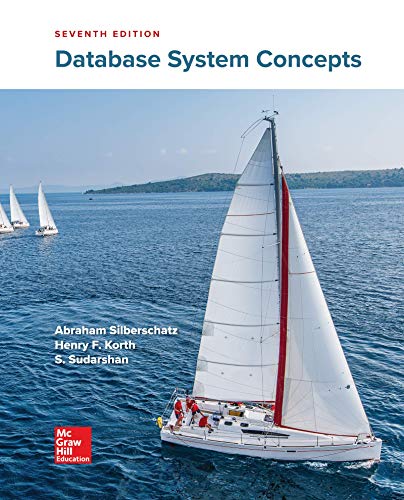
Using polymorphism and object-oriented programming Design an Elevator simulation. The simulation have 4 different types of elevators and passengers.
There are 4 types of passengers in the system:
Standard: This is the most common type of passenger and has a request percentage of 70%.
Standard passengers have no special requirements.
VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority
and are more likely to be picked up by express elevators.
Freight: This type of passenger has a request percentage of 15%. Freight passengers have large
items that need to be transported and are more likely to be picked up by freight elevators.
Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items
that need to be transported and are more likely to be picked up by glass elevators.
There are 4 types of elevators in the system:
StandardElevator: This is the most common type of elevator and has a request percentage of 70%.
Standard elevators have a maximum capacity of 10 passengers.
ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a
maximum capacity of 8 passengers and are faster than standard elevators.
FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a
maximum capacity of 5 passengers and are designed to transport heavy items.
GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a
maximum capacity of 6 passengers and are designed to transport fragile item
The input should look like this:
# Building parameters
floors=30
# Passengers to add to floors
add_passenger 1 6 25 Standard 30
add_passenger 2 2 28 VIP 10
add_passenger 3 7 15 Freight 20
add_passenger 4 4 20 Glass 15
# Elevator types
elevator_type StandardElevator 10 40
elevator_type ExpressElevator 8 25
elevator_type FreightElevator 5 20
elevator_type GlassElevator 6 15
# Percentage of passenger requests for each elevator type
request_percentage StandardElevator 70
request_percentage ExpressElevator 20
request_percentage FreightElevator 5
request_percentage GlassElevator 5
# Percentage of passenger requests for each passenger type
passenger_request_percentage Standard 70
passenger_request_percentage VIP 10
passenger_request_percentage Freight 15
passenger_request_percentage Glass 5
# Number of elevators in the system
number_of_elevators 8
# Run simulation for 60 iterations
run_simulation 60
Use these classes to make the elevator simulation:
Main class:
public class SimulatorSettings {
SimulatorSettings(String fileName){
}
private int nofloors;
public int getNofloors() {
return nofloors;
}
public void setNofloors(int nofloors) {
this.nofloors = nofloors;
}
}
Other Classes:
public class Simulations {
while(scanner.hasNextLine()){
String line = scanner.nextLine();
if(line.startsWith("floor="))
{
line= line.replace("floor=", "");
// Convert the value to the wanted type
System.out.println(line);
}
}
settings.setNofloors(55);
Passenger pass1 = new StandardPassenger();
pass1.requestElevator(settings);
ArrayList<Passenger> passengers = null;
for(int i = 0; i < 100; i++){
//Use the percentage from the file
passengers.add(new StandardPassenger());
}
}
}
public abstract class Passenger {
public static int passangerCounter = 0;
private String passengerID;
protected int startFloor;
protected int endFloor;
Passenger(){
this.passengerID = ""+passangerCounter;
passangerCounter++;
}
public abstract boolean requestElevator(SimulatorSettings settings);
}
public class StandardPassenger extends Passenger{
private String type;
public StandardPassenger() {
}
public boolean requestElevator(SimulatorSettings settings){
Random rand = new Random();
this.startFloor = rand.nextInt()% settings.getNofloors();
this.endFloor = rand.nextInt() % settings.getNofloors();
while(this.startFloor == this.endFloor){
this.endFloor = rand.nextInt() % settings.getNofloors();
}
return true;
}
}
And make Other 3 passengers classes that use polymorphism.
public class Elevator {
private int currentFloor = 0;
private int maxFloor;
public int getCurrentFloor() {
return currentFloor;
}
public int getMaxFloor() {
return maxFloor;
}
public void setCurrentFloor(int currentFloor) {
this.currentFloor = currentFloor;
}
public void setMaxFloor(int maxFloor) {
this.maxFloor = maxFloor;
}
}
And make Other 4 elevator classes that use polymorphism.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- The goal of this coding exercise is to create two classes BookstoreBook and LibraryBook. Both classes have these attributes: author: Stringtiltle: Stringisbn : String- The BookstoreBook has an additional data member to store the price of the book, and whether the book is on sale or not. If a bookstore book is on sale, we need to add the reduction percentage (like 20% off...etc). For a LibraryBook, we add the call number (that tells you where the book is in the library) as a string. The call number is automatically generated by the following procedure:The call number is a string with the format xx.yyy.c, where xx is the floor number that is randomly assigned (our library has 99 floors), yyy are the first three letters of the author’s name (we assume that all names are at least three letters long), and c is the last character of the isbn.- In each of the classes, add the setters, the getters, at least three constructors (of your choosing) and override the toString method (see samplerun…arrow_forwardThe Point2D should store an x and y coordinate pair, and will be used to build a new class via class composition. A Point2D has a x and a y, while a LineSegment has a start point and an end point (both of which are represented as Point2Ds). class Invariants The start and end points of a line segment should never be null Initialize these to the origin instead. Data A LineSegment has a start point This is a Point2D object All data will be private A LineSegment also has an end point. Also a Point2D object Methods Create getters and setters for your start and end points public Point2D getStartPoint() { public void setStartPoint(Point2D start) { Create a toString() function to build a string composed of the startPoint’s toString() and endPoint’s toString() Should look like “Line start(0,0) and end(1,1)” Create an equals method that determines if two LineSegments are equal public boolean equals(Object other) { if(other == null || !(other instanceof LineSegment)) return…arrow_forwardWrite GroceryChain class with instance variables and a constructor. Each GroceryChain has a name, number of stores it owns, and annual revenue.arrow_forward
- For this problem you are to join the following classes into a hierarchy (use the UML diagrams from Module 2 to get started): Player - Super Class Archer - Subclass Barbarian - Subclass Cleric - Subclass Rogue - Subclass Warlock - Subclass If the parent class has the same attributes as the sub class then you can remove those attributes from the subclass. If the class is new for this module, please come up with 2 unique attributes of your choice, and 1 piece of functionality. If the sub classes are shown on the same level, then they share a parent class.arrow_forwardJAVA QUESTION REQUIRES SEPARATE CLASSES, CANNOT BE WRITTEN IN ONE MAIN METHOD E.G. ACCOUNT SECTION WRITTEN IN ACCOUNT CLASS, CUSTOMER SECTION WRITTEN IN CUSTOMER CLASS ETC. Phase 2 In this phase you’ll be writing code within the methods of your classes to make the tests pass. Account Each account should have a constant, unique id. Id numbers should start from 1000 and increment by 5. The ACCOUNT_ID attribute should be initialised when the account object is created. The id should be generated internally within the class, it should not be passed in as an argument. The deposit method should increase the balance by the value passed in as an argument. The withdraw method should reduce the balance by the value passed in as an argument. It should return the value of the argument The account should be able to go overdrawn The correctBalance method should change the balance to match the value passed in as an argument. Testing your code for Account Run TestAccountInitialisation to check…arrow_forwardUsing Java an elevator simulator that uses polymorphism and object-oriented programming tosimulate the movement of elevators in a building with multiple types of passengers and elevators.The system has 8 elevators, each of which can be one of 4 types of elevators, with a certainpercentage of requests for each type. Similarly, each passenger can be one of 4 types, with adifferent percentage of requests for each type. Passenger Types:There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%.Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priorityand are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have largeitems that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request…arrow_forward
