UML diagram for the java code below public class CourseDBManager implements CourseDBManagerInterface { private CourseDBStructure dataMgr = new CourseDBStructure(20); public void readFile(File inputFile) throws FileNotFoundException{ try { Scanner fileData = new Scanner(inputFile); while(fileData.hasNext()) { String id = fileData.next(); int crn = fileData.nextInt(); int numCredits = fileData.nextInt(); String roomNum = fileData.next(); String instructor = fileData.nextLine(); add(id, crn, numCredits, roomNum, instructor); } fileData.close(); } catch(FileNotFoundException e) { System.out.print("File not found"); e.getMessage(); } } public void add(String id, int crn, int credits, String roomNum, String instructor) { CourseDBElement newElement = new CourseDBElement(id, crn, credits, roomNum, instructor); dataMgr.add(newElement); } public ArrayList showAll() { ArrayList list = new ArrayList(); for(int i = 0; i < dataMgr.getTableSize(); i++) { LinkedList tempList = dataMgr.hashTable[i]; if(tempList != null) { for(int j = 0; j < tempList.size(); j++) { CourseDBElement element = tempList.get(j); list.add(element.toString()); } } } return list; } public CourseDBElement get(int crn) throws IOException { try { return dataMgr.get(crn); } catch (IOException e) { e.getMessage(); } return null; } }
UML diagram for the java code below public class CourseDBManager implements CourseDBManagerInterface { private CourseDBStructure dataMgr = new CourseDBStructure(20); public void readFile(File inputFile) throws FileNotFoundException{ try { Scanner fileData = new Scanner(inputFile); while(fileData.hasNext()) { String id = fileData.next(); int crn = fileData.nextInt(); int numCredits = fileData.nextInt(); String roomNum = fileData.next(); String instructor = fileData.nextLine(); add(id, crn, numCredits, roomNum, instructor); } fileData.close(); } catch(FileNotFoundException e) { System.out.print("File not found"); e.getMessage(); } } public void add(String id, int crn, int credits, String roomNum, String instructor) { CourseDBElement newElement = new CourseDBElement(id, crn, credits, roomNum, instructor); dataMgr.add(newElement); } public ArrayList showAll() { ArrayList list = new ArrayList(); for(int i = 0; i < dataMgr.getTableSize(); i++) { LinkedList tempList = dataMgr.hashTable[i]; if(tempList != null) { for(int j = 0; j < tempList.size(); j++) { CourseDBElement element = tempList.get(j); list.add(element.toString()); } } } return list; } public CourseDBElement get(int crn) throws IOException { try { return dataMgr.get(crn); } catch (IOException e) { e.getMessage(); } return null; } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
UML diagram for the java code below
public class CourseDBManager implements CourseDBManagerInterface { | |
private CourseDBStructure dataMgr = new CourseDBStructure(20); | |
public void readFile(File inputFile) throws FileNotFoundException{ | |
try { | |
Scanner fileData = new Scanner(inputFile); | |
while(fileData.hasNext()) { | |
String id = fileData.next(); | |
int crn = fileData.nextInt(); | |
int numCredits = fileData.nextInt(); | |
String roomNum = fileData.next(); | |
String instructor = fileData.nextLine(); | |
add(id, crn, numCredits, roomNum, instructor); | |
} | |
fileData.close(); | |
} | |
catch(FileNotFoundException e) { | |
System.out.print("File not found"); | |
e.getMessage(); | |
} | |
} | |
public void add(String id, int crn, int credits, String roomNum, String instructor) { | |
CourseDBElement newElement = new CourseDBElement(id, crn, credits, roomNum, instructor); | |
dataMgr.add(newElement); | |
} | |
public ArrayList<String> showAll() { | |
ArrayList<String> list = new ArrayList<String>(); | |
for(int i = 0; i < dataMgr.getTableSize(); i++) { | |
LinkedList<CourseDBElement> tempList = dataMgr.hashTable[i]; | |
if(tempList != null) { | |
for(int j = 0; j < tempList.size(); j++) { | |
CourseDBElement element = tempList.get(j); | |
list.add(element.toString()); | |
} | |
} | |
} | |
return list; | |
} | |
public CourseDBElement get(int crn) throws IOException { | |
try { | |
return dataMgr.get(crn); | |
} catch (IOException e) { | |
e.getMessage(); | |
} | |
return null; | |
} | |
} |
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
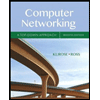
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
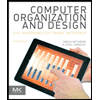
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
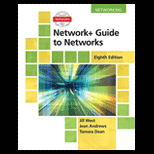
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
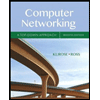
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
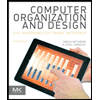
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
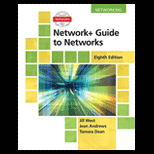
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
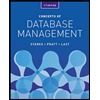
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
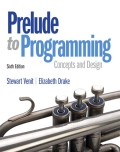
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
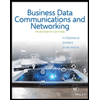
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY