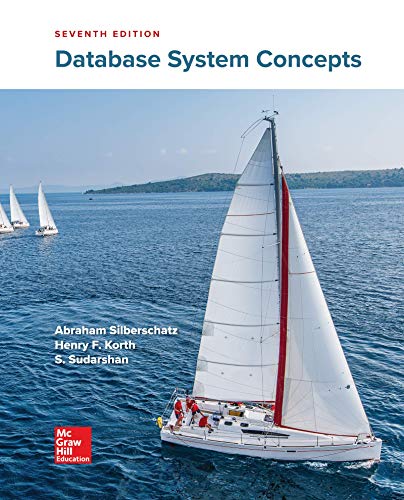
Using Java
3. Write the framework for a menu-based interface.
(a) Declare a boolean variable that will be used to determine if a loop should
keep going. Initialize it to true.
(b) Display a welcome message with your name.
(c) Write a do-while loop that uses the boolean variable as a loop condition. The
remaining steps should all appear in the body of the loop, unless stated
otherwise.
(d) Display the following menu:
Please choose from the following options:
1. Addition Quiz
2. Multiplication Quiz
3. Addition Table
4. Multiplication Table
5. Exit the program
(e) Read an int from the user and store it in a variable.
(f) Use that variable as a controlling expression for a switch statement.(g) For cases 1 and 2, output the following and exit the switch statement:
Quiz code goes here
(h) For cases 3 and 4, output the following and exit the switch statement:
Table code goes here
(i) For case 5, set the boolean variable to false and exit the switch statement.
(j) For the default case output the following and exit the switch statement:
Invalid choice
(k) Outside of the loop, display a thank-you message with your name.
4. Run your program to see if it works. Here is an example of what the output should
look like:
Welcome to Nicholas Coleman's arithmetic quiz program
Please choose from the following options:
1. Addition Quiz
2. Multiplication Quiz
3. Addition Table
4. Multiplication Table
5. Exit the program
1
Quiz code goes here
Please choose from the following options:
1. Addition Quiz
2. Multiplication Quiz
3. Addition Table
4. Multiplication Table
5. Exit the program
4
Table code goes here
Please choose from the following options:
1. Addition Quiz
2. Multiplication Quiz
3. Addition Table
4. Multiplication Table
5. Exit the program
5
Thank you for using John Leslie's arithmetic quiz program5. Write the code for the arithmetic quizzes.
(a) Create a named constant that represents the maximum value that you will
use for your quizzes and tables. Initialize it to 12.
(b) Remove the Quiz code goes here output statement.
(c) Replace it with a do-while statement that repeatedly prompts for a value
between 1 and the maximum value constant until the value entered is within
the range specified. The output should look something like this:
Please choose a number between 1 and 12
0
Please choose a number between 1 and 12
13
Please choose a number between 1 and 12
8
(d) After the do-while statement, initialize a variable to keep track of how many
questions the user got right to zero.
(e) Write a for statement that starts a counter at 1, increments it by 1 at each
iteration, and exits when the counter exceeds the maximum value constant.
(f) In the body of the for statement, do the following
i. Display a quiz question of the form NUM OP COUNTER =, where NUM
is the number the user chose, OP is either + or * depending on the
menu choice, and COUNTER is the counter.
ii. Read an int from the user and store it in a variable.
iii. If the user’s answer is correct, increment the number of questions the
user got right.
(g) After the for statment, display how many quiz questions the user got right.
6. Run your program to see if it works. Here is an example of what the output should
look like:
Please choose from the following options:
1. Addition Quiz
2. Multiplication Quiz
3. Addition Table
4. Multiplication Table
5. Exit the program
1Please choose a number between 1 and 12
8
8 + 1 =
9
8 + 2 =
10
8 + 3 =
11
...
8 + 10 =
18
8 + 11 =
19
8 + 12 =
20
You got 12 right out of 12
Please choose from the following options:
1. Addition Quiz
2. Multiplication Quiz
3. Addition Table
4. Multiplication Table
5. Exit the program
5
Thank you for using John Leslie's arithmetic quiz program
7. Write the code for the arithmetic tables.
(a) Remove the Quiz code goes here output statement.
(b) Display four spaces followed by a | character.
(c) Use a for loop to display the column headings on the same line, which should
start at one and go up to the maximum value constant. Each column heading
should take up four characters.
(d) On the next line display four - characters followed by a + character.
(e) Use a for loop to display four - characters under the column headings in the
previous line.