- Brainstorm below how you want to organize a game state class. This class keeps track of all information required to run the game. Remember, a class is composed of: State (data that is stored about an object) Behavior (behaviors of an object) For example, if you were to represent a Wordle game as a class, what data is stored within the game you’d want to have as member variables? What actions/behaviors can be taken?arrow_forwardCreate a class Person to represent a person according to the following requirements: A person has two attributes: id Add a constructer to initialize all the attributes to specific values. Add all setter and getter methods. Create a class Product to represent a product according to the following requirements: A product has four attributes: a reference number (can’t be changed) a price an owner (is a person) a shopName (is the same for all the products). Add a constructer without parameters to initialize all the attributes to default values (0 for numbers, "" for a string and null for object). Add a second constructer to initialize all the attributes to specific values. Use the keyword "this". Add the method changePrice that change the price of a product. The method must display an error message if the given price is negative. Add a static method changeShopName to change the shop name. Add all the getter methods. The method getOwner must return an owner. Create the class…arrow_forwardCode in JAVA and see attached images for information on classes and methods A company pays its personnel on a weekly basis. The personnel are of 4 types: FixedWeekly personnel are paid a fixed amount regardless of the number of hours workedByTheHour personnel are paid by the hour and receive overtime pay for all hours worked in excess of 40PercentOfSales personnel are paid a percentage of their salesFixedWeeklyPercentOfSales personnel receive a fixed amount plus a percentage of their sales. Create a class called Personnel. This class will represent the general concept of all personnel. All 4 types of personnel are considered Personnel. FixedWeeklyPercentOfSales personnel are considered to be PercentOfSales Personnel. The Personnel class will also be Payable. You will need to create the Payable interface. It will contain just one method called earnings(). Test Run Personnel processed polymorphically: FixedWeekly personnel: Harry Clarksocial security number: 111-11-1111weekly…arrow_forward
- Please prepare the case diagram and the class diagramarrow_forwardIf you fly in economy class, then you can be promoted to businessclass, especially if you have a gold airline card for privateflights. If you do not have a gold card, you can "reset" fromflight if the plane is full or you are late for check-in. All thesethe conditions are shown in the diagram below. Note that all statements(operators) are numbered. You run 3 tests:Test 1 - Gold card holder upgraded to business classTest 2 - Passenger without gold card remains in economy classTest 3 - Passenger who was "thrown" off the flightWhat is the statement coverage of these three tests?A. 60%B. 70%C. 80%D. 90%arrow_forwardThe total cost of a group of items at a grocery store is based on the sum of the individual product prices and the tax (which is 5.75%). Products that are considered “necessities” are not taxed, whereas products that are considered “luxuries” are. The Product class is abstract, and it has a method called getTotalPrice. Your task is to create two subclasses of Product: NecessaryProduct and LuxuryProduct and implement the getTotalPrice method in each of these classes appropriately. Then modify the driver program to instantiate four products (two necessary and two luxury) and store them in the product array, print out each item in the array, and display the total cost of the items. You should not make any changes at all to Product.java, and you should only add to ShoppingTripStartingCode.java. Do not change any code that is already present Example(Cheese and bread are necessities and soda and candy are luxuries)Cheese…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
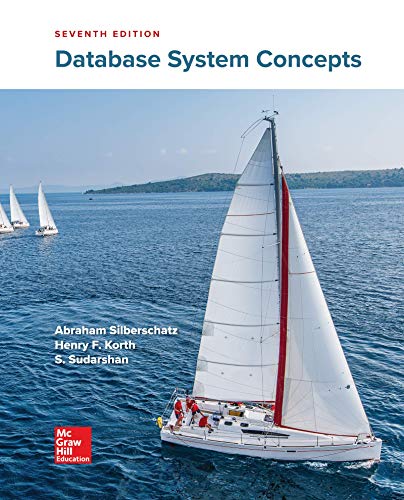
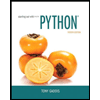
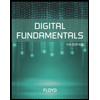
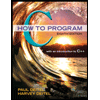
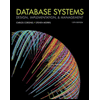
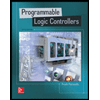