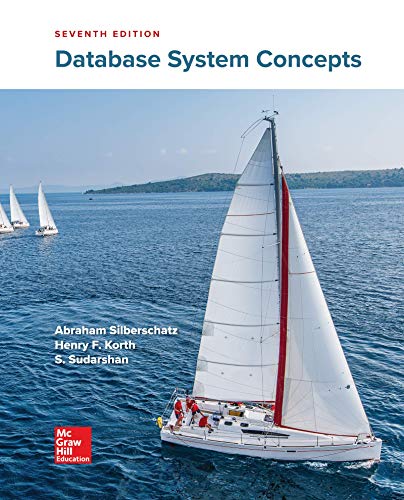
Write a code in Java programming
You are working in a company, and your new task is creating an encoding
All the even-frequency characters should be placed before the odd-frequency characters, and finally, all characters should be sorted in increasing order.
Your colleague is sick again, and he cannot finish his task. He gave you an unfinished code like the following:
public static String encode(String code) {
// MyFunction mf = // TODO
return mf.encode(code);
}
Your job is to complete the implementation of the functional interface MyFunction that encode() method should use to encode the given String code.
The MyFunction interface should have the following signature:
interface MyFunction {
String encode(String code);
}
Additionally, your algorithm should handle the following cases:
1. If the input String is null, the encode() method should return null.
2. If the input string is empty or contains only whitespaces, the encode() method should return an empty string.
3. If the input String contains non-alphanumeric characters, the encode() method should ignore them.
Thus, if you complete the above code correctly, you should have an output like the following:
encode("98089911223") returns "11228803999"
encode("333000111") returns "000111333"
encode("001119772") returns "007711129"
encode("") returns ""
encode(null) returns null
encode(" \t\n") returns ""
encode("aBcD-12!") returns "12Bac"
Note that you should not change the method signature of the MyFunction interface or the encode() method.

Step by stepSolved in 3 steps

- Write a java class method named findFourLetterWord that searches an array of words (String objects) for a wordthat’s four characters long. The array will be the method’s only parameters. findFourLetterWord will return thefirst four-letter word that it finds. If none of the words have the proper length, the method will return null.arrow_forwardComputer Science Consider a look-up service for the television shows on a given date. A file contains information about these shows. Each show’s data appears on two lines. The first line gives the name of the station, the channel, the name of the show, and a rating. These entries are separated by tildes (~). The second line briefly describes the show. Write pseudocode for a method with the header public void readFile(Scanner data) to read the file into a dictionary that will be searched. Decide what data should be the search key and what should be the associated value. Design any classes needed for the key and the value. Write pseudocode for the method Do not write any code for the answer. Use UML, pseudocode, and written Description.arrow_forwardWrite a java method that receives a reference to an array of integers and then sorts only the non-perfect numbers. if a number is perfect then it must remain in its current location in the array. Notes: 1) You are not allowed to use the Arrays.sort() method or any other ready-made sorting method. 2) You can not define new arrays. Your sorting must be in-place. 3) A number if perfect if the some of its divisors is equal to the number itself. For example the number 6 is perfect because the sum of its divisors is 1+2+3 = 6 while 8 is not perfect because the sum of its divisors is 1+2+4 = 7 which is not equal to 8.arrow_forward
- Write a Java program tat prompts user for a list of integers with 0 as the last value.Save the values in an array. Assume there can be maximum 100 values. Theprogram should have the following methods:- A method that takes the array as parameter and updates each value in thearray to the square of the value.- Another method that takes the original and modified arrays as parametersand displays the original and the squared values.arrow_forwardWrite a program called Guests.java that prompts a user to enter how many guests he will host. Next, reads a series of the names into an array of Strings using Scanner, and then prints back "Welcome" to all of those guests.arrow_forwardWrite in javaarrow_forward