(f) The output so far should look like this:
| 1 2 3 4 5 6 7 8 9 10 11 12
----+------------------------------------------------
(g) Use a for loop to display each of the row headings on a different line. Each
row heading should take up four characters followed by a | character.(h) The row headings should look like this:
1|
2|
3|
4|
5|
6|
7|
8|
9|
10|
11|
12|
(i) Inside of the row heading loop, write a nested for loop that displays a row of
the table. Each entry should take up 4 characters and contain the result of
adding or multiplying the row and column numbers depending on the menu
option chosen by the user

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Please help me with this using java. create a trivia game menu. Include the following: 1. Background (using fractals and recursion) (I would like a black brick wall as as the fractal for the background of the game menu 2. include the three clickable buttons ( play, settings, and instructions) 3. Include a title (Quiz time) (Image show below)arrow_forwardCreate a calculator that only supports division and multiplication operations using Java and a graphical user interface (GUI). Clear, ON, and OFF buttons must all be present.arrow_forwardOverview In this assignment, you will gain more practice with designing a program. Specifically, you will create pseudocode for a higher/lower game. This will give you practice designing a more complex program and allow you to see more of the benefits that designing before coding can offer. The higher/lower game will combine different programming constructs that you have been learning about, such as input and output, decision branching, and a loop. Higher/Lower Game DescriptionYour friend Maria has come to you and said that she has been playing the higher/lower game with her three-year-old daughter Bella. Maria tells Bella that she is thinking of a number between 1 and 10, and then Bella tries to guess the number. When Bella guesses a number, Maria tells her whether the number she is thinking of is higher or lower or if Bella guessed it. The game continues until Bella guesses the right number. As much as Maria likes playing the game with Bella, Bella is very excited to play the game…arrow_forward
- Accumulating Totals in a Loop Summary In this lab, you add a loop and the statements that make up the loop body to a Java program that is provided. When completed, the program should calculate two totals: the number of left-handed people and the number of right-handed people in your class. Your loop should execute until the user enters the character X instead of L for left-handed or R for right-handed. The inputs for this program are as follows: R, R, R, L, L, L, R, L, R, R, L, X Variables have been declared for you, and the input and output statements have been written. Instructions Ensure the file named LeftOrRight.java is open. Write a loop and a loop body that allows you to calculate a total of left-handed and right-handed people in your class. Execute the program by clicking Run and using the data listed above and verify that the output is correct.arrow_forwardjavascripts write a loop prints all three digits number in decreasing order (999 , 998 ,997,..,101,100)arrow_forwardJava Program A string is a palindrome if it is identical forward and backward. For example “anna”,“ civic”, “level” and “hannah” are all examples of palindromic words.Write a programt thatreads a string from the user and uses a loop to determines whether or not it is appalindrome Display the result, including a meaningful output message.arrow_forward
- Printing Triangles with Nested Loops Due Date: Thursday, April 13 at 11:59 PM Task Write a JAVA program that reads the height of a triangle (less than 10) from the user and then calls two void methods named plotTril and plotTri2. Each of the 2 methods should have a parameter for the height of the triangle and use printf inside of nested For loops to produce the output of the respective triangle for the user. Note that the numbers in the triangle are left-aligned. Create a project and class named HW8FirstnameLastname in NetBeans using your actual first and last names to hold your program. For height = 8, plotTril and plotTri2 will print the following triangles respectively. 12 1 1 2 1 4 2 8 4 16 8 32 16 1 2 4 1 2 4 8 1 2 4 8 4 12 2 1 844H 2 16 1 NL 1 2 48 16 32 2 1 1 724 COM 8 16 32 64 1 2 48 1 36 24 16 8 00 32 16 64 32 128 64 1 2 4 8 16 32 1 2 4 8 16 1 2 4 8 1 2 4 1 2 1arrow_forwardPrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forwardJAVAarrow_forward
- Java program I need help creating a program that creates a Christmas tree. It has a method that accepts two parameters (one for the number of segments and one for the height of each segment). The left tree has 3 segments with a height of 4 and the right one has two segments with a height of 5. Can you explain how the for loops would work?arrow_forwardComputer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwardImplement the counter increment before returning to the loop's beginning. After completing these stages, your code should resemble the following, implementing the counter control loop's fundamental structure: .text li $s0, 0 lw $s1, n start_loop: sle $t1, $s0, $s1 beqz $t1, end_loop # code block addi $s0, $s0, 1 b start_loop end_loop:.data n: .word 5arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
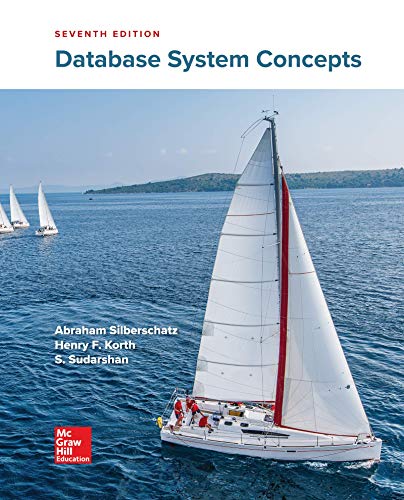
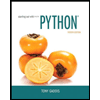
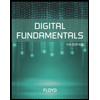
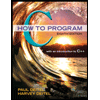
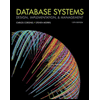
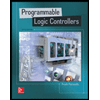