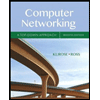
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
I want to write a program called Break.java. Using a while loop, it should prompt the user to enter an integer at most 10 times. If the user enters -1, exit the loop using a break statement. Count the number of times a user enters an integer (do not count times they enter -1), and display the count once the loop exits. Output should be like pictures attached below
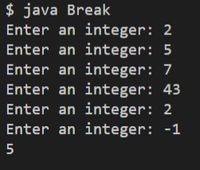
Transcribed Image Text:```plaintext
$ java Break
Enter an integer: 2
Enter an integer: 5
Enter an integer: 7
Enter an integer: 43
Enter an integer: 2
Enter an integer: -1
5
```
This is a sample Java program output. The program prompts the user to enter a series of integers, and the user inputs the numbers `2`, `5`, `7`, `43`, `2`. The loop continues until the user enters the integer `-1`, which presumably is a sentinel value that causes the loop to break.
The output `5` at the end might indicate the total number of integers entered before the break or some other logical result depending on the program's design beyond what's shown.
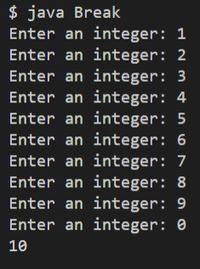
Transcribed Image Text:### Java Break Loop Example
Here is an example of a simple Java program that demonstrates the use of a break statement within a loop:
```plaintext
$ java Break
Enter an integer: 1
Enter an integer: 2
Enter an integer: 3
Enter an integer: 4
Enter an integer: 5
Enter an integer: 6
Enter an integer: 7
Enter an integer: 8
Enter an integer: 9
Enter an integer: 0
10
```
### Explanation
- **Program Execution**: The program appears to prompt the user to "Enter an integer" multiple times.
- **User Input**: The user inputs integers sequentially starting from 1 to 0.
- **Break Condition**: The loop terminates when the user enters the integer `0`.
- **Output**: The number `10` is printed after the loop ends, which may represent a summary or a result computed based on the input.
This example can be used to understand how user input can control the flow of a program using loops and break statements effectively.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

Knowledge Booster
Similar questions
- In Java Write a program to score the paper-rock-scissor game. Each of two users types ineither P, R, or S. The program then announces the winner as well as the basis fordetermining the winner: Paper covers rock, Rock breaks scissors, Scissors cut paper,or Nobody wins. Be sure to allow the users to use lowercase as well as uppercaseletters. Your program should include a loop that lets the user play again until theuser says she or he is done. The program should perform input validation for theplayer’s input (only ‘R’, ‘P’ or ‘S’ are acceptable).Sample Run:Player 1: Please enter either R)ock, P)aper, or S)cissors.RPlayer 2: Please enter either R)ock, P)aper, or S)cissors.SPlayer 1 wins.Totals to this move:Player 1: 1Player 2: 0Play Again? Y/y continues, other quits. YPlayer 1: Please enter either R)ock, P)aper, or S)cissors.SPlayer 2: Please enter either R)ock, P)aper, or S)cissors.PPlayer 1 wins.Totals to this move:Player 1: 2Player 2: 0Play Again? Y/y continues, other quits.…arrow_forwardWrite a program with the most appropriate loop that repeats user entered number of times (say the variable counts holds that number), asking the user counts times to enter numbers. The loop should also calculate the sum and the average of the numbers entered. Be sure to include the following: A class header with an appropriately named class. A main method. Use Scanner class for keyboard input. Declare and initialize any necessary variables. Use appropriate loop statement. Display any necessary input prompt and the output.arrow_forwardCan you please make this in Java?arrow_forward
- Your task: The maximum of the absolute values. For instance, if the numbers entered are [1, -7, -1, -3, 6, -2, 0], then the printed result must be 7.arrow_forwardIn Java: Forms often allow a user to enter an integer. Write a program that takes in a string representing an integer as input, and outputs yes if every character is a digit 0-9. Ex: If the input is: 1995 the output is: yes Ex: If the input is: 42,000 or 1995! the output is: no Hint: Use a loop and the Character.isDigit() function.arrow_forwardIn this lab you add two for loops to print an asterisk or a space in columns and rows to print out the letter A. The outer loop goes through each row. The inner loop moves from column to column. The inner loop will contain if then else statements that will decide whether to print an asterisk or a space. When a column is complete you have to print an endline statement. The output will look like : I\EFSC Spring 2015\COP 1000 Principles of Programming\Instructor Material Spring 2015\Workboo... Process exited after 0.02457 seconds with return value 0 Press any key to continue 1. Open the source code file named LetterASt.cpp in Dev C++ 2. Write the for loops to control the number of rows and columns that make up the letter A. The outer loop should go through the rows and the inner loop through the columns. 3. In the inner loop write the if then else statements to decide when to print an asterisk or a space. 4. Decide where you will need an end line statement to be printed after you have…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
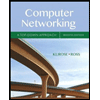
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
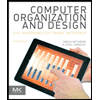
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
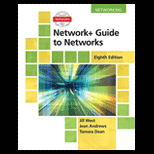
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
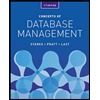
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
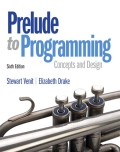
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
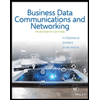
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY