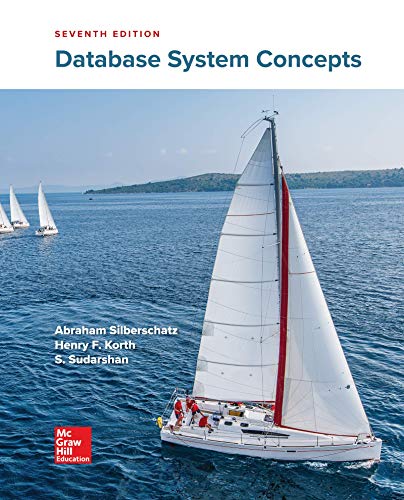
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Use Python for this question:
Implement a function eligible that returns a list of the names of people that are eligible for
vaccination, subject to a minimum age requirement. The function eligible must:
- accept two arguments:
- the minimum age for eligibility, a positive integer
- a multiline string, containing the people waiting to be vaccinated. Each line of the string
- contains a person's name and their age, separated by a single space.
- return a list of the people who are eligible to be vaccinated, i.e., those whose ages is at
- least that of the minimum age for eligibility. They should be listed in the order they occur in
- the input string
Output for this question is in the attached picture
![>>> people = 'sSue 25\nali 45\nsyd 45\nSally 105\nIan 16\nuli 65\noliver 23\nzoe
95'
>>> print (people)
Sue 25
Ali 45
Syd 45
Sally 105
Ian 16
uli 65
oliver 23
Zoe 95
>>> eligible(80, people) # who is at least 80?
['sally', 'zoe']
>>> eligible(45, people)
['Ali', 'syd', 'sally', 'uli', 'zoe']
>>> eligible(0, people)
['sue', 'Ali', 'Syd', 'sally', 'Ian', 'uli', 'oliver', 'zoe']
>>> eligible(40, 'Bob 25\nali 45\nNancy 45\nSally 105')==['Ali', 'Nancy',
'sally']
True](https://content.bartleby.com/qna-images/question/880d92ec-59f4-4b93-ae47-aeb3a2764c60/23c5ce0b-309d-4584-b591-267b7d6f8a79/h932epn_thumbnail.png)
Transcribed Image Text:>>> people = 'sSue 25\nali 45\nsyd 45\nSally 105\nIan 16\nuli 65\noliver 23\nzoe
95'
>>> print (people)
Sue 25
Ali 45
Syd 45
Sally 105
Ian 16
uli 65
oliver 23
Zoe 95
>>> eligible(80, people) # who is at least 80?
['sally', 'zoe']
>>> eligible(45, people)
['Ali', 'syd', 'sally', 'uli', 'zoe']
>>> eligible(0, people)
['sue', 'Ali', 'Syd', 'sally', 'Ian', 'uli', 'oliver', 'zoe']
>>> eligible(40, 'Bob 25\nali 45\nNancy 45\nSally 105')==['Ali', 'Nancy',
'sally']
True
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In python, write a function that receives three parameters: name, weight, and height. The default value for name is James. The function calculates the BMI and returns it. BMI is: weight/(height^2). Weight should be in kg and height should be in meters. For instance, if the weight is 60 kg and the height is 1.7 m, then the BMI should be 20.76. The function should print the name and BMI. The function should return 'BMI is greater than 22' if the MBI is greater than or equal to 22. Otherwise, the function should return 'BMI is less than 22'. Call the function and print its output.arrow_forwardCould you help me create a python code for this question? Thanks.arrow_forwardJava A jiffy is the scientific name for 1/100th of a secondarrow_forward
- In Python Assume that you have a data structure of the following: A list of hockey players. Each hockey player is a list that includes their Rank (int), Name (String), Team (String), Age (int), Goals (int), Assists (int), Points (int) in that order. Write a function called most_goals that takes in the list of hockey players and returns the name of the player with the most goals. For example: Test Result print(most_goals(nhl_list1)) David Pastrnakarrow_forwardplease code in pythonWrite a Python modulet.py that gets a block of text from the user and prints some statistics about the text.These are the minimum required functions for this program. Design and implement these functions. Youcan create more functions if you choose to.arrow_forward2. Safir Market wants you to create a simple python program for calculating Total cost and change for their customers. The program should start by reading price, articles and Money. Then you need to do the following: • Create a void function that will display "Safir Market" • Create a function with return value and with parameters that will compute Total cost as product of articles and price • Create a function that will accept the computed Total cost as a parameter and will compute the change. If the Total cost is more than or equal to Money, the balance is to be calculated as Money - Total cost, otherwise print the message 'Money not enough' • Display the output as shown below, Sample output Enter the price: 345 Enter number of articles: 4 Enter Money: 65 -Safir Market Total cost is: 1380.0 Money not enough Change is: Nonearrow_forward
- Write a function flipCase that accepts a word as an argument and returns the same word but with each upper case letter switched to lower case and vice-versa. Example: >>> flipCase('ostrich')'OSTRICH' #note the lanuage is in pythonarrow_forward# Write a function called ex4() which implements a "Guess the number" game# 1. Generate a random number between 1 and 100# See https://docs.python.org/3/library/random.html for random number# generation details # 1. Prompt the user with the rules of the game ('q' to quit)# 2. Iterively prompt a user for a guess# 3. Let the user know whether their last guess was high or low# 4. Count the number of guesses# 5. Congratulate them upon success. Print the number of guesses# 6. Prompt to play again or quit# 7. Proceed as directed by user.# 8. Invoke ex4() and play a game to print resultsarrow_forwardHelp me do a program using Java functionsarrow_forward
- rografmiming CSC 101 Introduction VB Bau Section21436.02. Sntinn 2022 ta46581 OPRIV NEXTO Workbench Exercise 40443- deadne 04020022 1150 PM WORK AREA toThePowerOf is a function thet accepts two int parameters and returns the value of the first parameter raised to the power of the second. An int variable cubeside has aiready been deciared and initialized. Another int vaciable, cubevolume, has already been declared. Write a statement that calls toThePowerof to compute the value of cubeside raised to the power of 3, and store this value in cubevolume. SUBMIT 1 of 1: Sat Apr 02 2022 23:03:03 GMT-0400 (Eastern Dayilght Time) Type your selution here.arrow_forwardPython Help Write a function rectangle_area(length, width)Write a function rectangle_perimeter(length, width)Write a function circle_area(radius)Write a function circle_circumference(radius)Write a main function that asks the user to choose whether to find the area of a rectangle, the perimeter of a rectangle, the area of a circle or the circumference of a circle. If the user chooses to find the area of a circle use circle_area function, if the user chooses to find the area of a rectangle use rectangle_area function and so on.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
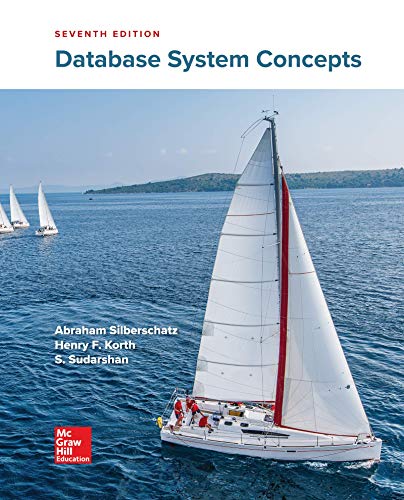
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
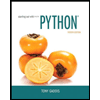
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
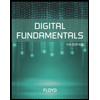
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
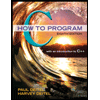
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
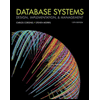
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
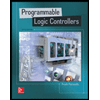
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education