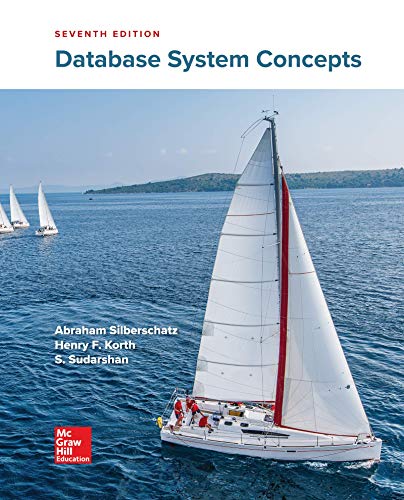
Concept explainers
using loops in C++
Write a program that will predict the size of a population of organisms. The program should ask the user for the starting number of organisms, their average daily population increase (as a percentage, expressed as a fraction in decimal form: for example 0.052 would mean a 5.2% increase each day), and the number of days they will multiply. A loop should display the size of the population for each day.
Prompts, Output Labels and Messages .The three input data should be prompted for with the following prompts: "Enter the starting number of organisms: ", "Enter the average daily population increase (as a percentage): , and "Enter the number of days they will multiply: " respectively. The population sizes displayed should appear on separate lines, each of the form "On day D the population size was P." where D is the day number (starting with 1) and P is the population you calculated for that day.
Input Validation.Do not accept a number less than 2 for the starting size of the population. If the user fails to satisfy this print a line with this message "The starting number of organisms must be at least 2.", display the prompt again and try to read the value . Similarly, do not accept a negative number for average daily population increase , using the message "The average daily population increase must be a positive value ." and retrying. Finally, do not accept a number less than 1 for the number of days they will multiply and use the message "The number of days must be at least 1."

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Your program will prompt the user for the following information: current population number of yearly births from the previous year number of yearly deaths from the previous year number of years into the future for your predictive value of the future population. You will then compute the following: birth rate death rate growth rate the predicted future population Assignment Notes: To allow the user to input the numeric data (such as current population) it is necessary to use the input function. The input function takes a string in parenthesis that prompts the user what to enter. The computer then waits until the user types a response, terminated by the user typing the Enter key. A string, as a sequence of characters, is returned to the variable on the lefthand side of the assignment statement. For example: currPopulation = input(“Enter the current US population”) The data in currPopulation is a string (because that is what the input statement does) but we need it to be a number…arrow_forwardTest2_Q2 Test Content Question 1 16 Points Write a JAVA program using loop that asks the user to input seven integer numbers representing. the humidity level in Bahrain for each of the day of the week (1 represents Sunday, 2 represents Monday, etc.). The program should find and display the following: 1- the maximum humidity level and the day on which it was recorded. 2- the number of days the humidity level was lower than 40. *Assume that there is only one maximum humidity level. Sample Input / Output Enter the humidity for 1 week: 57 61 37 49 50 62 38 The Maximum Humidity is 62 for the Week Day = 6 The no. of days the humidity level < 40 is 2 Note: Your answer should follow the given sample input / output.arrow_forwardcalcAverage function: This is function that returns no value and accept no parameters. This function will do the following: Ask the user to enter how many integers to enter. This has to be a positive integer and you need to validate that Write a “for” loop to accept this many integers and write the needed logic to calculate the average of these numbers and print that out Then the function will return back to the main functionarrow_forward
- mystery_value = 5 #You may modify the lines of code above, but don't move them!#When you Submit your code, we'll change these lines to#assign different values to the variables. #Write a program that divides mystery_value by mystery_value#and prints the result. If that operation results in an#error, divide mystery_value by (mystery_value + 5) and then#print the result. If that still fails, multiply mystery_value#by 5 and print the result. You may assume one of those three#things will work.##You may not use any conditionals.# #Add your code here!arrow_forwardUsing a Sentinel Value to Control a while Loop in Java Summary In this lab, you write a while loop that uses a sentinel value to control a loop in a Java program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the file named MovieGuide.java is open. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop to calculate the average star rating. Execute the program by clicking Run. Input the following: 0, 3, 4, 4, 1, 1, 2, -1 Check that the…arrow_forwardMUST BE IN PYTHON PROGRAMMING CODE!!!!! NO C++ or Java!! Directions: Design a program with a loop that lets the user enter a series of names (in no particular order). After the final person’s name has been entered, the program should display the name that is first alphabetically and the name that is last alphabetically. For example, if the user enters the names Kristin, Joel, Adam, Beth, Zeb, and Chris, the program would display Adam and Zeb. Additional requirements: Note that the possible set of input values can include negative, 0, or positive values, but not the sentinel value of -99. DESIGN HINT: • When determining the largest (or smallest) value in a set of numbers, remember to use variables to keep track of the current largest (or smallest) number found thus far. These variables can then be used as a comparison to each subsequent input number to see if the new number is larger (or smaller) than the current largest (or smallest) number and updated appropriately. At the end of all…arrow_forward
- Java:arrow_forwardA retail company assigns a $5000 store bonus if monthly sales are more than $100,000; otherwise a $500 store bonus is awarded. Additionally, they are doing away with the previous day off program and now using a percent of sales increase to determine if employees get individual bonuses. If sales increased by at least 4% then all employees get a $50 bonus. If they do not, then individual bonuses are 0. Step 7: The final step in completing the pseudocode is to call all the modules with the proper arguments. Complete the missing lines. Module main () //Declare local variables Declare Real monthlySales Declare Real storeAmount Declare Real empAmount Declare Real salesIncrease //Function calls Call getSales(monthlySales) Call getIncrease(salesIncrease) Call ______________(_______________, _____________) Call ______________(_______________, _____________) Call ______________(_______________, _____________) End Modulearrow_forwardPrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forward
- P7arrow_forwardin c++arrow_forwardprogram must include a processing loop that allows multiple sets of data to be processed and the program should be terminated when there is no more data to process Develop a program in python that allows the user to enter a start value of 1 to 4, a stop value of 5 to 12 and a multiplier of 2 to 8. The program must display a multiplication table with results using these values. For example, if the user enters a start value of 3, a stop value of 7 and a multiplier value of 3, the table should be displayed as follows: Multiplication Table 3 x 3 = 9 3 x 4 = 12 3 x 5 = 15 3 x 6 = 18 3 x 7 = 21 Run the program with the following values: Start Stop Multiplier 2 10 4 4 12 6arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
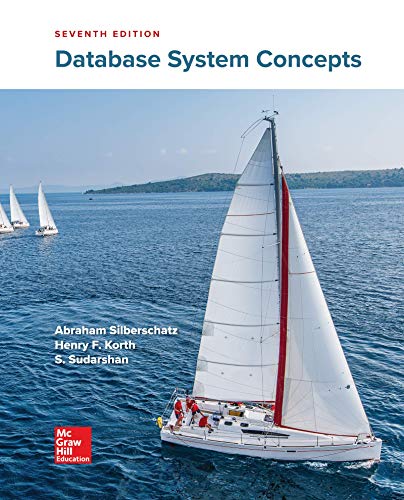
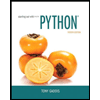
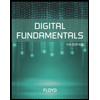
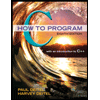
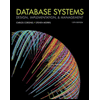
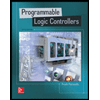