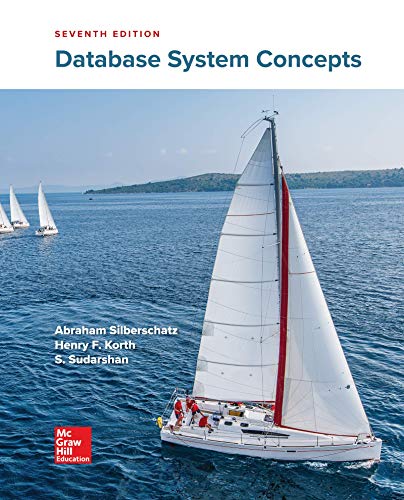
Concept explainers
We can create a numpy array from separate lists by specifying a format for each field:
roster = np.zeros(4, dtype={'names': ('name', 'age', 'major', 'gpa'), 'formats': ('U50', 'i4', 'U4', 'f8')})
roster['name'] = ['Alice', 'Bob', 'Carol', 'Dennis']
roster['age'] = [21, 25, 18, 29]
roster['major'] = ['CS', 'Math', 'Chem', 'Phys']
roster['gpa'] = [3.8, 3.2, 4.0, 3.5]
where U50 is a string of max length 50, i4 is a 4-byte integer, U4 is a string of max length 4, and f8 is an 8-byte floating-point number. Then roster has value
array([('Alice', 21, 'CS', 3.8), ('Bob', 25, 'Math', 3.2),
('Carol', 18, 'Chem', 4. ), ('Dennis', 29, 'Phys', 3.5)],
dtype=[('name', '<U50'), ('age', '<i4'), ('major', '<U4'), ('gpa', '<f8')])
Then, for example, roster['name'] will give an array of just the student names.
Write a program that reads the numpy array roster we just created that has this format:
name,age,major,gpa
Convert this to a numpy array as shown above. Then use that array to compute and print each of the following:
- The average GPA of all students
- The maximum GPA of students majoring in CS
- The number of students with a GPA over 3.5
- The average GPA of students who are at least 25 years old
- The major that has the highest average GPA among students at most 22 years old
For example, for the four students listed, your program should print:
3.625
3.8
2
3.35
Chem

1. Create the roster array using numpy with student data including name, age, major, and GPA.
2. Define a function, safe_mean(arr), that calculates the mean while handling empty slices:
- If the length of arr is 0, return NaN.
- Otherwise, calculate the mean using np.nanmean.
3. Calculate the average GPA of all students:
- Use safe_mean to compute the mean of roster['gpa'].
4. Calculate the maximum GPA of students majoring in CS:
- Filter students majoring in CS and calculate the maximum GPA.
- Handle empty slices using safe_mean.
5. Calculate the number of students with a GPA over 3.5:
- Use np.sum to count the number of students with GPA > 3.5.
6. Calculate the average GPA of students who are at least 25 years old:
- Filter students with age >= 25, and calculate the average GPA.
- Handle empty slices using safe_mean.
7. Calculate the major with the highest average GPA among students at most 22 years old:
- Iterate through unique majors.
- For each major, filter students in that major and at most 22 years old.
- Calculate the average GPA using,safe_mean.
8. Print the results:
- Print the average GPA of all students.
- Print the maximum GPA of CS students.
- Print the number of students with GPA > 3.5.
- Print the average GPA of students aged 25 or older.
- Print the major with the highest average GPA among students aged 22 or younger.
Step by stepSolved in 4 steps with 2 images

- Vectors are synchronized while ArrayLists are not. Group of answer choices True Falsearrow_forwardPython Write a program that allows the user to add, view, and delete names in an array list. The program must present the user with options (add, view, or delete) to choose from and an option to quit/exit the program. For example: Add adds name(s) to the list. View - view name(s) in the list. Delete - removes name(s) from the list. The user must type the option (Add, View, or Delete), and the program must work accordingly.arrow_forwardPoker A poker hand can be stored in a two-dimensional array. The statement int hand[4][13]; declares an array with 52 elements, where the first subscript ranges over the four suits and the second subscript ranges over the thirteen ranks. A poker hand is specified by placing 1's in the elements corresponding to the cards in the hand. See Figure 1. Figure 1 A 2 3 4 5 6 7 8 9 10 J Q K Club 1 Diamond Heart ♥ 1 Spade a 0|0 1 1, 1,arrow_forward
- 18. The mean of a set of numbers is the average computed by dividing the sum of the numbers by the quantity of the numbers. Complete method getMean below. // precondition: // postcondition: list is a non-empty array of integers. getMean returns the mean of the integers stored by list. public static double getMean(int[] list) {arrow_forwardint [] arrayInt={3,54,90,22,32}; To print the last element in the arrayInt you will write: a. System.out.println(arrayInt[5]); b. System.out.println(arrayInt[0]); c. System.out.println(arrayInt[length]); d. System.out.println(arrayInt[4]);arrow_forwardLook at the following array definition String[] Students= {"St1", "St222", "St3333"}; what will be returned by Students.length what will be returned by Students[1].length() how many total elements can be saved in this arrayarrow_forward
- P__Given an empty ArrayList<Integer> numList, what are numList's contents after the following operations: numList.add(12);numList.add(14);numList.add(16);numList.set(1, 13);numList.add(0, 10);.arrow_forwardJava Task 1 Create an object of type FitnessExperiment that stores an array of StepsFitnessTracker, DistanceFitnessTracker, and HeartRateFitnessTracker objects. You could use code such as the following to initialise the array containing the fitness tracker measurements: FitnessTracker[] trackers = { new StepsFitnessTracker("steps", new Steps(230)), new StepsFitnessTracker("steps2", new Steps(150)), new StepsFitnessTracker("steps2", new Steps(150)), new HeartRateFitnessTracker("hr", new HeartRate(80)), new HeartRateFitnessTracker("hr", new HeartRate(80)) }; In the FitnessExperiment class, complete the implementation of the methods named getTotalSteps()and printExperimentDetails(). The comments in the code specify how they should operate and provide you with additional hints to get you started. You can use the method getSteps()in StepsFitnessTracker, but think how you will know the real type of each object in the array of fitness trackers (trackers in the example above). FitnessTracker…arrow_forwardPOEM_LINE = str POEM = List[POEM_LINE] PHONEMES = Tuple[str] PRONUNCIATION_DICT = Dict[str, PHONEMES] def all_lines_rhyme(poem_lines: POEM, lines_to_check: List[int], word_to_phonemes: PRONUNCIATION_DICT) -> bool: """Return True if and only if the lines from poem_lines with index in lines_to_check all rhyme, according to word_to_phonemes. Precondition: lines_to_check != [] >>> poem_lines = ['The mouse', 'in my house', 'electric.'] >>> lines_to_check = [0, 1] >>> word_to_phonemes = {'THE': ('DH', 'AH0'), ... 'MOUSE': ('M', 'AW1', 'S'), ... 'IN': ('IH0', 'N'), ... 'MY': ('M', 'AY1'), ... 'HOUSE': ('HH', 'AW1', 'S'), ... 'ELECTRIC': ('IH0', 'L', 'EH1', 'K', ... 'T', 'R', 'IH0', 'K')} >>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes) True…arrow_forward
- Q2 You have a dynamic array with 3 elements in it and a capacity of 4. The capacity doubles when additional space is needed and shrinks by 50% (rounding down) when the size is at or below 1/3 capacity. Answer the questions below for the following operation sequence: Add, Add, Remove, Remove, Remove Q2.1 What will be the capacity of the list when the whole sequence is completed? Answer= Enter your answer herearrow_forward6. the grade is under 20 which is outlier, remove it from the array list. 7. Print array list using System.out.println() 8. Use indexOf to print index of 80. 9. Use get function. 10. What is the difference between get and index of? 11. Print the values of the array list using Iterator class. 12.. Delete all the values of the array list using clear function. 13. Print all the values of the array after you execute clear using System.out.println(). what is the result of using clear function? 14. What is the shortcoming of using array List?arrow_forwardTradng SelCLOon Write the state of the elements of the vector below after each of the first 3 passes of the outermost loop of the selection sort algorithrm // index 0 1 2 3 4 5 6 7 Vector numbers = {29, 17, 3, 94, 46, 8, -4, 12}; selectionSort(numbers); For example, if the vector was {(43125), you would write: {4 3 1 2 5} {1 3 4 2 5} (1 2 4 3 5} {1 2 3 4 5} Thought: How many vectors would I need here if I were to implement this in code? Write your answer here. hparrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
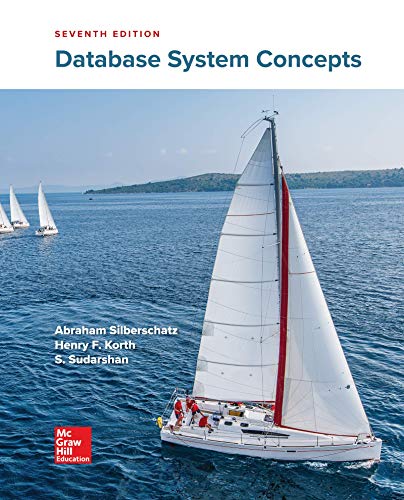
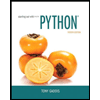
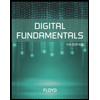
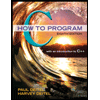
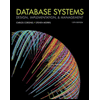
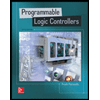