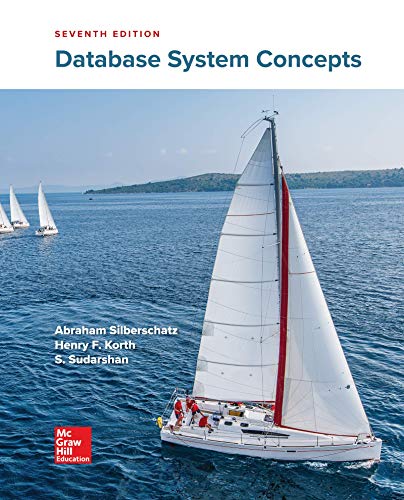
Use C++
Using dynamic arrays, implement a polynomial class with polynomial addition, subtraction, and multiplication.
Implement addition and subtraction functions first.
it should have five files
One is the main.cpp file
One is the Polynomial.cpp file it'sit's in the picture
One is the Polynomial.h file
One is A.txt
One is B.txt
The contents of A and B.txt are in the picture
Here is the main.cpp code I have:
#include <iostream>
#include <fstream>
#include "polynomial.h"
using namespace std;
int main()
{
// read data from A.txt
ifstream infile;
infile.open("A.txt");
if (infile.fail()) {
cout << "Input file opening failed." << endl;
exit(1);
}
int degree_A;
infile >> degree_A;
int* coef_A = new int[degree_A+1];
for (int i = 0; i < degree_A + 1; i++)
coef_A[i] = 0;
int coef, exp;
while (!infile.eof())
{
infile >> coef >> exp;
coef_A[exp] = coef;
}
infile.close();
// read data from B.txt
infile.open("B.txt");
if (infile.fail()) {
cout << "Input file opening failed." << endl;
exit(1);
}
int degree_B;
infile >> degree_B;
int* coef_B = new int[degree_B + 1];
for (int i = 0; i < degree_B + 1; i++)
coef_B[i] = 0;
while (!infile.eof())
{
infile >> coef >> exp;
coef_B[exp] = coef;
}
infile.close();
Polynomial p1(coef_A, degree_A);
Polynomial p2(coef_B, degree_B);
Polynomial p3 = add(p1, p2);
Polynomial p4 = subtract(p1, p2);
// Print out p1, p2, p3
return 0;
}
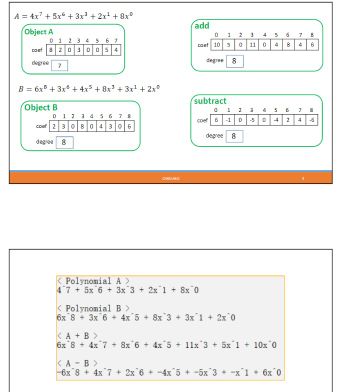
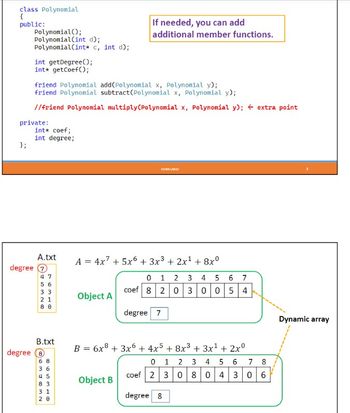

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Java Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forwardCounting the character occurrences in a file For this task you are asked to write a program that will open a file called "story.txt" and count the number of occurrences of each letter from the alphabet in this file. At the end your program will output the following report: Number of occurrences for the alphabets: a was used – times. b was used – times. c was used – times.... .and so, on Assume the file contains only lower-case letters and for simplicity just a single paragraph. Your program should keep a counter associated with each letter of the alphabet (26 counters) [Hint: Use array] Your program should also print a histogram of characters count by adding a new function print Histogram (int counters []). This function receives the counters from the previous task and instead of printing the number of times each character was used, prints a histogram of the counters. An example histogram for three letters is shown below) [Hint: Use the extended asci character 254]: A…arrow_forwardC++ I cannot run the code. Please help me fix it. Below is the requirement of the code. Design and write a C++ class that reads text, binary and csv files. The class functions: Size: Returns the file sizeName: Returns the file nameRaw: Returns the unparsed raw data (use vector here to get the unparsed raw data)Parse: A external function to Parse the data. The function accepts the raw data and returns the data parsed by the function. This type of function is called a "Call-Back Function". A Call-Back is necessary for each file as each file requires different regular expressions to parse. With binary files, need to use RegEx to do the parse(). [My code doesn't have this part] Please refer to the Callback.cpp example on how to setup a Call-Back. CallBack.cpp: #include <string>#include <functional>#include <iostream>#include <vector>using namespace std; string ToLower(string s){ string temp; for (char c : s)…arrow_forward
- JAVA PPROGRAM Write a program that prompts the user to enter a file name, then opens the file in text mode and reads names. The file contains one name on each line. The program then compares each name with the name that is at the end of the file in a symmetrical position. For example if the file contains 10 names, the name #1 is compared with name #10, name #2 is compared with name #9, and so on. If you find matches you should print the name and the line numbers where the match was found. While entering the file name, the program should allow the user to type quit to exit the program. If the file with a given name does not exist, then display a message and allow the user to re-enter the file name. The file may contain up to 100 names. You can use an array or ArrayList object of your choosing, however you can only have one array or ArrayList. Input validation: a) If the file does not exist, then you should display a message "File 'somefile.txt' is not found." and allow the…arrow_forwardCreate a C++ Program to do the following: Create a .txt file with up to 10 double numbers. Then in your code, create an array to hold 10 doubles. Openthe file. Read each number from the file into a place in the array. Count how many numbers you read. Close thefile.Now, go through the data in the array twice. Don’t go through all 10 elements in the array, only the elementsfor which you have read data. On the first pass, calculate the average of the numbers in the array. (Calculate thetotal, divide by count, that is µ).On the second pass through the data, for each number you should calculate how far it is from the average(number - average), square that, and keep a sum of the squares, that is S.When you’re done, calculate the average of that sum of squares, A. The square root of that number, σ is yourstandard deviation.Print out the average µ and standard deviation σarrow_forwardUse C++ In a student file, there are names and scores. Find the max score and print out the names of the students with the max score. • You must get a filename as standard input. See two sample outputs on the next page. • The number of students in the file is at most 50. • You need to make two arrays (see the next page). 1. Create a string array with size 50. The array will store the names of the students. 2. Create an integer array with a size of 50. The array will store the scores of the students.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
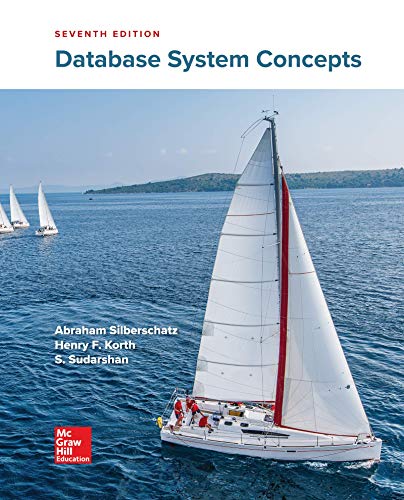
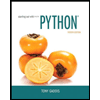
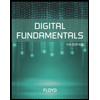
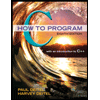
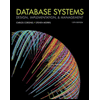
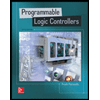