What is wrong with this code? # import random module import random #Main function def main(): # Initilize local variables randNumber=0 choice=1 while(choice>0): # Get a random number randNumber=random.randint(1,100)
What is wrong with this code?
# import random module
import random
#Main function
def main():
# Initilize local variables
randNumber=0
choice=1
while(choice>0):
# Get a random number
randNumber=random.randint(1,100)
# Call the function to guess the number
choice=GuessingGame(randNumber)
#Print the statement
print('Thanks for playing!')
# Function definition
def GuessingGame(num):
# Get a value from the user
guess=int(input('Enter a number between 1 and 100, or 0 to quit:'))
# Do until value is greater than 0
while guess>0:
if guess>num:
# Print the statement
print('Too high, try again')
# Get a value from the user
guess=int(input('Enter a number between 1 and 100,'\
'or 0 to quit:'))
elif guess<num:
# Print the statement
print('Too low, try again')
#Get a value from the user
guess=int(input('Enter a number between 1'\
'and 100, or 0 to quit:'))
else:
# Print the statement
print('Congratulations! You guessed the right number!')
# Return the value
return guess

Step by step
Solved in 2 steps with 3 images

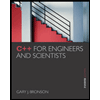
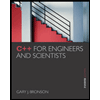