- Write the following programs in Java: In the Converter class write the static method, bin2decimal(String bin). The parameter for this method is an unsigned (nonnegative) binary number represented by a string. The method returns the decimal equivalent for the binary parameter, as an integer.If the binary string is invalid (contains characters other than ‘0’ and ‘1’), the method returns -1. Convert from a binary (b) string with up to 16 digits to decimal (d) using the following formula:? = ?0 + ?1 × 2 + ?2 × 22 +⋯+ ?7 × 215where b0, b1, ?2, … ?7 are the binary digits in order of increasing significance. For example, 1101 is: 1 + 0×2 + 1×22 + 1×23 = 13 in decimal. Side note: You may NOT use the decode method of the Long wrapper class instead of this formula. In the Converter class write another static method, english2encrypted(String english). The parameter for this method is a word or sentence represented by a string. The word or sentence should only contain letters (no digits,…arrow_forwardI need to write a Java ArrayList program called Search.java that counts the names of people that were interviewed from a standard input stream. The program reads people's names from the standard input stream and from time to time prints a sorted portion of the list of names. All the names in the input are given in the order in which they were found and interviewed. You can imagine a unique timestamp associated with each interview. The names are not necessarily unique (many of the people have the same name). Interspersed in the standard input stream are queries that begin with the question mark character. Queries ask for an alphabetical list of names to be printed to the standard output stream. The list depends only on the names up to that point in the input stream and does include any people's names that appear in the input stream after the query. The list does not contain all the names of the previous interviews, but only a selection of them. A query provides the names of two people,…arrow_forwardWrite a Java program that will allow the user to play a hangman game repeatedly until theychoose to stop. The game should randomly choose a secret word from a pre-set list of wordsfor the user to guess. Store the list of words available for play in an array so you can randomlychoose them by index during game play.During a turn, the user will guess one letter at a time. Each letter in the secret word shoulddisplay as an asterisk until the letter is guessed by the user. When a correct letter is guessed,the letter should be displayed in the correct position in place of the asterisk. The user hangshimself with the 8th wrong guess and loses the game.Make a character array to hold the characters and asterisks representing the word beingguessed. You can change the contents of this array as the letters are guessed and revealed. Inother words, this array can be used to display the current state of the secret word each round.Here is the list of words the game should choose from for the secret…arrow_forward
- This question is in java The Sorts.java file is the sorting program we looked at . You can use either method listed in the example when coding. You want to add to the grades.java and calculations.java files so you put the list in numerical order. Write methods to find the median and the range. Have a toString method that prints the ordered list, the number of items in the list, the mean, median and range. Ex List: 20 , 30 , 40 , 75 , 93Number of elements: 5Mean: 51.60Median: 40Range: 73 Grades.java is the main file import java.util.Scanner;public class grades { public static void main(String[] args) { int n; calculation c = new calculation(); int array[] = new int[20]; System.out.print("Enter number of grades that are to be entered: "); Scanner scan = new Scanner(System.in); n = scan.nextInt(); System.out.println("Enter the grades: "); for(int i=0; i<n; i++) { array[i] = scan.nextInt(); if(array[i] < 0) {…arrow_forwardComputer Programming Java Write a method named FirstHalf that receives a String parameter named word and then returns the first half of the string. You are guaranteed as a precondition that word has an even length greater than 1.arrow_forwardjava a. A method named contains exists that takes an array of Strings and a single String, in that order, as arguments and returns a boolean value that is true if the array contains the given String. Assume that an array of Strings named names is already declared and initialized. Call the method to see if the array contains the name "Fred", and save the result in a new variable named fredIsHere. b. Given a method named printMessage that takes a String argument and returns nothing, call the method to print out "I love Java!".arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
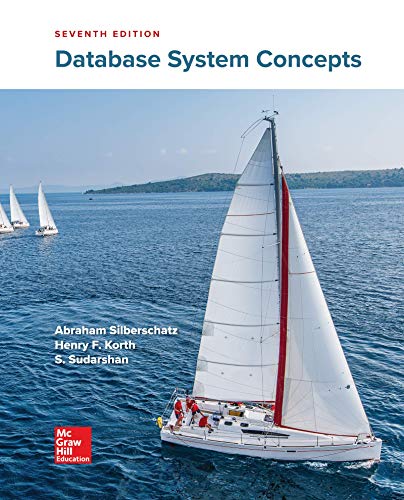
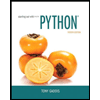
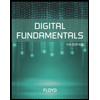
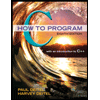
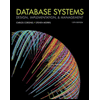
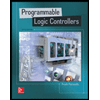