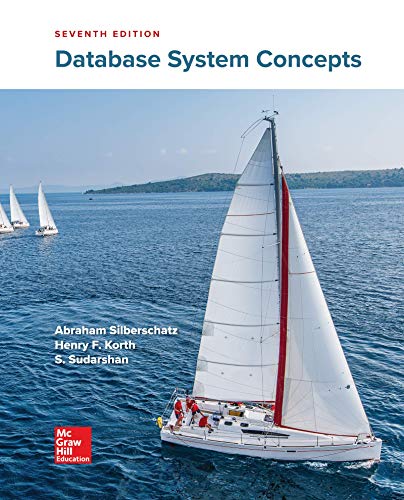
Using c++
Course information (derived classes)
Given main(), define a Course base class with functions to set and get the courseNumber and courseTitle. Also define a derived class OfferedCourse with functions to set and get instructorName, term, and classTime.
Ex. If the input is:
ECE287 Digitalthe output is:
Course Information: Course Number: ECE287 Course Title: Digital Systems Design Course Information: Course Number: ECE387 Course Title: Embedded Systems Design Instructor Name: Mark Patterson Term: Fall 2018 Class Time: WF: 2-3:30 pm#include "OfferedCourse.h"
int main() {
Course myCourse;
OfferedCourse myOfferedCourse;
string courseNumber, courseTitle;
string oCourseNumber, oCourseTitle, instructorName, term, classTime;
getline(cin, courseNumber);
getline(cin, courseTitle);
getline(cin, oCourseNumber);
getline(cin, oCourseTitle);
getline(cin, instructorName);
getline(cin, term);
getline(cin, classTime);
myCourse.SetCourseNumber(courseNumber);
myCourse.SetCourseTitle(courseTitle);
myCourse.PrintInfo();
myOfferedCourse.SetCourseNumber(oCourseNumber);
myOfferedCourse.SetCourseTitle(oCourseTitle);
myOfferedCourse.SetInstructorName(instructorName);
myOfferedCourse.SetTerm(term);
myOfferedCourse.SetClassTime(classTime);
myOfferedCourse.PrintInfo();
cout << " Instructor Name: " << myOfferedCourse.GetInstructorName() << endl;
cout << " Term: " << myOfferedCourse.GetTerm() << endl;
cout << " Class Time: " << myOfferedCourse.GetClassTime() << endl;
}

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 1 images

- In C++arrow_forwardA manager of a department at Google wants to schedule 15-min coffee chat between two employees every week for his 6 employees. Design and write a program to help this manager. Each employee should have a meeting with a new person. So, no meeting with the same person until they meet all the other colleagues. Use functional programming or object-oriented programing. You can have your own design to cover the requirements but you should consider a design with better time complexity. You can use any data structures (lists, dictionaries, stacks, queues, ...) The number 6 here is an example and imaging there are "n" (an even number) employees in the group (for this example n =6) Every week each employee should have a meeting and just one meeting with one person. Every week they should have a meeting with a new person. Until week 5 ( n-1 weeks) they should have a meeting with the same person. employees = [ employee_1, employee_2, employee_3, employee_4, employee_5, employee_6] It means…arrow_forwardCan you help me with a UML program please? I have a hard time understanding it Suppose you work at a robot company that has started building tiny disc-shaped vacuum cleaners that drive around people's houses and vacuum up debris. You are tasked with coding a program for the operation of this robot. Use that list of functions to inform your UML diagram. Using any program (like Draw.io or LucidChart) that creates UML diagrams, draft a UML diagram that is not too simple. Create your unique diagram. What interesting things can your robot do.? Be sure to follow the following Assure your symbols match the actions in your diagram (diamond shapes in decisions for instance). Check that your diagram is readable, and lines and text don't cross each other. Assure the diagram doesn't have too many lines crossing so it becomes confusing.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
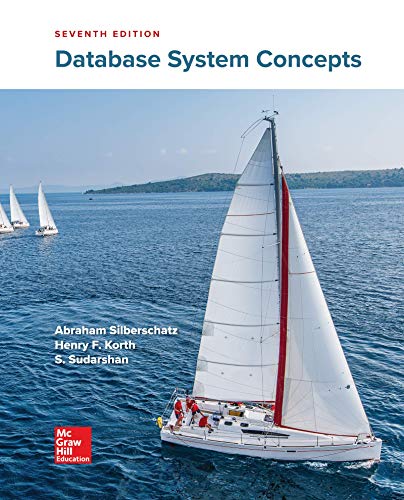
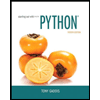
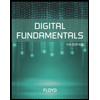
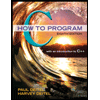
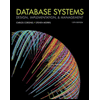
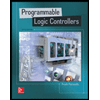