Submit via Moodle.
MUST BE ASSEMBLY LANGAUGE FOR PEP/9
COMMENTS RECOMMENDED

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- c++arrow_forwardConvert the following c++ code into pep9 assembly language. #include <iostream> using namespace std; void times(int& prod, int mpr, int mcand) { prod = 0; while (mpr != 0) { if (mpr % 2 == 1) prod = prod + mcand; mpr /= 2; mcand *= 2; } } int main(){ int product, n, m; cout << "Enter two numbers: "; cin >> n >> m; times(product, n, m); cout << "Product: " << product << endl; return 0; }arrow_forwardConvert the following c++ code into pep9 assembly language #include <iostream> using namespace std; void times(int& prod, int mpr, int mcand) { prod = 0; while (mpr != 0) { if (mpr % 2 == 1) prod = prod + mcand; mpr /= 2; mcand *= 2; } } int main(){ int product, n, m; cout << "Enter two numbers: "; cin >> n >> m; times(product, n, m); cout << "Product: " << product << endl; return 0; }arrow_forward
- Convert the following C++ programs into Pep/9 assembly #include <iostream> using namespace std; int minimum (int i1, int i2){if (i1 < i2)return i1;elsereturn i2;}int main (){int n, m;cin >> n >> m;cout << "Minimum: " << minimum (n, m) << endl;return 0;} Submit: Pep/9 source code along with screen capture showing it running in the Pep simulatorarrow_forwardtranslate the following C++ program into Pep/9 Assembly. #include<iostream> using namespace std; void showNext(int age){ int nextYr; nextYr = age + 1; cout << "Age:" << age<< endl; cout << "Age next year: " << nextYr << endl;}int main() { int myAge; cout << "Enter age: "; cin >> myAge; showNext (myAge); return 0;}arrow_forwardPEP/9 Translate the following C++ program into assembly language: #include <iostream> using namespace std; int main() { char letter; int countA = 0, countB = 0, countC = 0; cin >> letter; do { switch (letter) { case 'A' : countA++; break; case 'B' : countB++; break; case 'C' : countC++; break; } cin >> letter; } while (letter != 'X'); cout << "Number of A's " << countA << endl << "Number of B's " << countB << endl << "Number of C's " << countC << endl; return 0; } Use local variables (except for messages, of course) and the branch indexed for the switch statement.arrow_forward
- Convert the following C++ programs into Pep/9 assembly #include <iostream> using namespace std; void minimum (int i1, int i2){ if (i1 < i2) cout << i1 << " is less than " << i2 << endl; else if (i1 > i2) cout << i2 << " is less than " << i1 << endl; else cout << i1 << " equals " << i2 << endl;}int main (){ int n, m; cout << "Enter two integers: "; cin >> n >> m; minimum (n, m); return 0;} Submit: Pep/9 source code along with screen capture showing it running in the Pep simulatorarrow_forwardConvert the following C++ programs into Pep/9 assembly #include <iostrea> using namespace std; int minimum (int i1, int i2){ if (i1 < i2) cout << i1 << " is less than " << i2 << endl; else if (i2 < i1) cout << i2 << " is less than " << i1 << endl; else cout << i1 << " equals " << i2 << endl;}int main (){int n, m;cin >> n >> m;cout << "Minimum: " << minimum (n, m) << endl;return 0;} Submit: Pep/9 source code along with screen capture showing it running in the Pep simulatorarrow_forwardFind the terms az through a, of the sequence defined by the recurrence relationship an = 3an-1 – 2a-2 + an-3 and the initial conditions a, = 4, a, = 1, and az = 3.arrow_forward
- Complete the following C++ programs into Pep/9 assembly language: 1) int main(){int cop; int driver; cop = 0; driver = 40; while (cop <= driver) { cop += 25; driver += 20; } cout << cop; return 0;}arrow_forwardtranslate a c++ program into pep/9 assembly language #include <iostream> using namespace std; int myAge;void ShowVal(int age){ cout << "Age:" << age << endl;} int main() {cout << "Enter age: ";cin >> myAge;ShowVal(myAge);return 0;}arrow_forwardI need this code to be with python 3 1. Approach: Non recursive: //include necessary header files#include <iostream>using namespace std;//main functionint main(){ int days,buy_on_this_day ,sell_on_this_day; //get number of days as input from user cout<<"Enter number of days: "; cin>>days; int stock_price[days]; for(int i=0;i<days;i++) { cout<<"Enter stock_price"; cin>>stock_price[i]; } int i=0; for(int i=0;i<days-1;i++) { //comparing current price with next day price and finding the minima while(i<days-1 && stock_price[i+1]<=stock_price[i]) i++; if(i==days-1) break; buy_on_this_day =i++; while(i<days && stock_price[i]>= stock_price[i-1]) i++; sell_on_this_day =i-1; cout<<buy_on_this_day <<" : index of the change before we buy"<<endl; cout<<sell_on_this_day<<" :index…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
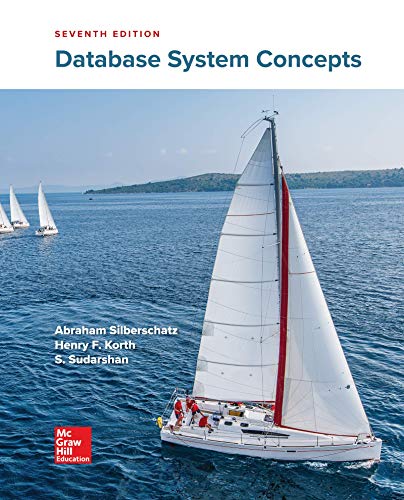
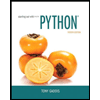
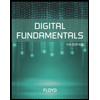
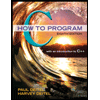
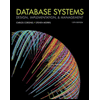
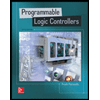