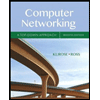
What am I doing wrong on this and how can I fix it?
#include <iostream>
#include <string>
using namespace std;
//declare Student Class
class Student
{
//private member of class
private:
string Full_Name;
float Student_GPA;
int Student_Rank;
//public class members
public:
//function to get student data
void setStudentData(string studentName,float studentGpa,int studentRank)
{
this->Full_Name = studentName;
this->Student_GPA = studentGpa;
this->Student_Rank = studentRank;
}
//return student data function
string getStudentData(string &studentName, float &studentGpa, int &StudentRank)
{
return Full_Name;
return Student_GPA;
return Student_Rank;
}
};
int main()
{
int Number_Student;
//prompt user to enter total number of student
cout << "Please input the total students: ";
cin >> Number_Student;
Student Info[Number_Student];
//variable declaration
string studentName[20];
float studentGpa;
int studentRank;
//prompt user to enter name GPA and rank
for (int i = 0; i < Number_Student; i++)
{
cout << "\nPlease enter the information for the student " << i + 1;
cout << "\nStudent Name: ";
cin.get();
getline(cin >> ws, studentName[i]);
cout << "GPA: ";
cin >> studentGpa;
cout << "Student Rank(Year): ";
cin >> studentRank;
Info[i].setStudentData(studentName, studentGpa, studentRank);
}
//Displays the Winner of the Scholarship contest
cout << "\nThe winner of the scholarship contest is: ";
float highest = -1;
int index = -1;
for (int i = 0; i < 3; i++)
{
info[i].getStudentData(studentName, studentGpa, studentRank);
if (highest < studentGpa)
{
index = i;
highest = studentGpa;
}
}
Info[index].getStudentData(studentName, studentGpa, studentRank);
cout << "\nThe winner of the scholarship contest is: " << endl;
cout << "Name: " << studentName << endl;
cout << "GPA: " << studentGpa << endl;
cout << "Rank: " << studentRank << endl;
cout << "Congratulations " << studentName << "!\n" << endl;
cout << "You will get your scholarship funds deposited in your student account";
system("pause");
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Create an Organization class. Organization has 10 Employees (Hint: You will need an array of pointers to Employee class) Organization can calculate the total amount to be paid to all employees Organization can print the details(name & salary) of all employees note: write code in main,header and function.cpp filearrow_forwardMake Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardIn C++ struct myGrades { string class; char grade; }; Declare myGrades as an array that can hold 5 sets of data and then set a class string in each position as follows: “Math” “Computers” “Science” “English” “History” and give each class a letter gradearrow_forward
- Sultan Qaboos University Department of Computer Science COMP2202: Fundamentals of Object Oriented Programming Assignment # 2 (Due 5 November 2022 @23:59) The purpose of this assignment is to practice with java classes and objects, and arrays. Create a NetBeans/IntelliJ project named HW2_YourId to develop a java program as explained below. Important: Apply good programming practices: 1- Provide comments in your code. 2- Provide a program design 3- Use meaningful variables and constant names. 4- Include your name, university id and section number as a comment at the beginning of your code. 5- Submit to Moodle the compressed file of your entire project (HW1_YourId). 1. Introduction: In crowded cities, it's very crucial to provide enough parking spaces for vehicles. These are usually multistory buildings where each floor is divided into rows and columns. Drivers can park their cars in exchange for some fees. A single floor can be modeled as a two-dimensional array of rows and columns. A…arrow_forwardC:/Users/r1821655/CLionProjects/untitled/sequence.cpp:48:5: error: return type specification for constructor invalidtemplate <class Item>class sequence{public:// TYPEDEFS and MEMBER SP2020typedef Item value_type;typedef std::size_t size_type;static const size_type CAPACITY = 10;// CONSTRUCTORsequence();// MODIFICATION MEMBER FUNCTIONSvoid start();void end();void advance();void move_back();void add(const value_type& entry);void remove_current();// CONSTANT MEMBER FUNCTIONSsize_type size() const;bool is_item() const;value_type current() const;private:value_type data[CAPACITY];size_type used;size_type current_index;};} 48 | void sequence<Item>::sequence() : used(0), current_index(0) { } | ^~~~ line 47 template<class Item> line 48 void sequence<Item>::sequence() : used(0), current_index(0) { }arrow_forward#include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; class Movie { private: string title = ""; int year = 0; public: void set_title(string title_param); string get_title() const; // "const" safeguards class variable changes within function string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } int main() { cout << "The Movie List program\n\n"…arrow_forward
- #include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; class Movie { private: string title = ""; int year = 0; public: void set_title(string title_param); string get_title() const; // "const" safeguards class variable changes within function string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } int main() { cout << "The Movie List program\n\n"…arrow_forwardBasic javaarrow_forwardMake Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
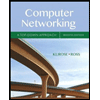
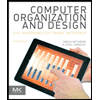
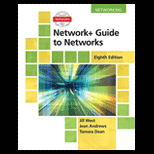
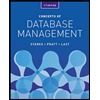
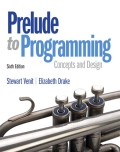
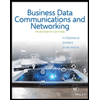